Create Duplicate of an Array
When working with arrays, there are situations where we may need to perform operations or modifications on an array while preserving the original data. In such cases, it's common to create a copy of the original array. By doing this, any modifications or operations can be performed on the duplicate array, ensuring that the original array remains unchanged. This approach allows us to retain the original array in its unaltered state while working on the duplicate.
Write a program that takes an array as input and creates a duplicate of the given array.
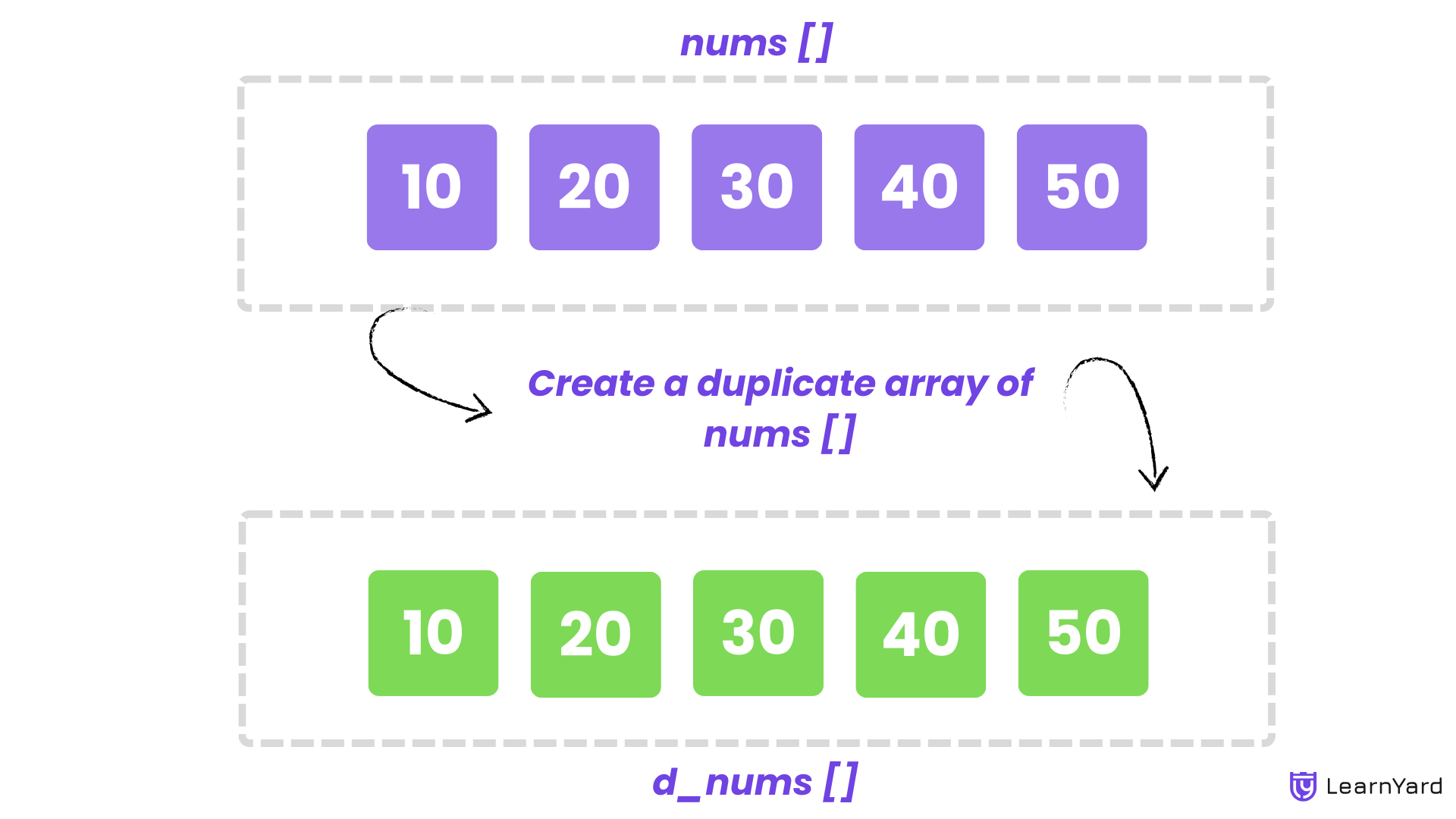
Example:
Input: nums = [4, 8, 15, 16, 23, 42]
Output: [4, 8, 15, 16, 23, 42]
Explanation: The duplicate array is identical to the input array, preserving the original array for future use.
Input: nums = [10, 20, 30, 40, 50]
Output: [10, 20, 30, 40, 50]
Approach
To create a duplicate array of nums called d_nums, we first need to declare d_nums with the same size as nums. After the declaration, we will assign values to each element in d_nums such that they match the corresponding elements in nums. This will be done by iterating over the array nums, and for every index i from 0 to n-1 (where n is the size of nums), we will set d_nums[i] = nums[i]. This process ensures that each element in d_nums is an exact copy of the element at the same index in nums.
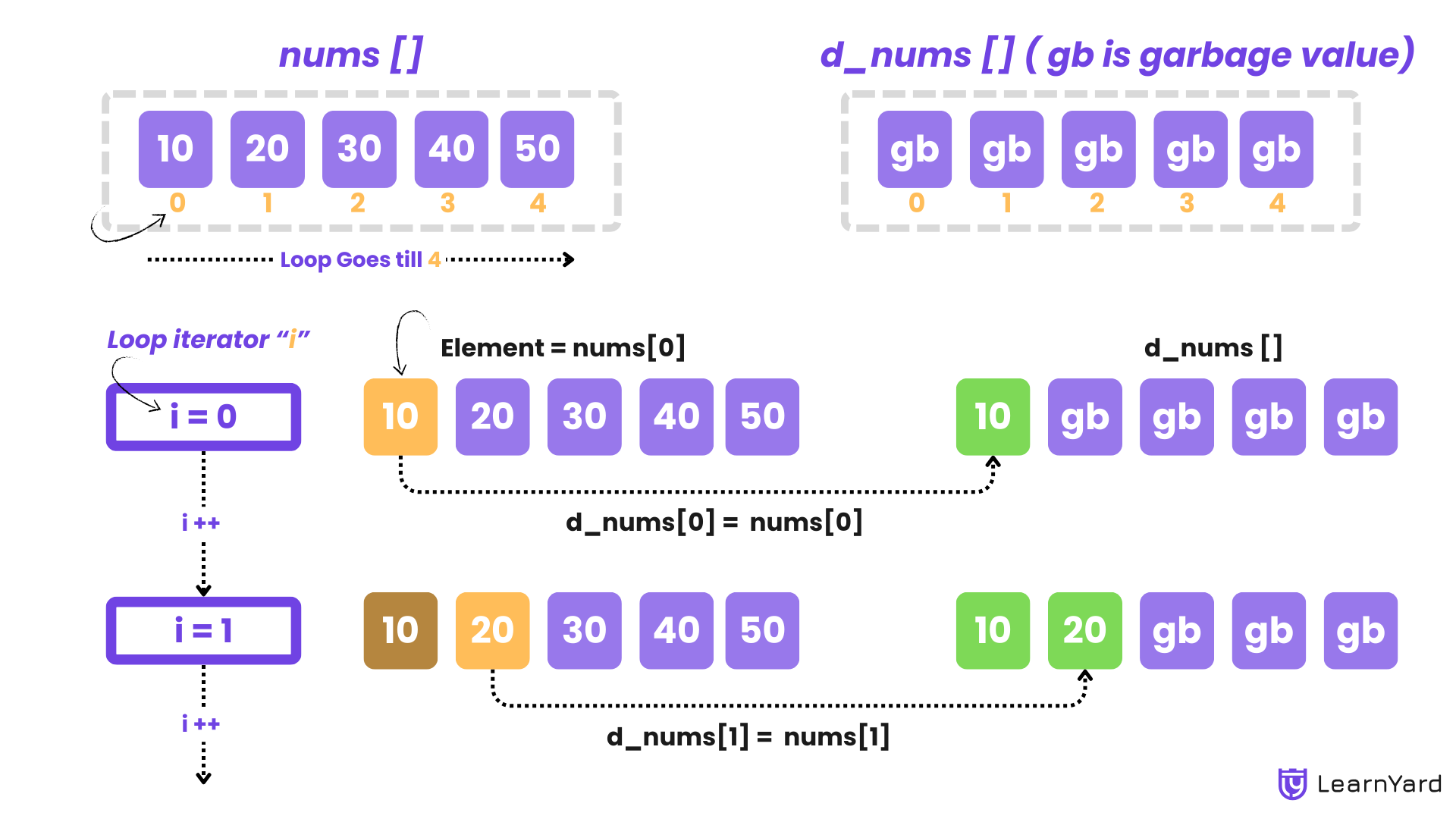
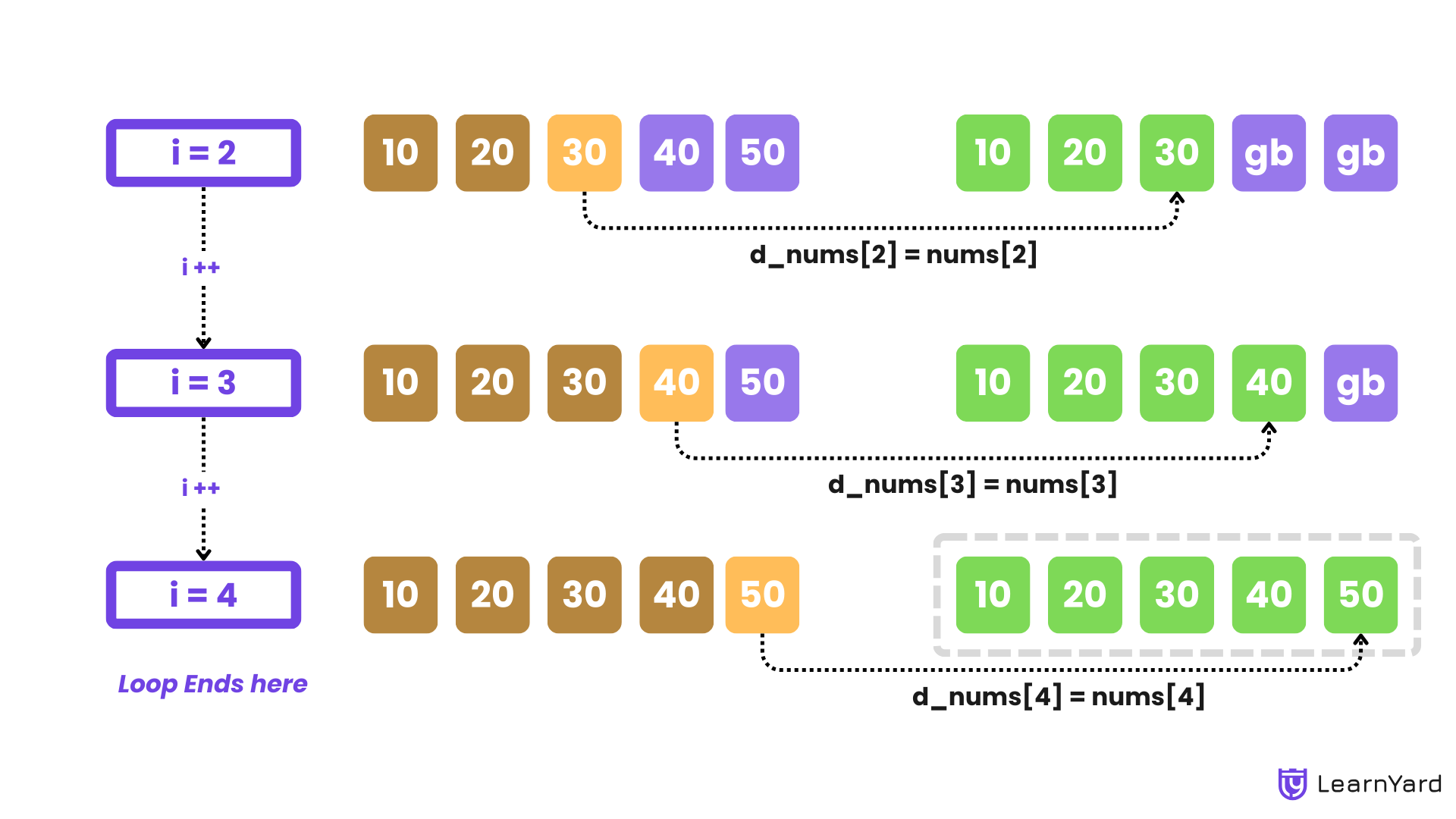
Dry Run
Input: nums = [10, 20, 30, 40, 50], size = 5
Output: [10, 20, 30, 40, 50]
- A new array d_nums is declared with the same size as nums.
Initially, d_nums = [ gb, gb, gb, gb, gb ] (empty array of size 5). - Start copying elements from nums to d_nums:
- Iteration 1 (index = 0): d_nums[0] = nums[0]
d_nums = [10, gb, gb, gb, gb ] - Iteration 2 (index = 1): d_nums[1] = nums[1]
d_nums = [10, 20, gb, gb, gb ] - Iteration 3 (index = 2): d_nums[2] = nums[2]
d_nums = [10, 20, 30, gb, gb ] - Iteration 4 (index = 3): d_nums[3] = nums[3]
d_nums = [10, 20, 30, 40, gb ] - Iteration 5 (index = 4): d_nums[4] = nums[4]
d_nums = [10, 20, 30, 40, 50]
- Iteration 1 (index = 0): d_nums[0] = nums[0]
Final Output : The duplicate array is printed exactly as the input array: [10, 20, 30, 40, 50]
Time Complexity : O(n)
The time complexity of the approach is determined by the iteration over the array nums. We iterate through each element of nums exactly once to copy it into d_nums. If n is the size of the array nums, the loop runs n times, and each assignment operation (d_nums[i] = nums[i]) takes constant time, O(1). Therefore, the total time complexity is O(n)
Space Complexity : O(n)
Auxiliary Space Complexity: This refers to any extra space used by the algorithm that is independent of the input space and output space. In this case, we only use a constant amount of extra space, specifically for the variables. These variables do not depend on the size of the array and therefore take up constant space. so the auxiliary space complexity is O(1).
Total Space Complexity: This includes the space required for the input, output and extra space used by the algorithm as well. The input array nums[] is of size n, So the space required for input space is O(n). Output space is used as we make a duplicate array of same length i.e. n, so it takes O(n) space. Also, the algorithm takes only constant extra space.
Total Space Complexity = O(n) + O(n) + O(1) = O(n+n) = O(2n) = O(n)
Join Our Course
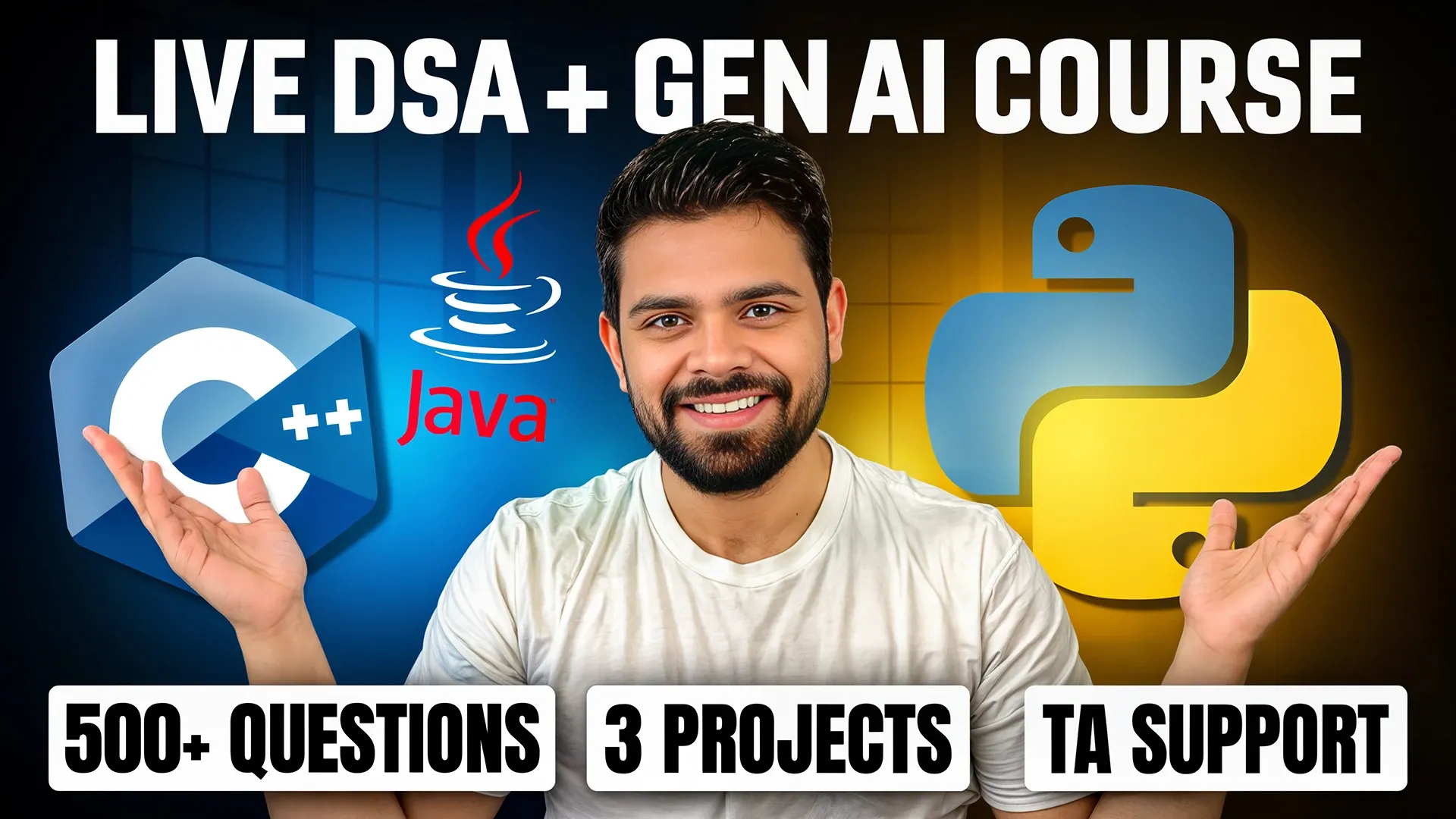
Offer ends in:
Days
Hours
Mins
Secs