Sum of Digits of a Number
Given a number num, find the sum of digits of the number.
What is the "Sum Of Digits of A Number"
Input: 687
Output: 21
Explanation: The sum of digits i.e. 6 + 8 + 7 is equal to 21.
Input: 12
Output: 3
Explanation: The Sum Of Digits of A Number i.e. 1 + 2 is equal to 3.
So, if you are given an integer num and you are told to find the sum of the digits of that number.
The simple approach would be to extract the digit of that number and keep adding it to the sum.
But the question is how to extract the digit.
Approach
The idea is to add the digits starting from the rightmost (least significant) digit and moving towards the leftmost (most significant) digit. This can be done by repeatedly extracting the last digit from the number using modulo 10 operation, adding it to the sum, and then removing it using integer division by 10.
Let’s understand modulo in brief
The modulo (%) operator helps us to extract the last digit of a number by giving the remainder when divided by 10.
Example:
Let's take the number 15:
- If we perform 15 % 10, we get 5.
- Why? Because 15 divided by 10 gives a quotient of 1 and a remainder of 5.
- Mathematically, 15÷10=1 (quotient),15%10=5 (remainder)
NOTE: Using number % 10, we always get the rightmost digit of the number.
Why not extract digits from Front?
Extracting digits from the front of a number is complex because it requires first determining the number of digits, then repeatedly subtracting and dividing by powers of 10. For example, extracting the leftmost digit of 9742 requires:
9742÷1000=9,(9742−9×1000)÷100=7,and so on.
This involves multiple operations for each digit.
In contrast, extracting from the back is simpler using modulo (%) and integer division (//). We repeatedly take number % 10 to get the last digit and remove it using number // 10. This avoids extra calculations, making it more efficient and straightforward.
Let's take an example for number 15, if we want to find the digit in the one's place of this number what should we do here?
We know that the given number is in base 10, so somehow, if we get the remainder of the number when divided by 10, we will get the digit in the one place of that number.
Now, there is an operator that makes our work easy here, i.e., the modulo(%) operator. Now there is an operator that makes our work easy here i.e. modulo(%) operator.
As discussed above, modulo (%) Operator is used to find the remainder.
Let's take a question: when A = 15 is divided by B = 10, what is the remainder?
We can simply find it using A%B i.e. it will give us the remainder when 15 is divided by 10.
So the remainder is 5.
In general, In the decimal number system, to get the :
Last digit: we modulo it with 10^1 = 10 (remainder lies from 0 to 9).
Last two digits, we modulo it with 10^2 = 100 (the remainder lies from 0 to 99).
For the last n digit, we modulo it with 10^n = 1000…. n times (remainder lies from 0 to 10^n - 1).
But here in this question, we only need a single digit at a time and add that digit to our answer to get the final result. But the question is, after adding one digit to our answer, what should we do to add the tens place digit?
To add the tens place digit to our answer, we have to first divide the current number by 10, which shifts the tens place to the one’s place. Then, we extract that particular digit using the modulo (%) operator. This process will continue until our number becomes 0.
Let's understand it with an example for num = 12345
Initially, num is 12345, and sum is 0. The number is in the decimal system (Base 10).
- First iteration:
- Take the last digit using modulo: 12345 % 10 = 5.
- Add 5 to the sum.
- Remove the last digit by dividing: 12345 / 10 = 1234.
- num becomes 1234.
- sum = 0 + 5.
- Second iteration:
- Take the last digit using modulo: 1234 % 10 = 4.
- Add 4 to the sum.
- Remove the last digit by dividing: 1234 / 10 = 123.
- num becomes 123.
- sum = 0 + 5 + 4.
- Third iteration:
- Take the last digit using modulo: 123 % 10 = 3.
- Add 3 to the sum.
- Remove the last digit by dividing: 123 / 10 = 12.
- num becomes 12.
- sum = 0 + 5 + 4 + 3.
- Fourth iteration:
- Take the last digit using modulo: 12 % 10 = 2.
- Add 2 to the sum.
- Remove the last digit by dividing: 12 / 10 = 1.
- num becomes 1.
- sum = 0 + 5 + 4 + 3 + 2.
- Fifth iteration:
- Take the last digit using modulo: 1 % 10 = 1.
- Add 1 to the sum.
- Remove the last digit by dividing: 1 / 10 = 0.
- num becomes 0.
- sum = 0 + 5 + 4 + 3 + 2 + 1.
Now, num has become 0, so dividing it further would always give a remainder of 0.
Since there are no more digits left to process, the loop terminates. Exit.
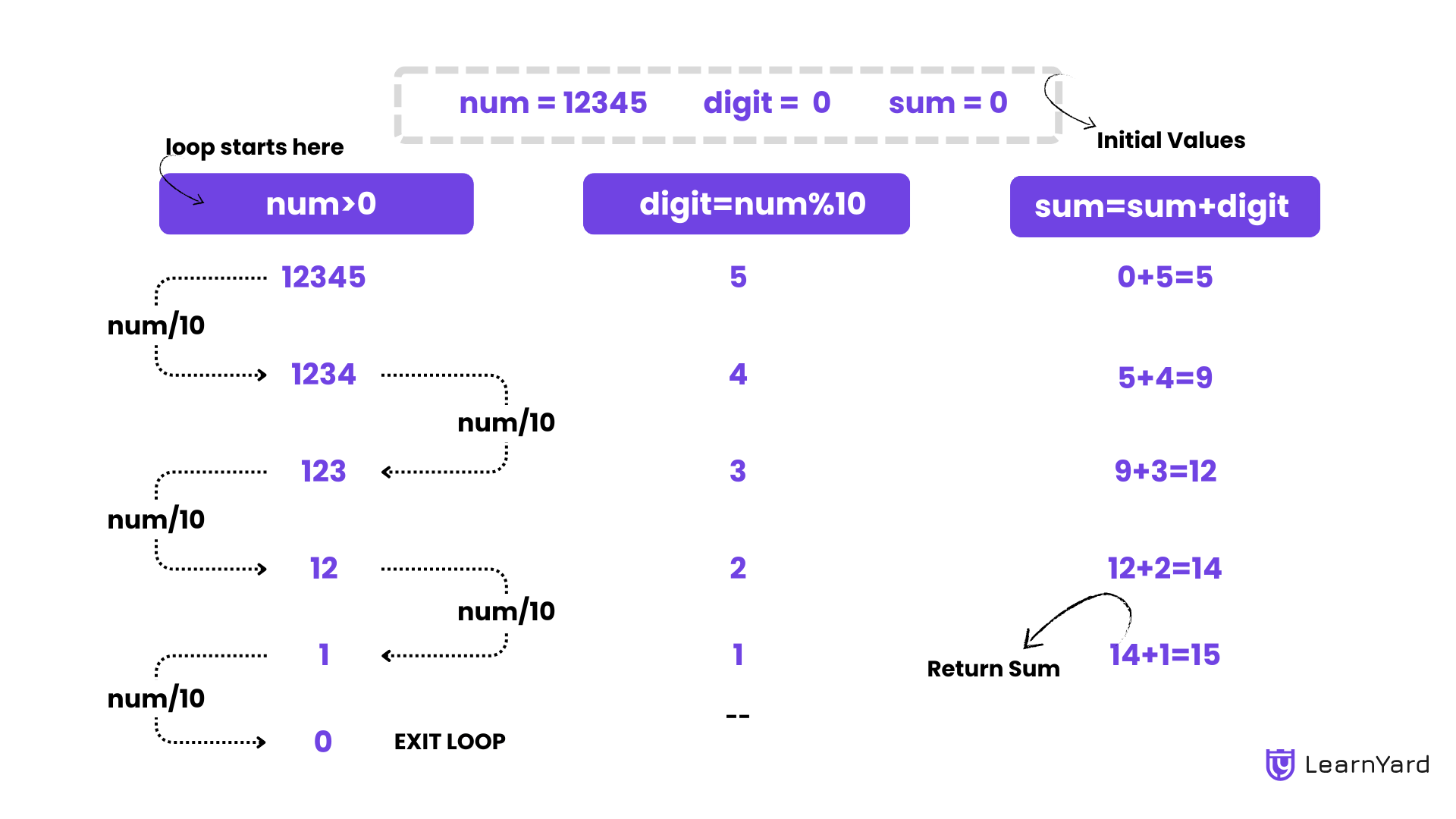
Code Implementation
C++ Code Try on Compiler!
int sumOfDigits(int num) {
int sum = 0;
while (num > 0) {
// Extract the last digit
int digit = num % 10;
// Add the digit to the sum
sum = sum + digit;
// Remove the last digit
num =num / 10;
}
return sum;
}
Java Code Try on Compiler!
class Solution {
public static int sumOfDigits(int num) {
int sum = 0;
while (num > 0) {
// Extract the last digit
int digit = num % 10;
// Add the digit to the sum
sum += digit;
// Remove the last digit
num /= 10;
}
return sum;
}
}
Python Code Try on Compiler!
def sum_of_digits(num):
sum = 0
while num > 0:
# Extract the last digit
digit = num % 10
# Add the digit to the sum
sum += digit
# Remove the last digit
num //= 10
return sum
Javascript Code Try on Compiler!
function sumOfDigits(num) {
let sum = 0;
while (num > 0) {
// Extract the last digit
let digit = num % 10;
// Add the digit to the sum
sum += digit;
// Remove the last digit
num = Math.floor(num / 10);
}
return sum;
}
Time Complexity : O(logn)
The time complexity arises from the number of digits in the number. The number of times the while loop runs depends on the number of digits in the given number num.
For a given number num in a decimal representation, the number of digits is approximately equal to log10(n). This is because each digit represents a power of 10.
The loop executes once for each digit in num.
Therefore, the loop runs log10(num) times, simplifying to O(logn).
Space Complexity : O(1)
We are using only the sum variable, which uses constant space.
Since the space required by these variables does not depend on the size of the input number num, the space complexity is O(1).
Now we have learned how to extract digits from a number and we have solved the Sum Of Digits of A Number.