Print Alternate Elements of an Array
In certain scenarios, it's necessary to access elements at specific intervals, such as accessing only alternate elements or focusing on elements at odd or even indexes. Let's explore how this can be done
Write a program that takes an array as input and prints the alternate elements of the array.
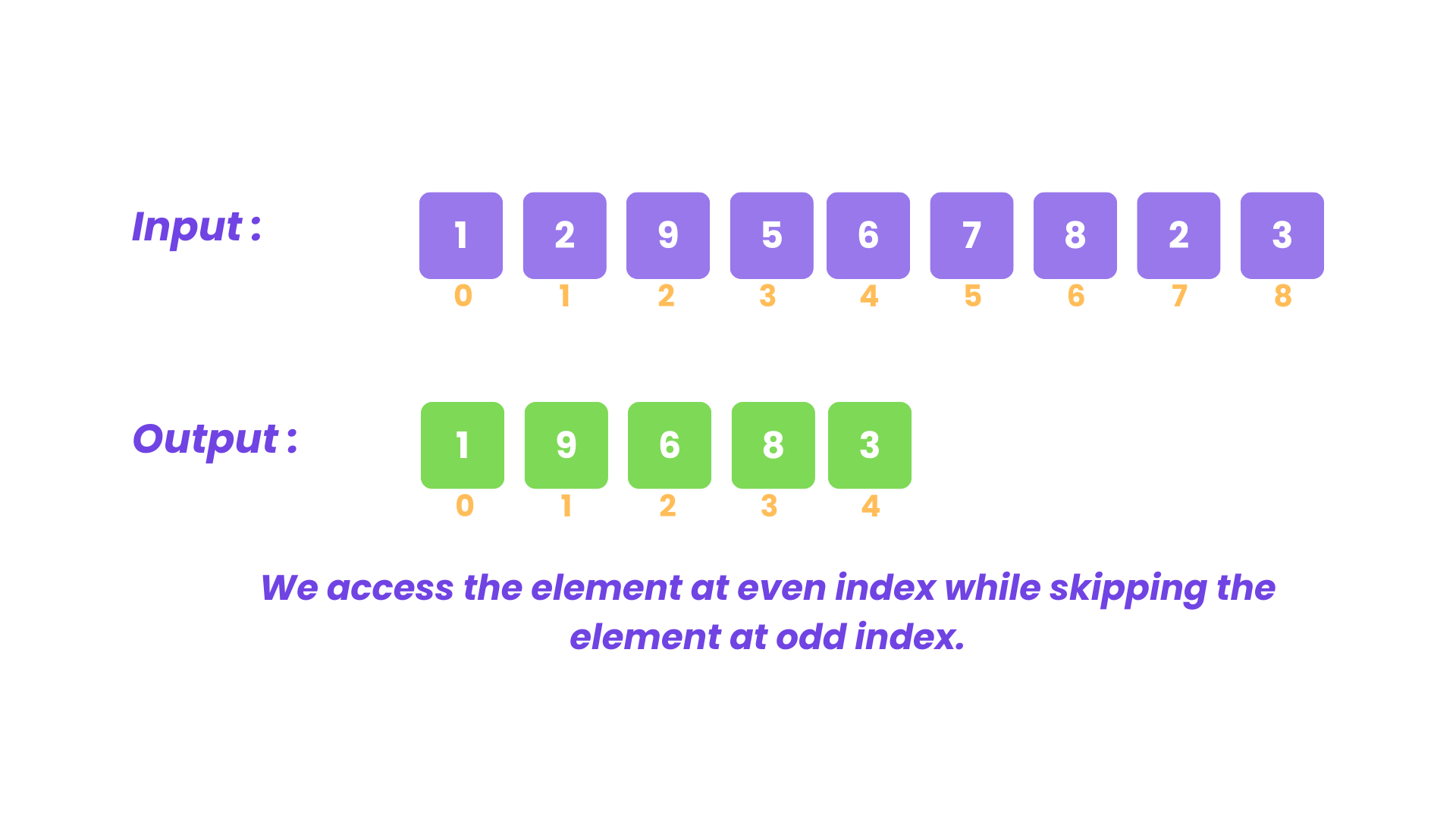
Example
Input: nums = [1, 2, 3, 5, 6]
Output: [1, 3, 6]
Explanation: Starting from first element, we access the 1st, 3rd, and 5th element while skipping the 2nd and 4th element.
Input: nums = [1, 2, 9, 5, 6, 7, 8, 2, 3]
Output: [1, 9, 6, 8, 3]
Approach
To print the values of an array nums alternately, starting from the first element (index 0), we can utilize two approaches:
- Incremental Loop Approach: Typically, we increment our loop variable by 1 for normal traversal. However, in this case, we increment the loop variable by 2. By doing so, we skip one index after each iteration, effectively printing every other element.
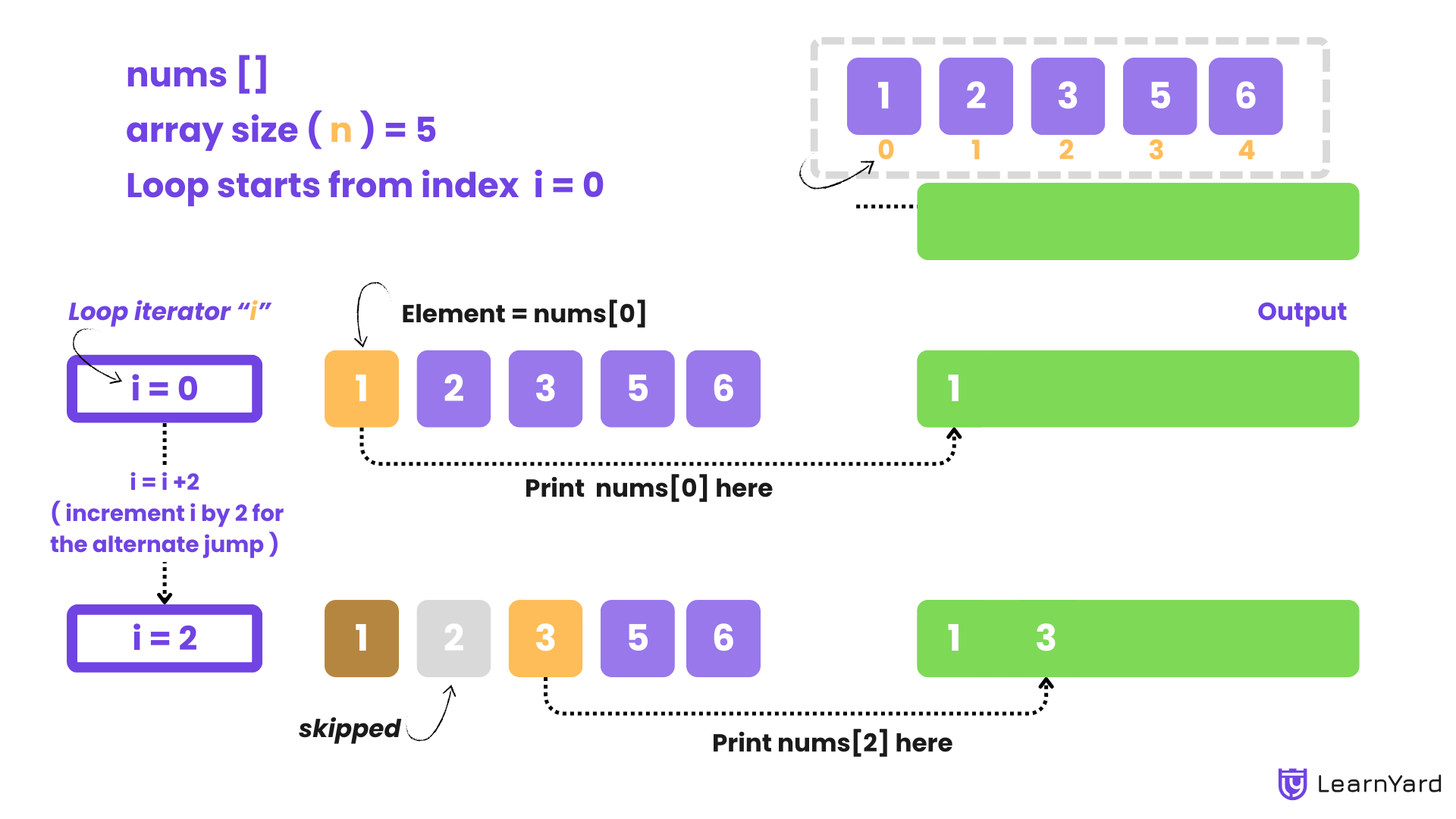
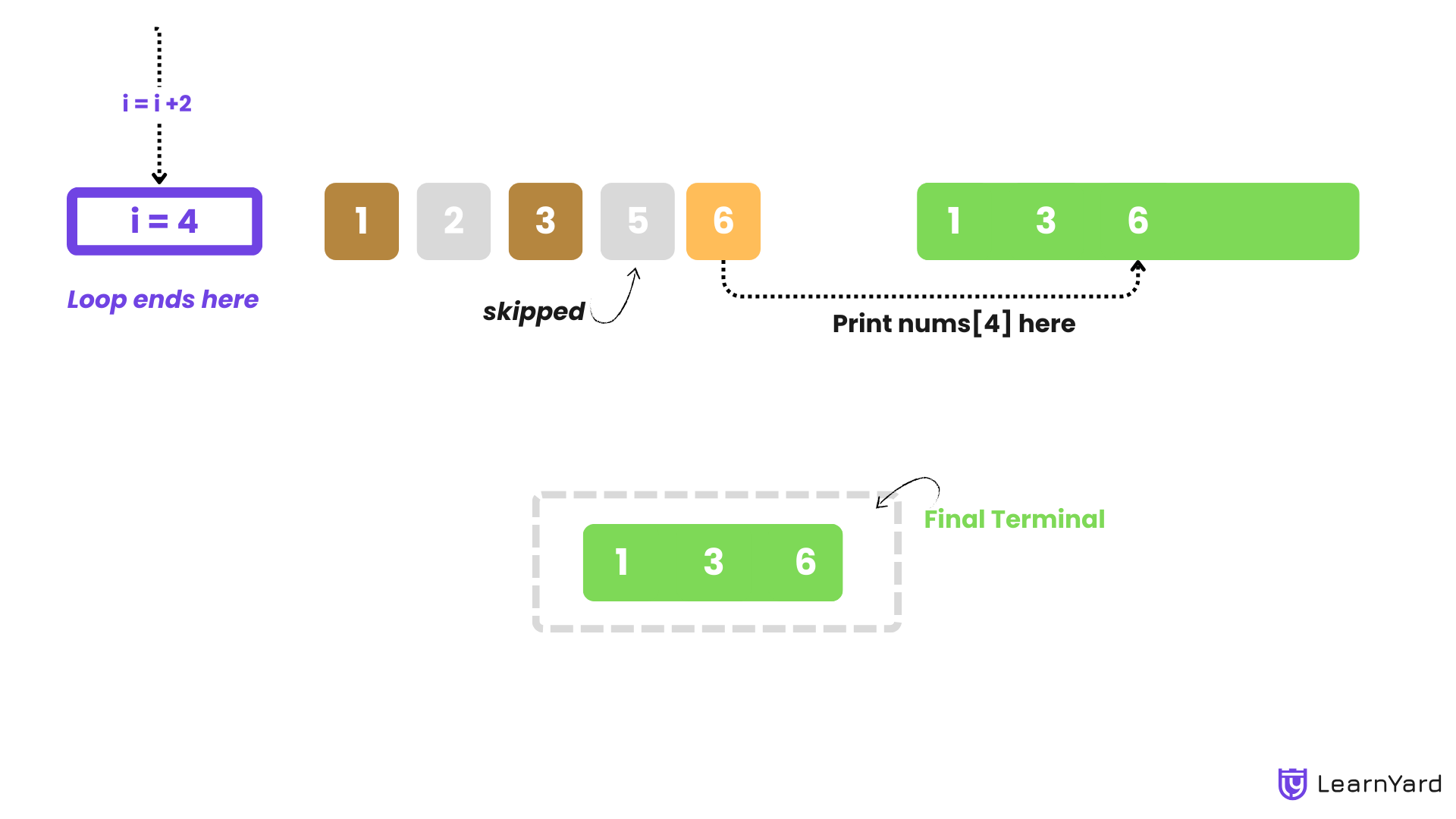
- Even Index Approach: If we observe the printed values in the example (i.e., nums[0], nums[2], nums[4]), it's clear that we need to print elements located at even indices. An index is even if it satisfies the condition index % 2 == 0. By checking this condition, we can selectively print only the elements at even positions in the array.
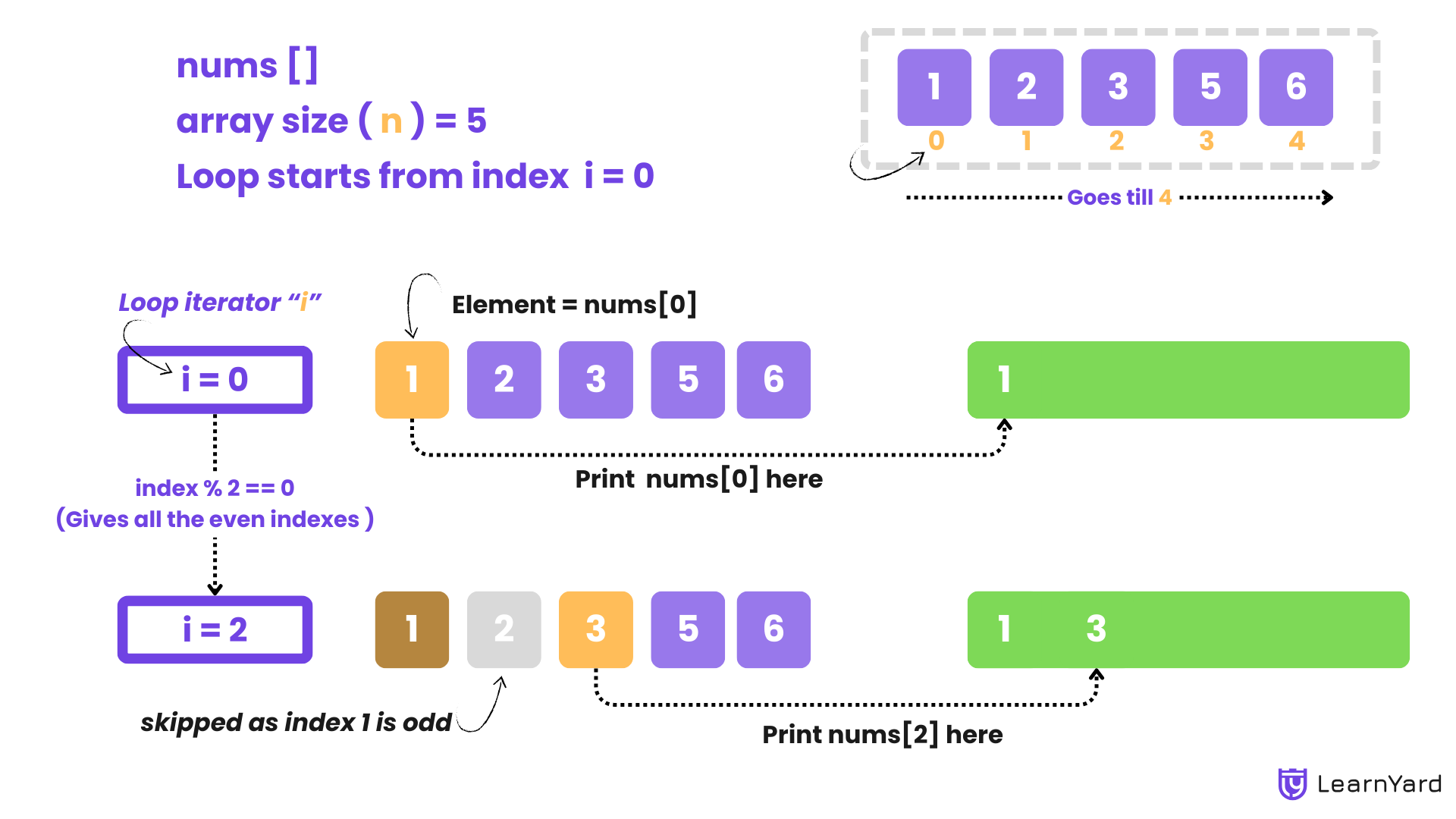
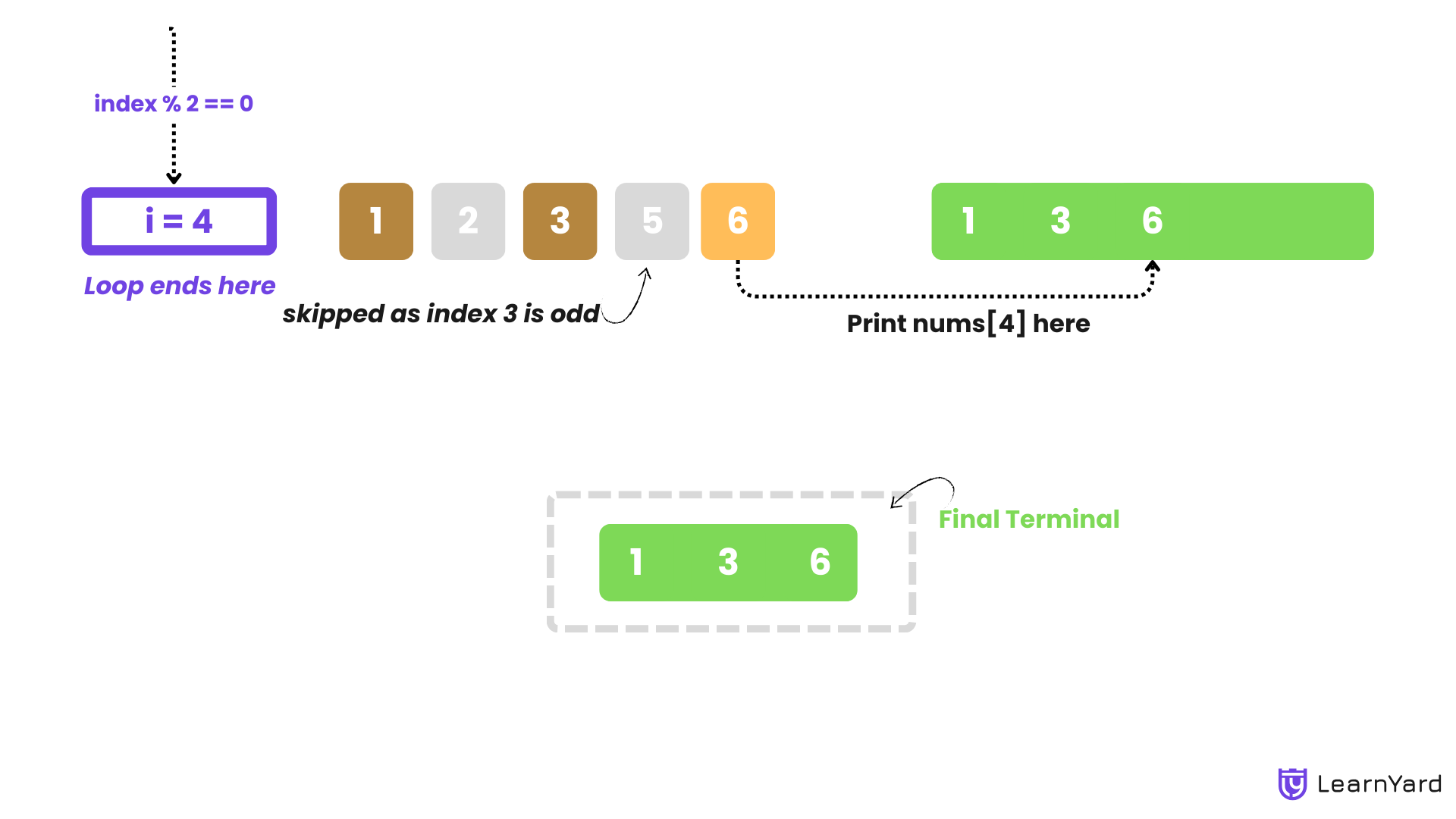
Dry Run
Input:
nums = [1, 2, 3, 5, 6]
size = 5
Output (Expected):
[1, 3, 6]
Incremental Loop Approach:
- Start with index = 0. The loop increments index by 2 in each iteration.
- First iteration: index = 0,
Element at nums[0] is 1. Add 1 to the output. - Second iteration: index = 2,
Element at nums[2] is 3. Add 3 to the output. - Third iteration: index = 4,
Element at nums[4] is 6. Add 6 to the output. - Fourth iteration: index = 6, which is out of bounds. The loop terminates.
Final output: [1, 3, 6]
Even Index Approach:
- Start with index = 0. The loop increments index by 1 in each iteration.
- First iteration: index = 0, 0 % 2 == 0.
Element at nums[0] is 1.
Add 1 to the output. - Second iteration: index = 1, 1 % 2 != 0.
Skip this index. - Third iteration: index = 2, 2 % 2 == 0.
Element at nums[2] is 3. Add 3 to the output. - Fourth iteration: index = 3, 3 % 2 != 0.
Skip this index. - Fifth iteration: index = 4, 4 % 2 == 0.
Element at nums[4] is 6. Add 6 to the output.
Final output: [1, 3, 6]
Code for All Languages
C++
// Program to Print Each alternate elements of an Array
#include <iostream>
using namespace std;
// Incremental Loop Approach: Print alternate elements by incrementing the index by 2
void printAlternateByIncrement(int nums[], int size) {
// Loop through the array, incrementing the index by 2 each time
for (int index = 0; index < size; index += 2) {
// Print the element at the current index
cout << nums[index] << " ";
}
cout << endl;
}
// Even Index Approach: Print elements at even indices
void printAlternateByEvenIndex(int nums[], int size) {
// Loop through the entire array
for (int index = 0; index < size; ++index) {
// Check if the current index is even
if (index % 2 == 0) {
// Print the element at the current index if it's even
cout << nums[index] << " ";
}
}
cout << endl;
}
Java
import java.util.*;
public class LearnYard {
// Incremental Loop Approach: Print alternate elements by incrementing the index by 2
static void printAlternateByIncrement(int[] nums, int size) {
// Loop through the array, incrementing the index by 2 each time
for (int index = 0; index < size; index += 2) {
// Print the element at the current index
System.out.print(nums[index] + " ");
}
System.out.println();
}
// Even Index Approach: Print elements at even indices
static void printAlternateByEvenIndex(int[] nums, int size) {
// Loop through the entire array
for (int index = 0; index < size; ++index) {
// Check if the current index is even
if (index % 2 == 0) {
// Print the element at the current index if it's even
System.out.print(nums[index] + " ");
}
}
System.out.println();
}
}
Python
def print_alternate_by_increment(nums, size):
# Incremental Loop Approach: Print alternate elements by incrementing the index by 2
for index in range(0, size, 2):
# Print the element at the current index
print(nums[index], end=" ")
# Move to the next line after printing all elements
print()
def print_alternate_by_even_index(nums, size):
# Even Index Approach: Print elements at even indices
for index in range(size):
# Check if the current index is even
if index % 2 == 0:
# Print the element at the current index if it's even
print(nums[index], end=" ")
# Move to the next line after printing all elements
print()
Javascript
function printAlternateByIncrement(nums, size) {
// Incremental Loop Approach: Print alternate elements by incrementing the index by 2
for (let index = 0; index < size; index += 2) {
// Print the element at the current index
process.stdout.write(nums[index] + " ");
}
// Move to the next line after printing all elements
console.log();
}
function printAlternateByEvenIndex(nums, size) {
// Even Index Approach: Print elements at even indices
for (let index = 0; index < size; ++index) {
// Check if the current index is even
if (index % 2 === 0) {
// Print the element at the current index if it's even
process.stdout.write(nums[index] + " ");
}
}
// Move to the next line after printing all elements
console.log();
}
Time Complexity: O(n)
Both approaches involve traversing the array, either by incrementing the index by 2 or by checking if the index is even. In either case, we are visiting roughly half of the elements in the array. The time complexity is therefore O(n/2), which simplifies to O(n), where n is the number of elements in the array.
Space Complexity: O(1)
Auxiliary Space Complexity
This refers to any extra space used by the algorithm that is independent of the input size.
In this case, the only additional space used is for variables such as index, i, and size, which all take up constant space. These variables do not grow with the size of the input, so the auxiliary space complexity is O(1).
Total Space Complexity
This is the space required for both the input array and any auxiliary space used by the algorithm.
The input array nums[] is of size n, which is determined by the user's input. No additional arrays or data structures are created apart from this.
So, the total space complexity is O(n).