Maximum Sum With Exactly K Elements
Problem Description
You are given a 0-indexed integer array nums and an integer k. Your task is to perform the following operation exactly k times in order to maximize your score:
1. Select an element m from nums.
2. Remove the selected element m from the array.
3. Add a new element with a value of m + 1 to the array.
4. Increase your score by m.
Return the maximum score you can achieve after performing the operation exactly k times.
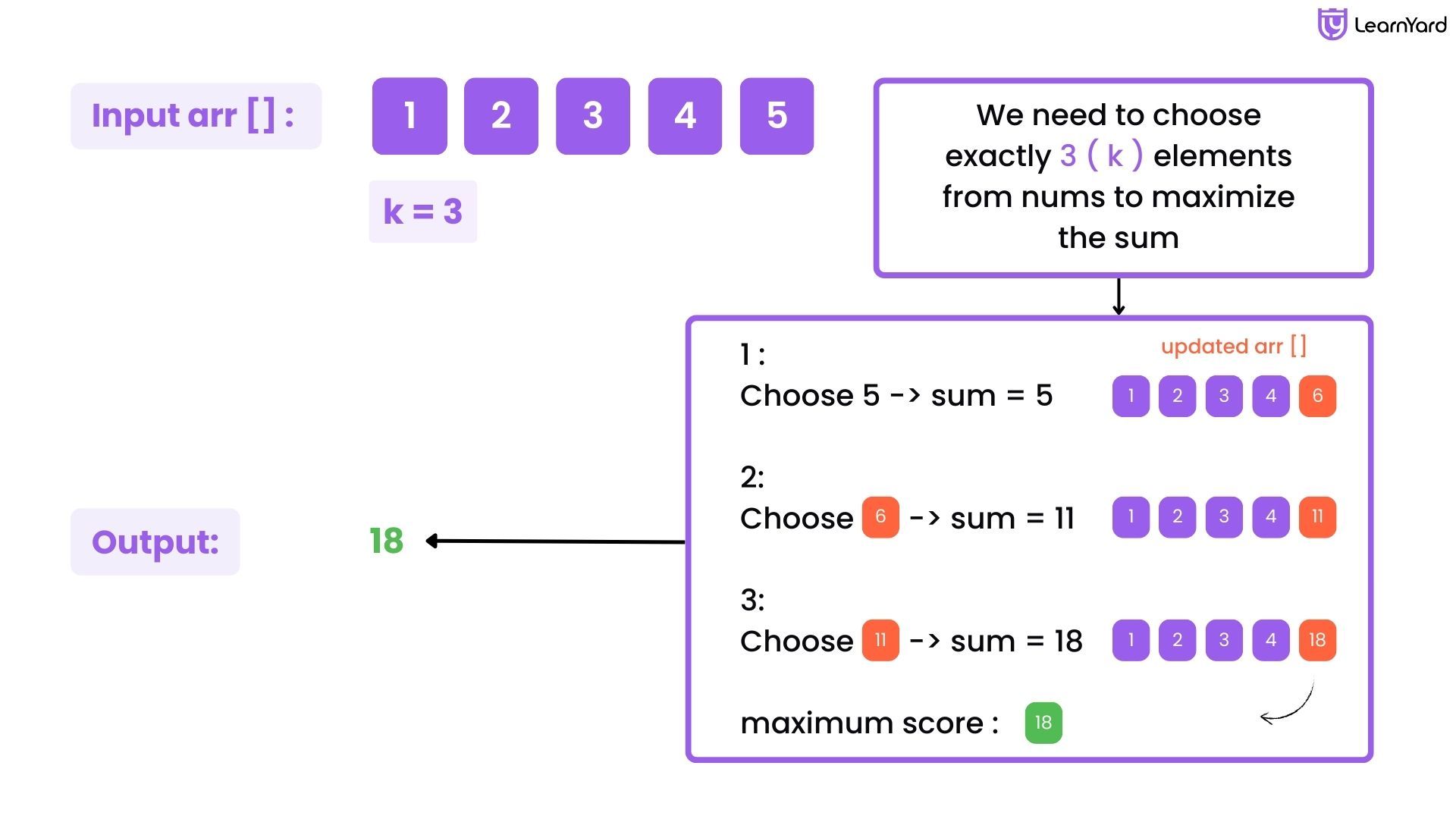
Example
Input: nums = [1,2,3,4,5], k = 3
Output: 18
Explanation: We need to choose exactly 3 elements from nums to maximize the sum.
- For the first iteration, we choose 5. Then sum is 5 and nums = [1,2,3,4,6]
- For the second iteration, we choose 6. Then sum is 5 + 6 and nums = [1,2,3,4,7]
- For the third iteration, we choose 7. Then sum is 5 + 6 + 7 = 18 and nums = [1,2,3,4,8]
So, we will return 18. It can be proven, that 18 is the maximum answer that we can achieve.
Input: nums = [5,5,5], k = 2
Output: 11
Explanation: We need to choose exactly 2 elements from nums to maximize the sum.
- For the first iteration, we choose 5. Then sum is 5 and nums = [5,5,6]
- For the second iteration, we choose 6. Then sum is 5 + 6 = 11 and nums = [5,5,7]
So, we will return 11. It can be proven, that 11 is the maximum answer that we can achieve.
Constraints
- 1 <= nums.length <= 100
- 1 <= nums[i] <= 100
- 1 <= k <= 100
The initial idea that comes to mind is to simulate the steps provided in the problem statement. Of course it will be computationally expensive, Let us see how we can build an optimal solution.
Brute Force Approach
Intuition
When solving this problem, the first idea that naturally comes to mind is to simulate the process step by step.
Stimulation
Given nums = [3, 5, 2] and k = 3.
We want to maximize the score by performing the operation exactly 3 times.
Step 1:
- Find the maximum value in the array, which is 5, located at index 1.
- Add 5 to the score. Now, the score becomes 5.
- Replace 5 with 5 + 1 = 6. The updated array becomes [3, 6, 2].
Observation: Replacing 5 with 6 is the same as simply incrementing the value at index 1.
Step 2:
- Find the maximum value in the array again, which is now 6, still located at index 1.
- Add 6 to the score. Now, the score becomes 5 + 6 = 11.
- Replace 6 with 6 + 1 = 7. The updated array becomes [3, 7, 2].
Observation: Again, we are modifying the value at index 1 by incrementing it.
Step 3:
- Find the maximum value in the array again, which is now 7, still located at index 1.
- Add 7 to the score. Now, the score becomes 11 + 7 = 18.
- Replace 7 with 7 + 1 = 8. The updated array becomes [3, 8, 2].
Observation: Once again, we are going back to the same index and incrementing its value.
Observation
From the simulation, it becomes clear that:
- We always go back to the same index for the maximum element because we are incrementing the largest value, which ensures it remains the maximum. Which means we only need to find the maximum value once.
- Replacing the maximum value with m + 1 is simply incrementing the value at the maximum index by 1.
Now let’s simplify this further. Since we are only concerned with the maximum element, the process can be broken down into two parts: If we think about it, this means we are essentially adding the maximum element k times to our score, but every time we add it, its value increases by 1 (because of the +1 operation).
- Add the current maximum element to the score.
- Increment that maximum element by 1 for the next operation.
Approach
Step 1: Initialize a score variable to 0. This variable will store the cumulative score as we perform the operation.
Step 2: Find the maximum element in the array. This is done just once at the beginning, as the maximum will be updated with each operation.
Step 3: Loop k times:
- In each iteration, add the current maximum element to the score.
- Then, increment the maximum element by 1 to simulate the "add m + 1" operation. This updates the value of the maximum element, and it will be used in the next iteration.
Step 4: Once the loop is complete, return the final score, which is the total score accumulated over k iterations.
Let us understand with this a video,
Dry Run
Initial Setup:
- nums = [1, 2, 3, 4, 5]
- k = 3
- score = 0
We need to choose 3 elements from the array to maximize the sum. At each step, we will:
- Find the maximum element in the array.
- Add this element to the score.
- Increment that maximum element by 1 for the next operation.
Step-by-Step Dry Run:
First Iteration (k = 1):
Initial array: nums = [1, 2, 3, 4, 5]
Maximum element: 5 (this is the largest value in the array).
Add the maximum element (5) to the score.
- Score = 0 + 5 = 5
- Increment the maximum element by 1: The element 5 is replaced with 6, so the updated array becomes: nums = [1, 2, 3, 4, 6]
At the end of this iteration:
- Score = 5
- Array after update: nums = [1, 2, 3, 4, 6]
Second Iteration (k = 2):
Current array: nums = [1, 2, 3, 4, 6]
Maximum element: 6 (this is now the largest value in the array).
Add the maximum element (6) to the score:
- Score = 5 + 6 = 11
- Increment the maximum element by 1: The element 6 is replaced with 7, so the updated array becomes: nums = [1, 2, 3, 4, 7]
At the end of this iteration:
- Score = 11
- Array after update: nums = [1, 2, 3, 4, 7]
Third Iteration (k = 3):
Current array: nums = [1, 2, 3, 4, 7]
Maximum element: 7 (this is now the largest value in the array).
Add the maximum element (7) to the score:
- Score = 11 + 7 = 18
- Increment the maximum element by 1: The element 7 is replaced with 8, so the updated array becomes: nums = [1, 2, 3, 4, 8]
At the end of this iteration:
- Score = 18
- Array after update: nums = [1, 2, 3, 4, 8]
Final Result:
Score = 18
The process stops after 3 iterations, and the final score is 18.
Code for All Languages
C++
class Solution {
public:
// Function to maximize the score by performing the operation exactly k times
int maximizeSum(vector<int>& nums, int k) {
// Step 1: Find the initial maximum element in the array
int maxElement = *max_element(nums.begin(), nums.end());
// Step 2: Initialize the score variable to 0
int score = 0;
// Step 3: Perform the operation k times
for (int i = 0; i < k; i++) {
// Add the current maximum element to the score
score += maxElement;
// Increment the maximum element by 1 for the next iteration
maxElement += 1;
}
// Return the final score after performing the operation k times
return score;
}
};
Java
class Solution {
// Function to maximize the score by performing the operation exactly k times
public int maximizeSum(int[] nums, int k) {
// Step 1: Find the initial maximum element in the array
int max_element = Integer.MIN_VALUE;
for (int num : nums) {
max_element = Math.max(max_element, num);
}
// Step 2: Initialize the score variable to 0
int score = 0;
// Step 3: Perform the operation k times
for (int i = 0; i < k; i++) {
// Add the current maximum element to the score
score += max_element;
// Increment the maximum element by 1 for the next iteration
max_element += 1;
}
// Return the final score after performing the operation k times
return score;
}
}
Python
class Solution:
# Function to maximize the score by performing the operation exactly k times
def maximizeSum(self, nums: List[int], k: int) -> int:
# Step 1: Find the initial maximum element in the array
max_element = max(nums)
# Step 2: Initialize the score variable to 0
score = 0
# Step 3: Perform the operation k times
for i in range(k):
# Add the current maximum element to the score
score += max_element
# Increment the maximum element by 1 for the next iteration
max_element += 1
# Return the final score after performing the operation k times
return score
Javascript
// Function to maximize the score by performing the operation exactly k times
var maximizeSum = function(nums, k) {
// Step 1: Find the initial maximum element in the array
let maxElement = Math.max(...nums);
// Step 2: Initialize the score variable to 0
let score = 0;
// Step 3: Perform the operation k times
for (let i = 0; i < k; i++) {
// Add the current maximum element to the score
score += maxElement;
// Increment the maximum element by 1 for the next iteration
maxElement += 1;
}
// Return the final score after performing the operation k times
return score;
};
Time Complexity : O(n + k)
Finding Maximum Element:
- Finding the maximum element takes O(n) time to find the maximum element in the array nums.
Loop Execution:
- The loop runs k times, and inside each iteration, we perform constant time operations (adding to the score and incrementing maxElement), so it takes O(k) time.
Total Time Complexity:
- O(n) for finding the maximum and O(k) for the loop.
- Final Time Complexity: O(n + k)
Space Complexity : O(1)
Auxiliary Space Complexity:
- The auxiliary space refers to the extra space used by the algorithm aside from the input.
- In this case, we are only using a few variables (maxElement, score, and loop counter), which require a constant amount of space.
- Auxiliary Space Complexity: O(1)
Total Space Complexity:
- The total space includes both the input and the auxiliary space.
- The input array nums takes O(n) space, but we are not using any extra space proportional to the size of the input (apart from the constants for variables).
- Total Space Complexity: O(n) for the input array, but the extra space used by the algorithm is O(1).
The previous solution works well, but we can do even better! By thinking mathematically, we can eliminate the extra O(k) operations and directly compute the result in a more efficient way. Let’s explore how to optimize this further.
Optimal Approach
Intuition
If we look closely at what we are doing in each iteration:
- We add the current maximum element, say maxElement, to the score.
- Then we increment maxElement by 1 and repeat.
After k iterations, the numbers added to the score will look like this
maxElement + (maxElement + 1) + (maxElement + 2) + ... + (maxElement + k - 1)
This is nothing but the sum of the first k terms of an arithmetic progression (AP) where:
- The first term (a) is maxElement.
- The common difference (d) is 1.
- The number of terms (n) is k.
Arithmetic Progression Formula
The sum of the first n
terms of an AP can be calculated using the formula:
Sum = n/2 * (2a + (n - 1) * d)
- Here, a is the first term.
- d is the common difference.
- n is the number of terms.
Applying the Formula:
In our case:
- a = maxElement
- d = 1 (as we increment by 1 each time)
- n = k (we perform the operation k times)
Substituting these values into the formula:
Sum = k / 2 * (2 * maxElement + (k - 1) * 1)
This simplifies to:
Sum = k / 2 * (2 * maxElement + k - 1)
Approach
Step 1: Find the Maximum Element
- First, identify the largest number in the array, nums. This will be the starting point for our arithmetic progression.
- Iterate through the array or use a built-in function to find the maximum element.
Step 2: Apply the Arithmetic Progression Formula
- Once you have the maximum element (maxElement), calculate the total score using the arithmetic progression formula:
Sum = k / 2 * (2 * maxElement + k - 1) - Break the formula into smaller steps for clarity:
- Compute the first term as 2 * maxElement.
- Add (k - 1) to the first term.
- Multiply the result by k.
- Divide the entire result by 2.
Step 3: Return the Result
- Return the result as the maximum score after performing the operations.
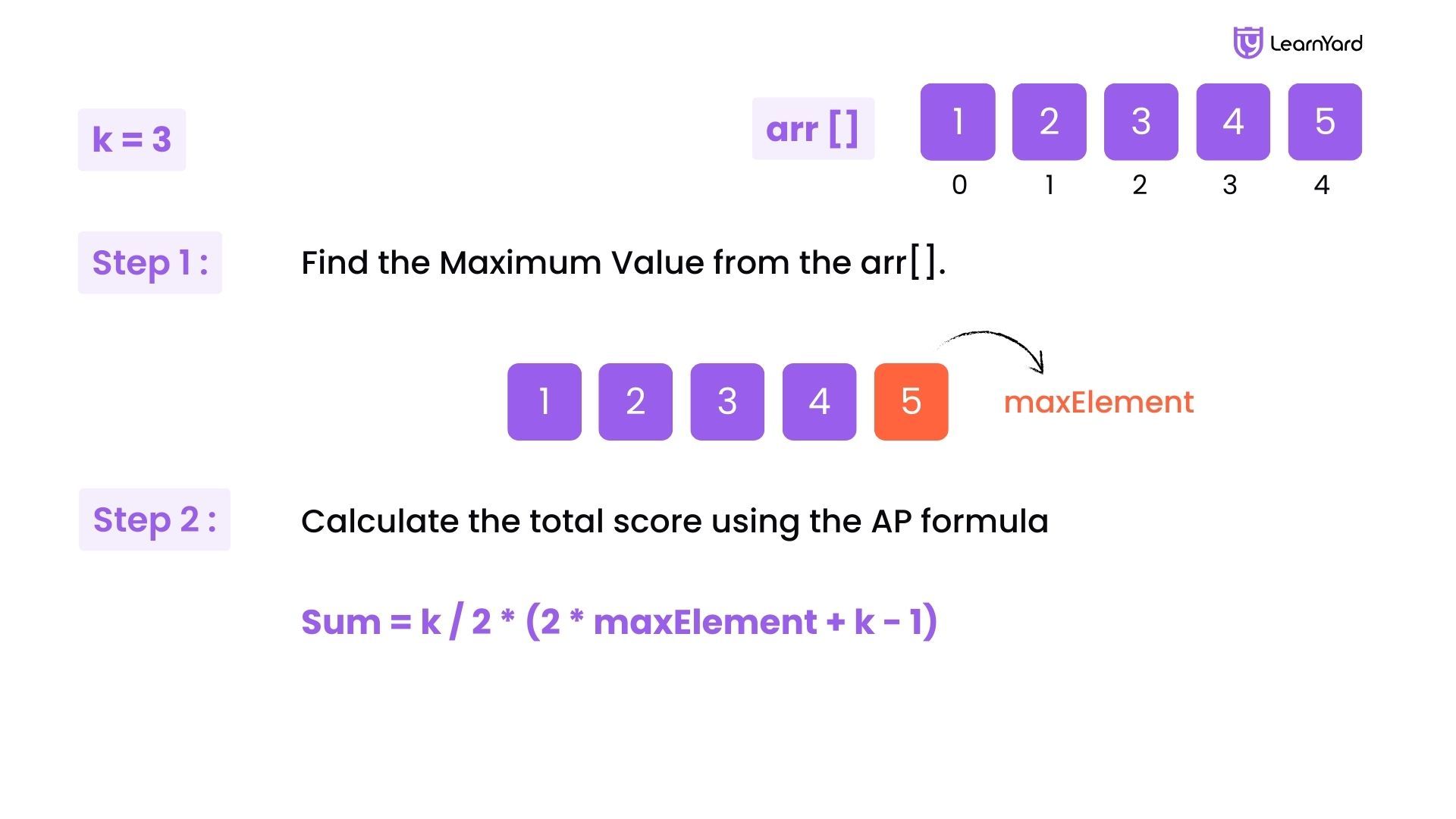
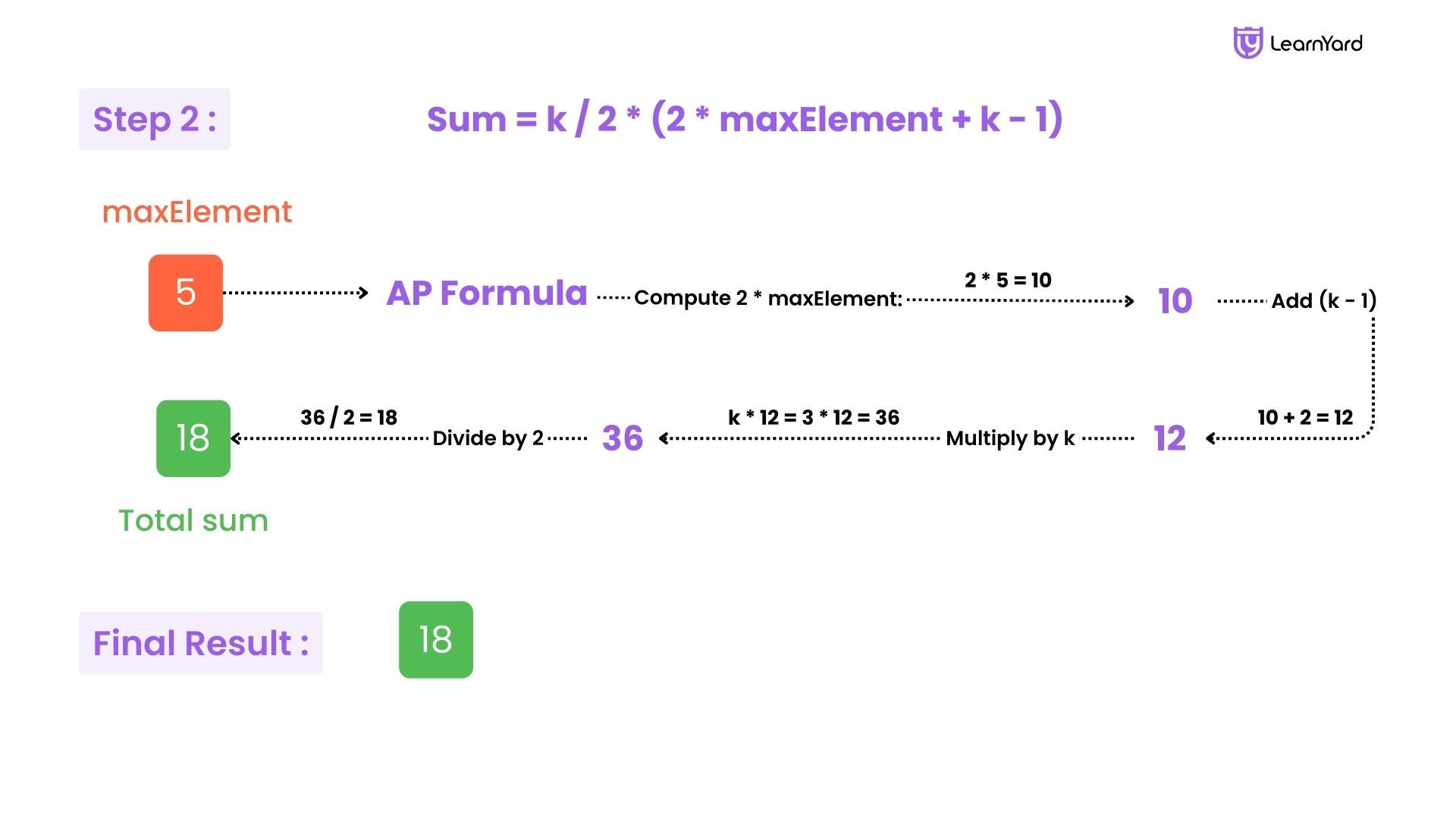
Dry Run
Initial Setup:
- nums = [1, 2, 3, 4, 5]
- k = 3
We will calculate the total score using the arithmetic progression formula:
Sum = k / 2 * (2 * maxElement + k - 1)
Step-by-Step Dry Run:
Step 1: Find the Maximum Element
- The array is nums = [1, 2, 3, 4, 5].
- Maximum element: maxElement = 5.
Step 2: Break Down the Formula We calculate the total score using the
AP formula:
- Compute 2 * maxElement:
- 2 * maxElement = 2 * 5 = 10.
- Add (k - 1) to the result:
- 10 + (k - 1) = 10 + 2 = 12.
- Multiply the result by k:
- k * 12 = 3 * 12 = 36.
- Divide by 2 to get the total sum:
- 36 / 2 = 18.
Final Result:
- The total score after 3 operations is 18.
Code for All Languages
C++
class Solution {
public:
// Function to maximize the score by calculating the sum using the AP formula
int maximizeSum(vector<int>& nums, int k) {
// Step 1: Find the initial maximum element in the array
int maxElement = *max_element(nums.begin(), nums.end());
// Step 2: Use the arithmetic progression formula to calculate the score
// Sum = k / 2 * (2 * maxElement + k - 1)
int score = k * (2 * maxElement + k - 1) / 2;
// Return the final score
return score;
}
};
Java
class Solution {
// Function to maximize the score using the optimized AP formula
public int maximizeSum(int[] nums, int k) {
// Step 1: Find the initial maximum element in the array
int max_element = Integer.MIN_VALUE;
for (int num : nums) {
max_element = Math.max(max_element, num);
}
// Step 2: Use the arithmetic progression formula to calculate the score
// Sum = k * (2 * max_element + k - 1) / 2
int score = k * (2 * max_element + k - 1) / 2;
// Return the final score
return score;
}
}
Python
class Solution:
# Function to maximize the score using the optimized AP formula
def maximizeSum(self, nums: List[int], k: int) -> int:
# Step 1: Find the initial maximum element in the array
max_element = max(nums)
# Step 2: Use the arithmetic progression formula to calculate the score
# Sum = k * (2 * max_element + k - 1) // 2
score = k * (2 * max_element + k - 1) // 2
# Return the final score
return score
Javascript
// Function to maximize the score using the optimized AP formula
var maximizeSum = function(nums, k) {
// Step 1: Find the initial maximum element in the array
let maxElement = Math.max(...nums);
// Step 2: Use the arithmetic progression formula to calculate the score
// Formula: Sum = k * (2 * maxElement + k - 1) / 2
let score = k * (2 * maxElement + k - 1) / 2;
// Return the final score
return score;
};
Time Complexity : O(n)
- Finding the Maximum Element:
- To find the maximum element in the array nums, we iterate through the array once.
- This operation takes O(n) time.
- Using the AP Formula:
- The arithmetic progression formula is a constant-time operation.
- This step takes O(1) time.
- Total Time Complexity:
- Adding the complexities from both steps, the overall time complexity is: O(n)+O(1) = O(n)
Final Time Complexity: O(n)
Space Complexity : O(1)
Auxiliary Space Complexity:
- The auxiliary space refers to the extra space used by the algorithm aside from the input.
- In this case, we are using only a few variables (maxElement, score, and loop counters), which require a constant amount of space.
- Auxiliary Space Complexity: O(1)
Total Space Complexity:
- The total space includes both the input and the auxiliary space.
- The input array nums takes O(n) space, but we are not using any extra space proportional to the size of the input (apart from the constants for variables).
Total Space Complexity: O(n) for the input array, with an additional O(1) for the algorithm.
Learning Tip
Now we have successfully tackled this problem, let's try these similar problems.
A circular typewriter has lowercase English letters 'a' to 'z' with a pointer initially at 'a'.
In each second, you can move the pointer one step clockwise or counterclockwise or type the current character.
Given a string word, return the minimum time in seconds to type the word.
Given an integer array nums, determine the minimum number of increments needed to make the array strictly increasing, where nums[i] < nums[i+1] for all valid indices.