Special Array with X Elements Greater Than or Equal X Solution In C++/Java/Python/JS
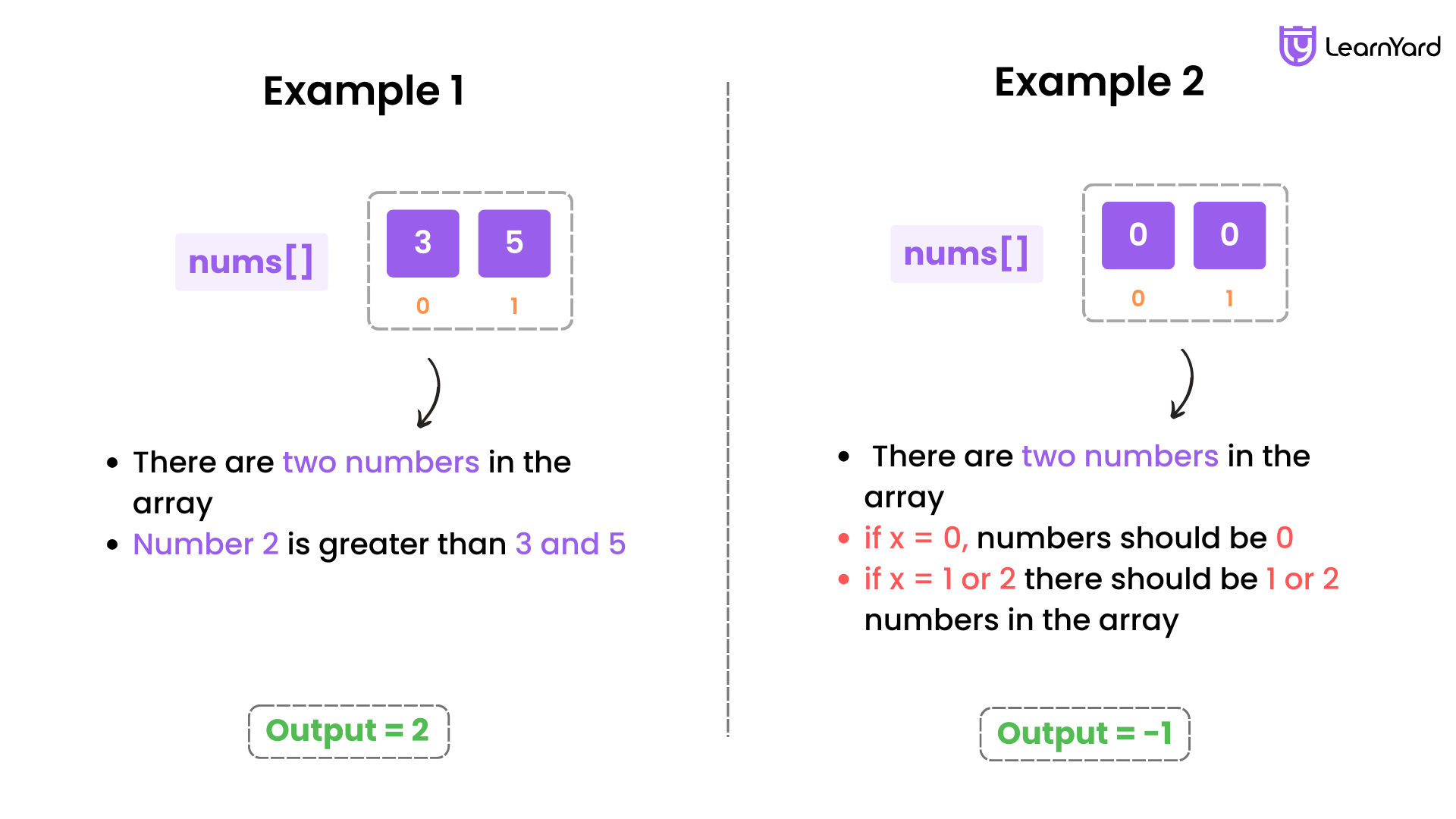
Problem Description:
You are given an array nums of non-negative integers. nums is considered special if there exists a number x such that there are exactly x numbers in nums that are greater than or equal to x.
Notice that x does not have to be an element in nums.
Return x if the array is special, otherwise, return -1. It can be proven that if nums is special, the value for x is unique.
Examples:
Input: nums = [3,5]
Output: 2
Explanation: There are 2 values (3 and 5) that are greater than or equal to 2.
Input: nums = [0,0]
Output: -1
Explanation: No numbers fit the criteria for x.
If x = 0, there should be 0 numbers >= x, but there are 2.
If x = 1, there should be 1 number >= x, but there are 0.
If x = 2, there should be 2 numbers >= x, but there are 0.
x cannot be greater since there are only 2 numbers in nums.
Input: nums = [0,4,3,0,4]
Output: 3
Explanation: There are 3 values that are greater than or equal to 3.
Constraints:
- 1 <= nums.length <= 100
- 0 <= nums[i] <= 1000
Special Array With X Elements Greater Than or Equal X Problem
Given an array nums. We need to find a special array with X elements greater than or equal to X. That means we need to find some value x such that there are exactly x elements in nums with values greater than or equal to x.
Now that we understand the problem, ask yourself what steps to follow to the given array as a special array.
Brute Force Approach
Intuition:
Think about the problem by focusing on what we need to find—a single number x. For the given array to be a special array, there must be some x such that the array contains x elements such that their value is greater than or equal to x. Our goal is to find this x.
Special Array With X Elements Greater Than or Equal X Solution
To understand the possible values of x, we should note that since the list has n numbers, x can only be between 1 and n. It’s impossible to have more than n numbers that are at least n because the array itself only contains n numbers. So, we only need to check the values of x in this range.
To determine whether a particular value of x is valid, we check how many elements in the array are greater than or equal to x. If this count matches x, then x is a valid answer. As we test increasing values of x, we can observe a pattern. If x is too small, there are usually more than x elements that meet the condition, making x a possible candidate. However, as x increases, the number of elements that satisfy the condition decreases.
Special Array With X Elements Greater Than or Equal X example
nums = [2, 3, 1, 4, 5]
- Let x = 1, then we see the number of elements greater than equal to 1 are [2, 3, 1, 4, 5], that is 5 elements.
- Let x = 2, then we see the number of elements greater than equal to 2 are [2, 3, 4, 5], that is 4 elements.
- Let x = 3, then we see the number of elements greater than equal to 3 are [3, 4, 6], that is 3 elements.
- Let x = 4, then we see the number of elements greater than equal to 4 are [4, 5], that is 2 elements.
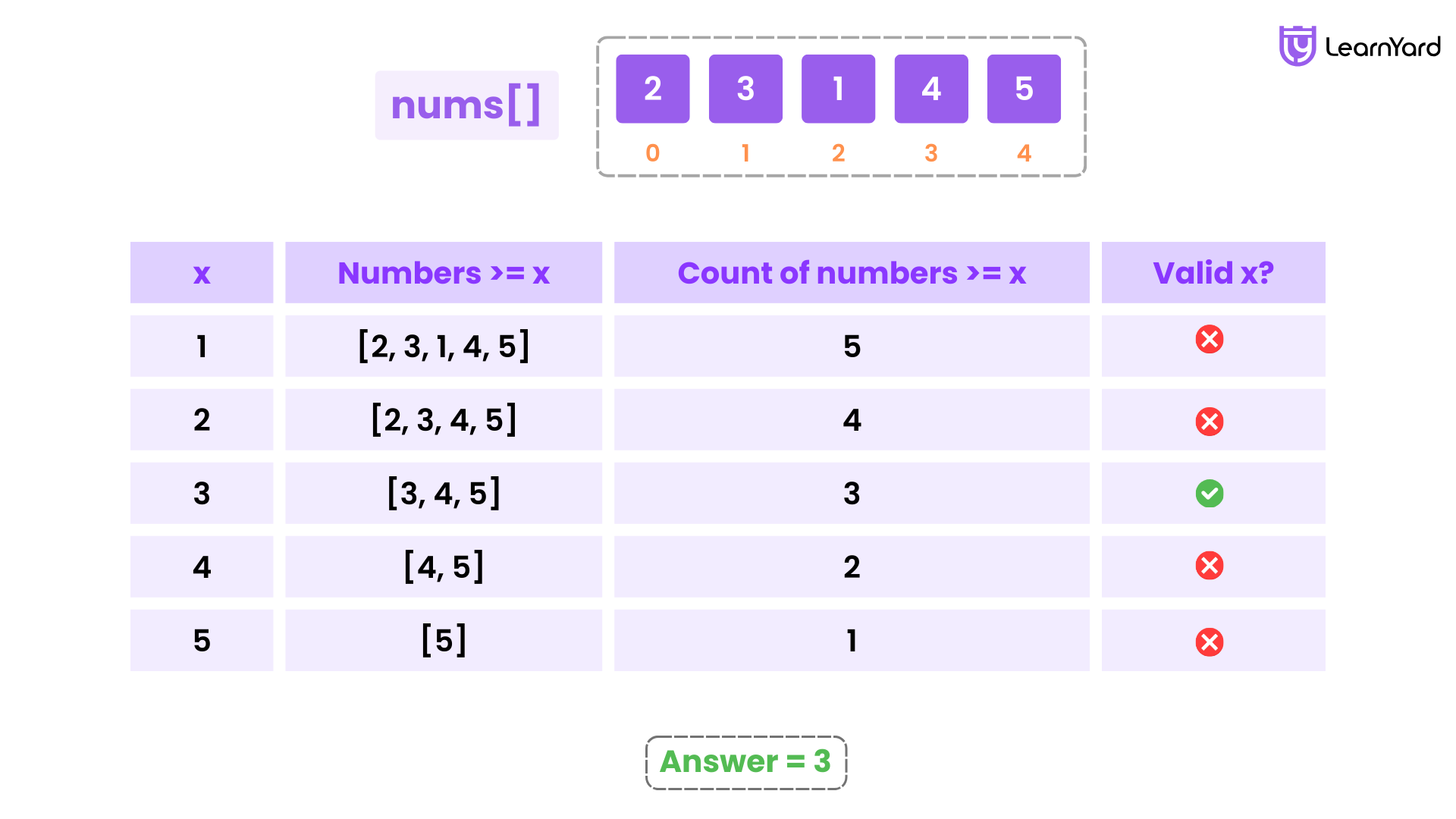
Initially, for small values of x, enough elements are satisfying the requirement. But as we increase x, there comes a point where the count of elements meeting the condition is no longer sufficient. Beyond this point, for larger values of x, the condition continues to fail. This means that if a valid x exists, it is the highest value where the condition holds before it starts failing for all larger values.
Special Array With X Elements Greater Than or Equal X Solution
For each possible x, we count how many numbers in the list are greater than or equal to x. If this count exactly matches x, then we have found our answer. Otherwise, we continue checking the next value. If no such x is found by the time we check all possibilities, we return -1.
This method ensures we systematically check all valid values of x and return the correct answer efficiently. Easy right?
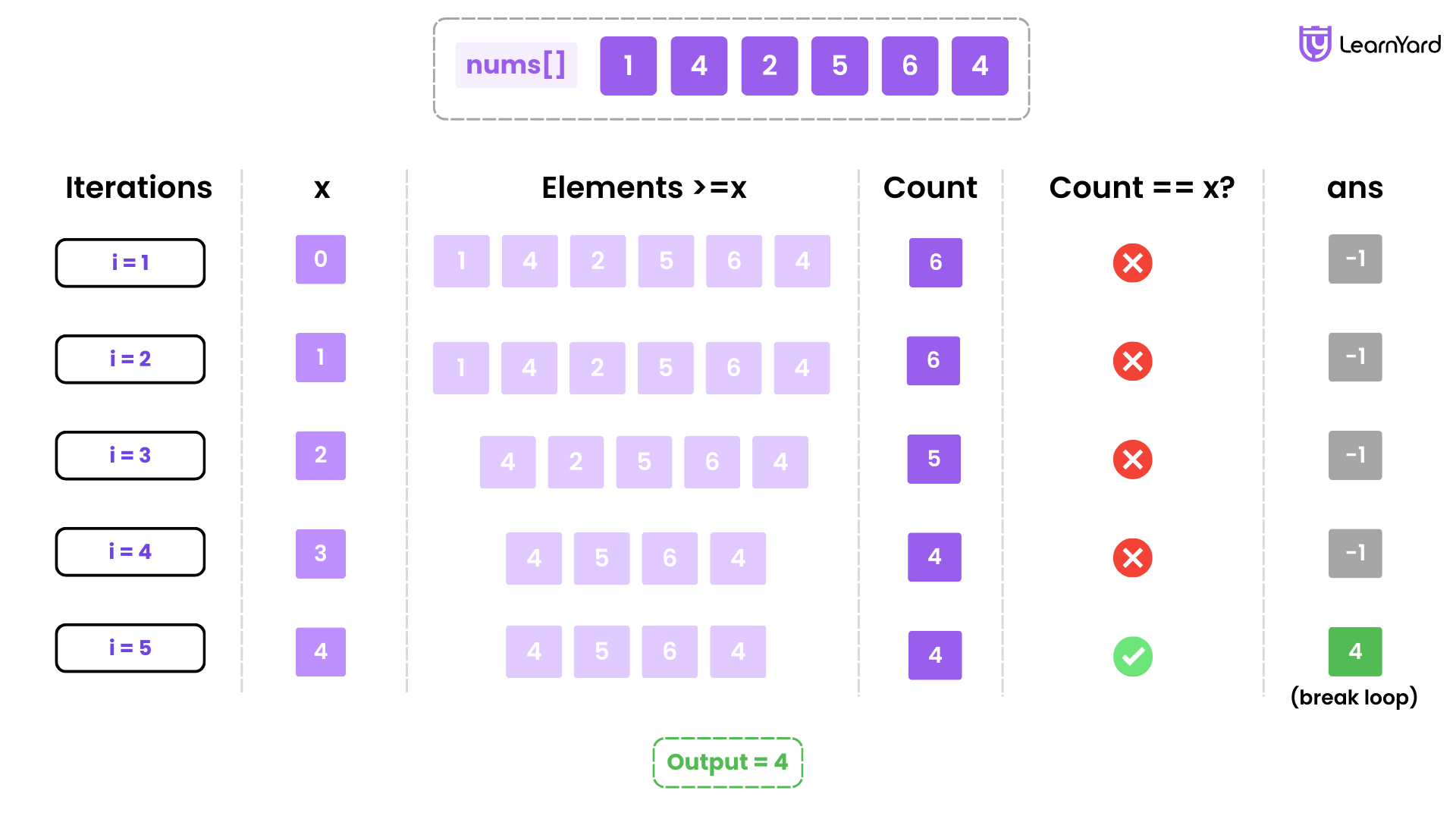
Dry run
Input: nums = [1, 4, 2, 5, 6, 4]
Output: 3
Explanation: There are 4 values [4, 5, 6, 4] which are greater then 4 in the array. Hence we return 4.
Initialization
- n=6 (size of nums)
- ans = -1 (default answer)
Loop over possible values of x (from 0 to n)
Iteration 1: x = 0
- Count elements ≥ 0: 6 (all elements)
- Since cnt != x (6 ≠ 0), continue.
Iteration 2: x = 1
- Count elements ≥ 1: 6 (all elements)
- Since cnt != x (6 ≠ 1), continue.
Iteration 3: x = 2
- Count elements ≥ 2: 5 ([4, 2, 5, 6, 4])
- Since cnt != x (5 ≠ 2), continue.
Iteration 4: x = 3
- Count elements ≥ 3: 4 ([4, 5, 6, 4])
- Since cnt != x (4 == 3), continue
Iteration 5: x = 4
- Count elements ≥ 4: 4 ([4, 5, 6, 4])
- Since cnt == x (4 == 4), set ans = 4 and break the loop.
Final Output
- Since we found x = 4 which satisfies the condition, the function returns 4.
Steps to write code
Step 1: Initialization
- Read the input array nums.
- Get the size of nums, denoted as n.
- Initialize ans = -1 (default return value).
Step 2: Iterate Over Possible Values of x
- Loop x from 0 to n (since x must be a valid count of elements).
- For each x, count how many elements in nums are greater than or equal to x.
Step 3: Count Elements ≥ x
- Initialize a counter cnt = 0.
- Iterate through nums:
- If an element is ≥ x, increment cnt.
Step 4: Check Condition
- If cnt == x, update ans = x and break the loop.
Step 5: Return Result
- Return the value stored in ans.
Special Array With X Elements Greater Than or Equal X solution
Special Array With X Elements Greater Than or Equal X solution in C++
class Solution {
public:
// Function to find the special array value
int specialArray(vector<int>& nums) {
// Get the size of the array
int n = nums.size();
// Iterate from 0 to n
for (int x = 0; x <= n; x++) {
// Initialize counter to count elements >= x
int cnt = 0;
// Loop through all elements in nums
for (int num : nums) {
// Check if element is greater than or equal to x
if (num >= x) {
// Increment count
cnt++;
}
}
// If count matches x, return x
if (cnt == x) {
return x;
}
}
// If no such x is found, return -1
return -1;
}
};
Special Array With X Elements Greater Than or Equal X solution in java
class Solution {
// Function to find the special array value
public int specialArray(int[] nums) {
// Get the size of the array
int n = nums.length;
// Iterate from 0 to n
for (int x = 0; x <= n; x++) {
// Initialize counter to count elements >= x
int cnt = 0;
// Loop through all elements in nums
for (int num : nums) {
// Check if element is greater than or equal to x
if (num >= x) {
// Increment count
cnt++;
}
}
// If count matches x, return x
if (cnt == x) {
return x;
}
}
// If no such x is found, return -1
return -1;
}
}
Special Array With X Elements Greater Than or Equal X solution in python
class Solution:
# Function to find the special array value
def specialArray(self, nums: List[int]) -> int:
# Get the size of the array
n = len(nums)
# Iterate from 0 to n
for x in range(n + 1):
# Count elements that are greater than or equal to x
cnt = sum(1 for num in nums if num >= x)
# If count matches x, return x
if cnt == x:
return x
# If no such x is found, return -1
return -1
Special Array With X Elements Greater Than or Equal X solution in javascript
/**
* Function to find the special array value
* @param {number[]} nums - The input array
* @return {number}
*/
var specialArray = function(nums) {
// Get the size of the array
let n = nums.length;
// Iterate from 0 to n
for (let x = 0; x <= n; x++) {
// Count elements that are greater than or equal to x
let cnt = nums.filter(num => num >= x).length;
// If count matches x, return x
if (cnt === x) {
return x;
}
}
// If no such x is found, return -1
return -1;
};
Complexity Analysis of the Solution
Time Complexity: O(n2)
The function iterates through values of x from 0 to n, and for each x, it counts elements in nums that are >= x.
Breaking it Down:
- Outer loop: Runs from 0 to n, so it iterates O(n) times.
- Inner loop (counting elements ≥ x): For each x, we iterate over nums, which takes O(n) time.
Since the inner loop runs for each iteration of the outer loop, the total worst-case complexity is:
O(n)×O(n)=O(n2)
Space Complexity: O(1)
Auxiliary Space Complexity
Extra space used beyond input storage (temporary variables, additional data structures, etc.).
- The function only uses a few integer variables (n, x, cnt), which take O(1) space.
- No extra data structures are created (like arrays, hash maps, etc.).
- In Python and JavaScript, functions like .sum() and .filter() iterate over the input but do not allocate extra space.
Thus, auxiliary space complexity = O(1) (constant space).
Total Space Complexity
It includes both Input space + auxiliary space.
- Input storage (nums[] array): O(n) space is required to store n integers.
- Auxiliary space: O(1).
Hence, the Total Space Complexity is O(n)+O(1)=O(n)
Is the brute force approach efficient?
The given constraint on n is up to 100.
The brute-force approach we designed to check if an array is a special array has a time complexity of O(n²). This means that in the worst case, the solution will perform at most: 100×100=10,000
Since modern systems can handle 106 operations per second, our approach, which takes only 104 operations, is efficient and expected.
However, let's see if we can optimize it further to improve our Special Array With X Elements Greater Than or Equal X leetcode solution.
Optimal Approach
Intuition:
Our current solution has a time complexity of O(n²), mainly due to the two nested loops used to iterate over n.
To optimize our approach, let's first understand why we need both loops and how they contribute to the solution. This will help us find a way to optimize our solution.
Understanding the purpose of both loops
- The first loop iterates over every possible value of x to check if this x is the one that can make nums a special array.
- For each x, the second loop counts how many elements in nums are greater than or equal to x. This is according to the condition of the special array mentioned in the problem statement.
Now, if we look closely, we see that for every fixed x, we must go through the entire array to count the elements that meet the condition. Since this step is necessary, we should focus on optimizing the first loop to improve efficiency.
Let's explore what happens if we choose x as a valid value, such as the middle (mid) of the possible range of x which is from 0 to n inclusive.
Now after counting the values in the array that are greater than or equal to x, suppose we found a few elements that are greater than or equal to x using a simple loop. Now we know that if the count is equal to x we got our answer. But let's see the cases where count is not equal to x.
Case 1: Count of elements greater than or equal to x is less than x
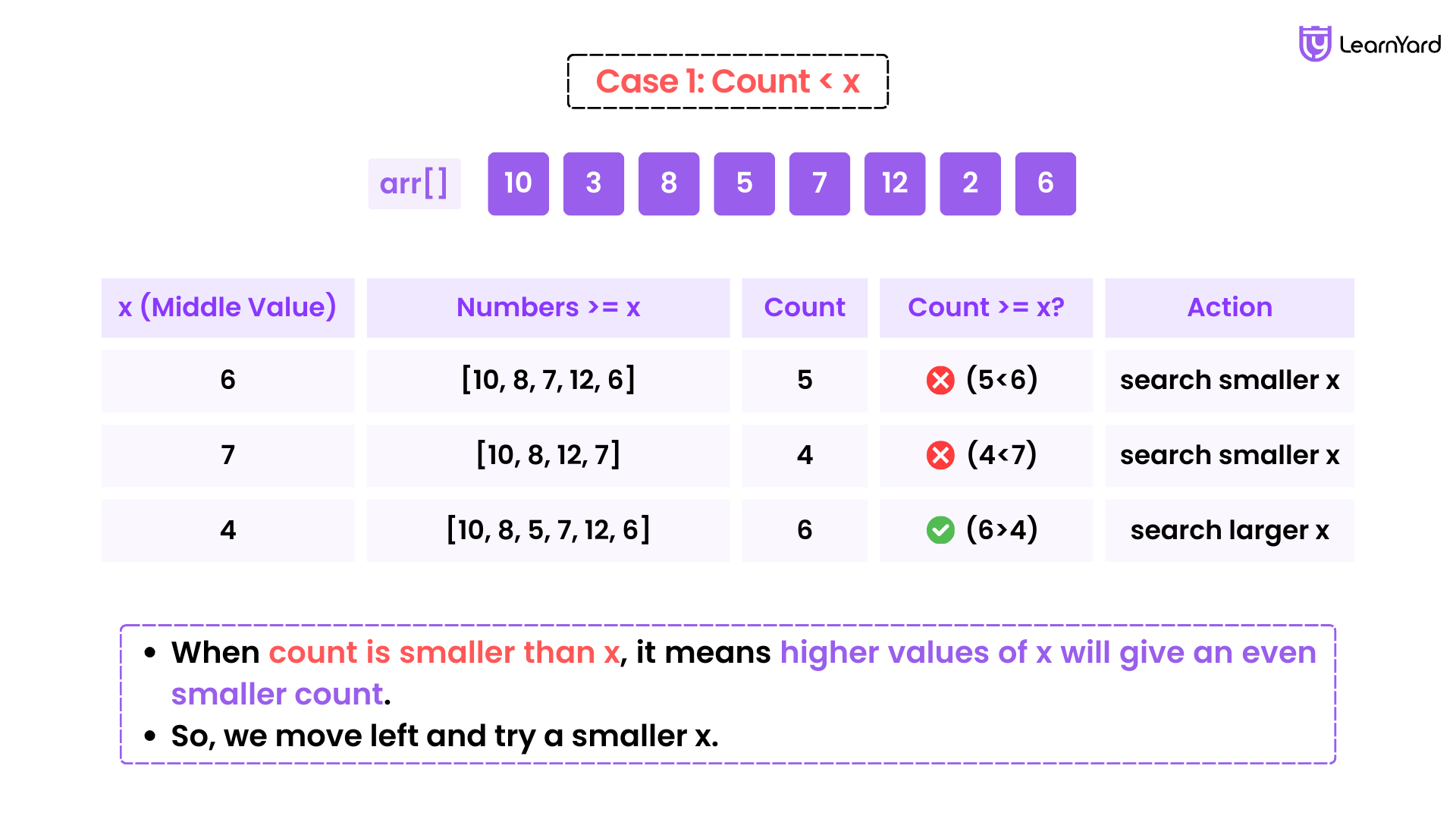
If we find that the count of elements greater than or equal to a specific value x is less than x, then we know that any value greater than x will also give a smaller count. This happens because as x increases, fewer elements will be greater than or equal to x. So, once we find an x where the count is less than x, there's no need to check values greater than x.
It's because counting for the values that are greater than x will only give an even smaller count. Due to the monotonic nature of the search space.
With this knowledge, we can safely rule out all values larger than x as potential answers.
If the count is less than x, we then look at the values to the left of x, in the range from 0 to x-1, because the answer might be in this range.
Case 2: Count of elements greater than or equal to x is greater than x
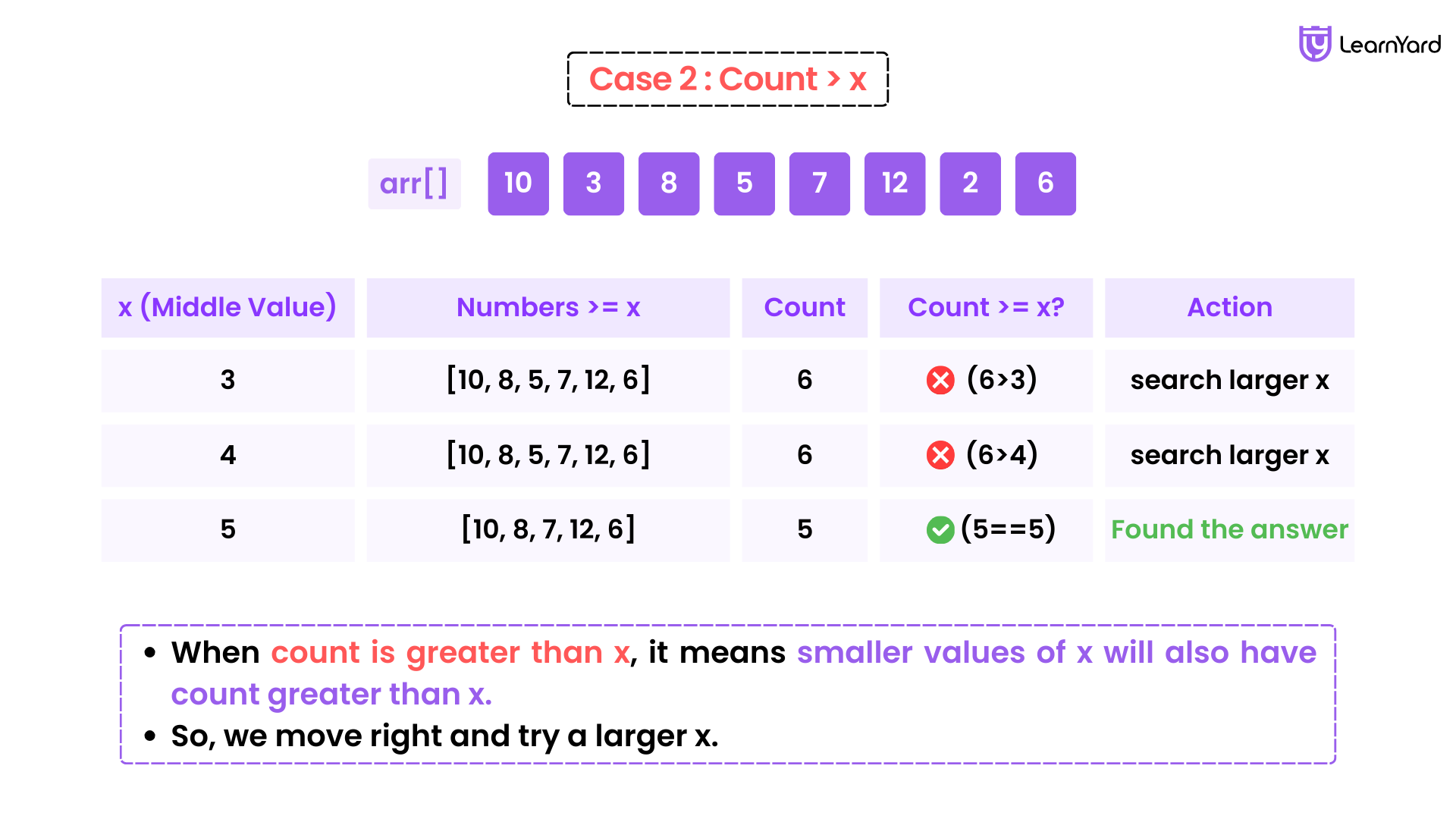
If we find that the count of elements greater than or equal to a specific value x is greater than x, this means that more elements are satisfying the condition than needed. Since x is already providing a count larger than x, it indicates that any value smaller than x will also give a larger count.
It's because the values that are greater than equal to this x are also greater for the x in the range 0 to x-1. Due to monotonic nature of the search space.
So, once we find an x where the count is greater than x, we know that values smaller than x will never give us the exact count of x. Therefore, we can safely rule out all values smaller than x as potential solutions.
With this information, we then focus on the values to the right of x, in the range from x+1 to n, because the answer could be in this range, where the count might match exactly x.
Example
Let’s consider the array:
nums = [10, 3, 8, 5, 7, 12, 2, 6]
We need to find an x such that the number of elements greater than or equal to x equals x.
Consider x = 6
First, We need to count how many elements in the array are greater than or equal to 6. The elements in the array that meet this condition are [10, 8, 7, 12, 6]. So, the count is 5.
Now, compare this count (5) with x (6). Since 5 is less than 6, we know that x = 6 cannot be the answer. The count is too small.
And because the count for x = 6 is already smaller than 6, any larger value of x will result in an even smaller count. This is because as x increases, fewer elements will be greater than or equal to x.
To understand this better, consider x = 7
Now, let’s check x = 7. We count how many elements are greater than or equal to 7. The elements in the array that meet this condition are [10, 8, 12, 7]. So, the count is 4.
Now, compare this count (4) with x (7). Since 4 is less than 7, we know that x = 7 also cannot be the answer.
At this point, we realize that any value greater than 7 (such as x = 8, x = 9, etc.) will only give a smaller count. This happens because, as x increases, even fewer elements will satisfy the condition of being greater than or equal to x.
Now, consider x = 4
First, We need to count how many elements in the array are greater than or equal to 4. The elements that meet this condition are: [10, 8, 5, 7, 12, 6]. So, the count is 6.
Now, compare this count (6) with x (4). Since 6 is greater than 4, it means that any value less than 4 will also have a count greater than or equal to 6.
To understand this better, Consider x = 3
Now, let’s check for x = 3. The elements that are greater than or equal to 3 are: [10, 8, 5, 7, 12, 6]. So, the count is 6.
Since the count is still 6, and the count is larger than x (3), we can infer that for x = [0, 1, 2] also count will be either 6 or greater than 6, hence we can now safely conclude that values smaller than 3 can't be a valid x.
At this point. You might be thinking that you have studied a familiar algorithm that works exactly like we have discussed... Yes, it is none other than binary search. To know more about it refer to the article below.
Special Array With X Elements Greater Than or Equal X Algorithm
To begin with, we define the search space. This space is represented by two pointers, low and high. Initially, low is set to 0, which is the smallest possible value that x can take. On the other hand, high is set to n, where n is typically the total number of elements in the array. The largest value x can take.
Once the search space is defined, we proceed by choosing the middle element. The middle element, denoted as mid, serves as a candidate for x. The idea behind selecting the middle value is to reduce the search space efficiently. By checking the middle value, we can determine if the answer lies to the left or right of the middle.
After selecting the middle value mid, the next step is to check if this value of x makes the array "special." To do this, we count how many elements in the array are greater than or equal to the chosen mid.
If the count of elements is equal to mid
- We got our answer. Hence, we return mid.
If the count of elements is less than mid
- We eliminate the right half of the search space and look from 0 to x-1
If the count of elements is greater than mid
- We eliminate the left half of the search space and look from x+1 to n.
By using this binary search approach, we efficiently explore potential values of x without having to check each one individually, which is much more efficient than a brute-force approach.
Let us understand this with a video,
Special Array With X Elements Greater Than or Equal X-Optimal Approach Animation
Dry run
Input: nums = [1, 4, 2, 5, 6, 4]
Output: 3
Explanation: There are 4 values [4, 5, 6, 4] which are greater then 4 in the array. Hence we return 4.
Initial Setup:
- The array nums contains 6 elements, so n = 6.
- The search space for the potential values of x is defined by low = 0 and high = n = 6.
Step 1: First Iteration
Initial Values:
- low = 0
- high = 6
Calculate mid:
- mid = (low + high) / 2 = (0 + 6) / 2 = 3
Count Elements Greater Than or Equal to mid = 3:
- We count the number of elements in nums that are greater than or equal to 3.
- Elements greater than or equal to 3 in nums = [1, 4, 2, 5, 6, 4] are: [4, 5, 6, 4].
- So, cnt = 4.
Compare cnt with mid:
- cnt = 4, mid = 3.
- Since cnt > mid, we adjust the search space:
- low = mid + 1 = 3 + 1 = 4.
Step 2: Second Iteration
Updated Values:
- low = 4
- high = 6
Calculate mid:
- mid = (low + high) / 2 = (4 + 6) / 2 = 5
Count Elements Greater Than or Equal to mid = 5:
- We count the number of elements in nums that are greater than or equal to 5.
- Elements greater than or equal to 5 in nums = [1, 4, 2, 5, 6, 4] are: [5, 6].
- So, cnt = 2.
Compare cnt with mid:
- cnt = 2, mid = 5.
- Since cnt < mid, we adjust the search space:
- high = mid - 1 = 5 - 1 = 4.
Step 3: Third Iteration
Updated Values:
- low = 4
- high = 4
Calculate mid:
- mid = (low + high) / 2 = (4 + 4) / 2 = 4
Count Elements Greater Than or Equal to mid = 4:
- We count the number of elements in nums that are greater than or equal to 4.
- Elements greater than or equal to 4 in nums = [1, 4, 2, 5, 6, 4] are: [4, 5, 6, 4].
- So, cnt = 4.
Compare cnt with mid:
- cnt = 4, mid = 4.
- Since cnt == mid, we have found our answer.
Final Output:
- The function returns 4, which is the correct answer.
Steps to write code
Step 1: Initialize Variables
- low: This will start at 0 (the smallest possible value for x).
- high: This will start at the size of the array n (the largest possible value for x).
- nums: This is the input array.
Step 2: Binary Search Loop
- Use a binary search loop where you repeatedly check the middle value of the current search range (low to high).
- This loop continues until low <= high.
Step 3: Calculate the Midpoint (mid)
- In each iteration of the binary search, calculate mid as the average of low and high.
- mid = (low + high) / 2
- This mid represents a candidate value for x.
Step 4: Count Elements Greater Than or Equal to mid
- Traverse the array nums to count how many elements are greater than or equal to mid.
- Initialize a counter cnt to 0 and loop through each element in the array.
- For each element nums[i], if nums[i] >= mid, increment the counter cnt.
Step 5: Compare the Count (cnt) with mid
- If the number of elements greater than or equal to mid (cnt) is equal to mid, then you’ve found the answer, and you return mid.
- If cnt > mid, it means we should search for a larger value for x, so adjust the search boundaries:
- Set low = mid + 1.
- If cnt < mid, it means we should search for a smaller value for x, so adjust the search boundaries:
- Set high = mid - 1.
Step 6: Return Result
- If no such value of x is found after the binary search loop ends, return -1 indicating that no solution exists.
Special Array With X Elements Greater Than or Equal X leetcode solution
Special Array With X Elements Greater Than or Equal X solution in c++
class Solution {
public:
// Function to find the special array value
int specialArray(vector<int>& nums) {
// Step 1: Get the size of the array
int n = nums.size();
// Step 2: Set binary search boundaries
int low = 0, high = n;
// Step 3: Perform binary search
while (low <= high) {
// Step 4: Calculate the middle value
int mid = (low + high) / 2;
// Step 5: Initialize counter for elements >= mid
int count = 0;
// Step 6: Count elements >= mid
for (int num : nums) {
if (num >= mid) {
count++;
}
}
// Step 7: If count equals mid, return mid
if (count == mid) {
return mid;
}
// Step 8: If count > mid, search for larger values
else if (count > mid) {
low = mid + 1;
}
// Step 9: If count < mid, search for smaller values
else {
high = mid - 1;
}
}
// Step 10: Return -1 if no valid x is found
return -1;
}
};
Special Array With X Elements Greater Than or Equal X solution in java
class Solution {
// Function to find the special array value
public int specialArray(int[] nums) {
// Step 1: Get the size of the array
int n = nums.length;
// Step 2: Set binary search boundaries
int low = 0, high = n;
// Step 3: Perform binary search
while (low <= high) {
// Step 4: Calculate the middle value
int mid = (low + high) / 2;
// Step 5: Initialize counter for elements >= mid
int count = 0;
// Step 6: Count elements >= mid
for (int num : nums) {
if (num >= mid) {
count++;
}
}
// Step 7: If count equals mid, return mid
if (count == mid) {
return mid;
}
// Step 8: If count > mid, search for larger values
else if (count > mid) {
low = mid + 1;
}
// Step 9: If count < mid, search for smaller values
else {
high = mid - 1;
}
}
// Step 10: Return -1 if no valid x is found
return -1;
}
}
Special Array With X Elements Greater Than or Equal to X solution in python
class Solution:
# Function to find the special array value
def specialArray(self, nums: List[int]) -> int:
# Step 1: Get the size of the array
n = len(nums)
# Step 2: Set binary search boundaries
low, high = 0, n
# Step 3: Perform binary search
while low <= high:
# Step 4: Calculate the middle value
mid = (low + high) // 2
# Step 5: Initialize counter for elements >= mid
count = 0
# Step 6: Count elements >= mid
for num in nums:
if num >= mid:
count += 1
# Step 7: If count equals mid, return mid
if count == mid:
return mid
# Step 8: If count > mid, search for larger values
elif count > mid:
low = mid + 1
# Step 9: If count < mid, search for smaller values
else:
high = mid - 1
# Step 10: Return -1 if no valid x is found
return -1
Special Array With X Elements Greater Than or Equal X solution in javascript
/**
* Function to find the special array value
* @param {number[]} nums - The input array
* @return {number}
*/
var specialArray = function(nums) {
// Step 1: Get the size of the array
let n = nums.length;
// Step 2: Set binary search boundaries
let low = 0, high = n;
// Step 3: Perform binary search
while (low <= high) {
// Step 4: Calculate the middle value
let mid = Math.floor((low + high) / 2);
// Step 5: Initialize counter for elements >= mid
let count = 0;
// Step 6: Count elements >= mid
for (let num of nums) {
if (num >= mid) {
count++;
}
}
// Step 7: If count equals mid, return mid
if (count === mid) {
return mid;
}
// Step 8: If count > mid, search for larger values
else if (count > mid) {
low = mid + 1;
}
// Step 9: If count < mid, search for smaller values
else {
high = mid - 1;
}
}
// Step 10: Return -1 if no valid x is found
return -1;
};
Complexity Analysis of the Solution
Time Complexity: O(n logn)
Binary Search Loop:
- The loop runs with low and high boundaries, and each time we calculate mid. The loop will continue as long as low <= high.
- The number of iterations of the binary search is proportional to log(n) because the search space is halved with each iteration.
Breaking It Down
- At the beginning: The search space has n elements.
- After 1st iteration: The search space is reduced to n/2.
- After 2nd iteration: The search space is n/4.
- After k iterations: The search space becomes n / 2k.
The search ends when only one element remains, which happens when: n/2k = 1
Taking log base 2 on both sides: k=log2(n)
Thus, binary search runs in O(log n) time complexity
Counting Elements:
- For each iteration of the binary search loop, we iterate over the entire array to count how many elements are greater than or equal to
mid
. This takes O(n) time for each iteration.
Thus, the overall time complexity is the product of these two steps:
- O(n) for counting the elements in the array.
- O(log n) for the binary search iterations.
So, the total time complexity is O(n log n).
Space Complexity: O(1)
Auxiliary Space Complexity:
Auxiliary space complexity refers to the additional space (memory) required by the algorithm during its execution, excluding the input data.
- For this problem, the auxiliary space complexity considers the variables like low, high, mid, and count used for the binary search and element counting. Variables take constant space O(1).
- No extra data structure used: The algorithm does not allocate any additional data structures (like arrays or hashmaps) and only uses a few scalar variables.
Thus, auxiliary space complexity is O(1).
Total Space Complexity:
Total space complexity refers to the overall memory used by the algorithm, including both the input data and any extra space (auxiliary space) required for computation.
- For the input array nums, the total space complexity is O(n) where n is the size of the input array. This is because the input array nums takes up n space in memory.
- The space used by auxiliary variables like low, high, mid, and count is O(1), so they do not change the total space complexity.
Hence, total space complexity is O(n).
Similar Problems
Now we have successfully tackled this problem, let's try these similar problems:-
You are given an integer n indicating there are n speciality retail stores. There are m product types of varying amounts, which are given as a 0-indexed integer array quantities, where quantities[i] represents the number of products of the ith product type.
You need to distribute all products to the retail stores following these rules:
- A store can only be given at most one product type but can be given any amount of it.
- After distribution, each store will have been given some number of products (possibly `0). Let x represent the maximum number of products given to any store. You want x to be as small as possible, i.e., you want to minimize the maximum number of products that are given to any store.
Return the minimum possible x.
Given an integer array nums of 2n integers, group these integers into n pairs (a1, b1), (a2, b2), ..., (an, bn) such that the sum of min (ai, bi) for all i is maximized. Return the maximized sum.