Check Palindrome
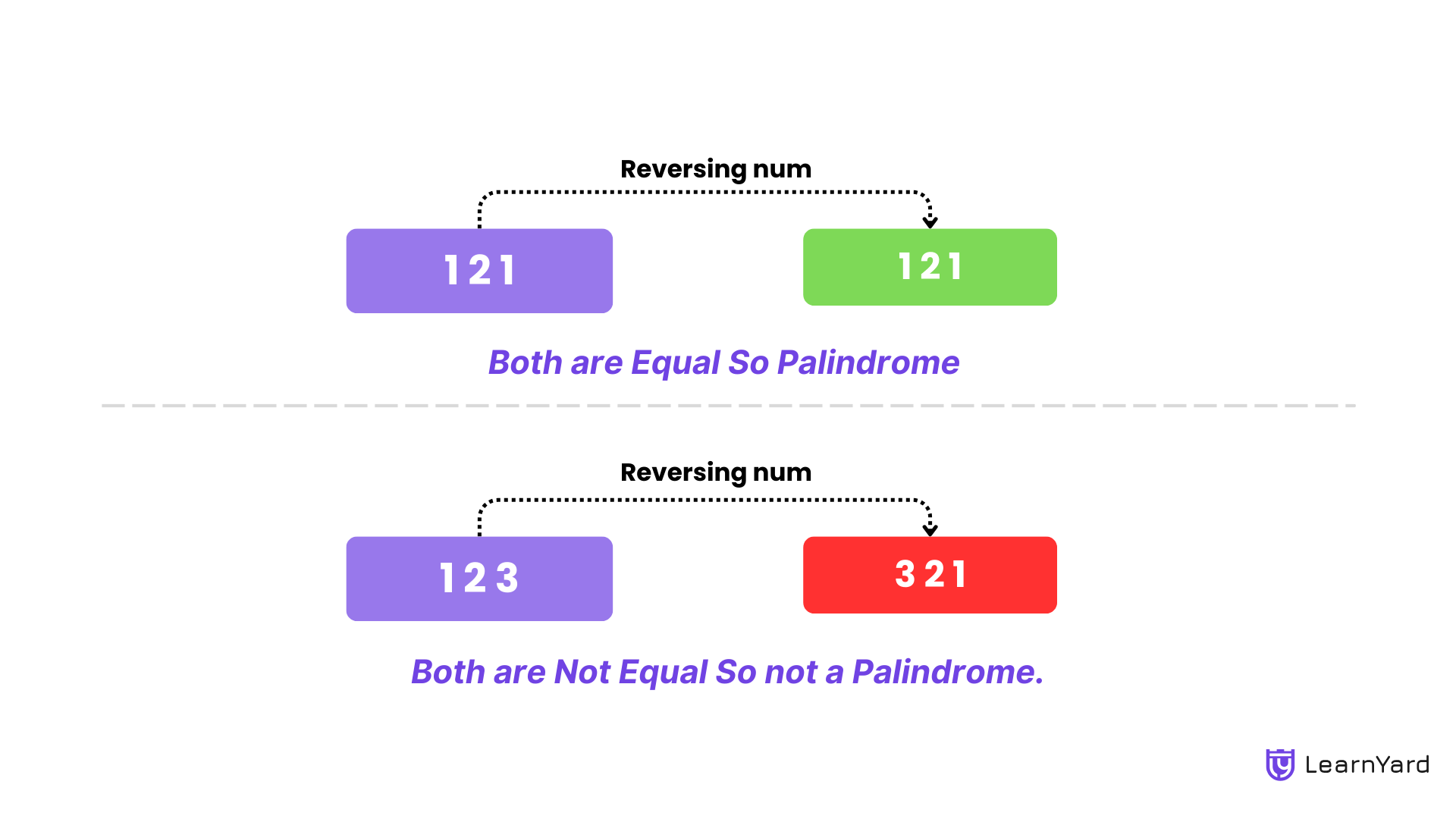
Given a non-negative integer num, check whether the given number is palindrome or not.
What is a palindrome?
A palindrome is a word, phrase, number, or any other sequence of characters that reads the same forward and backward.
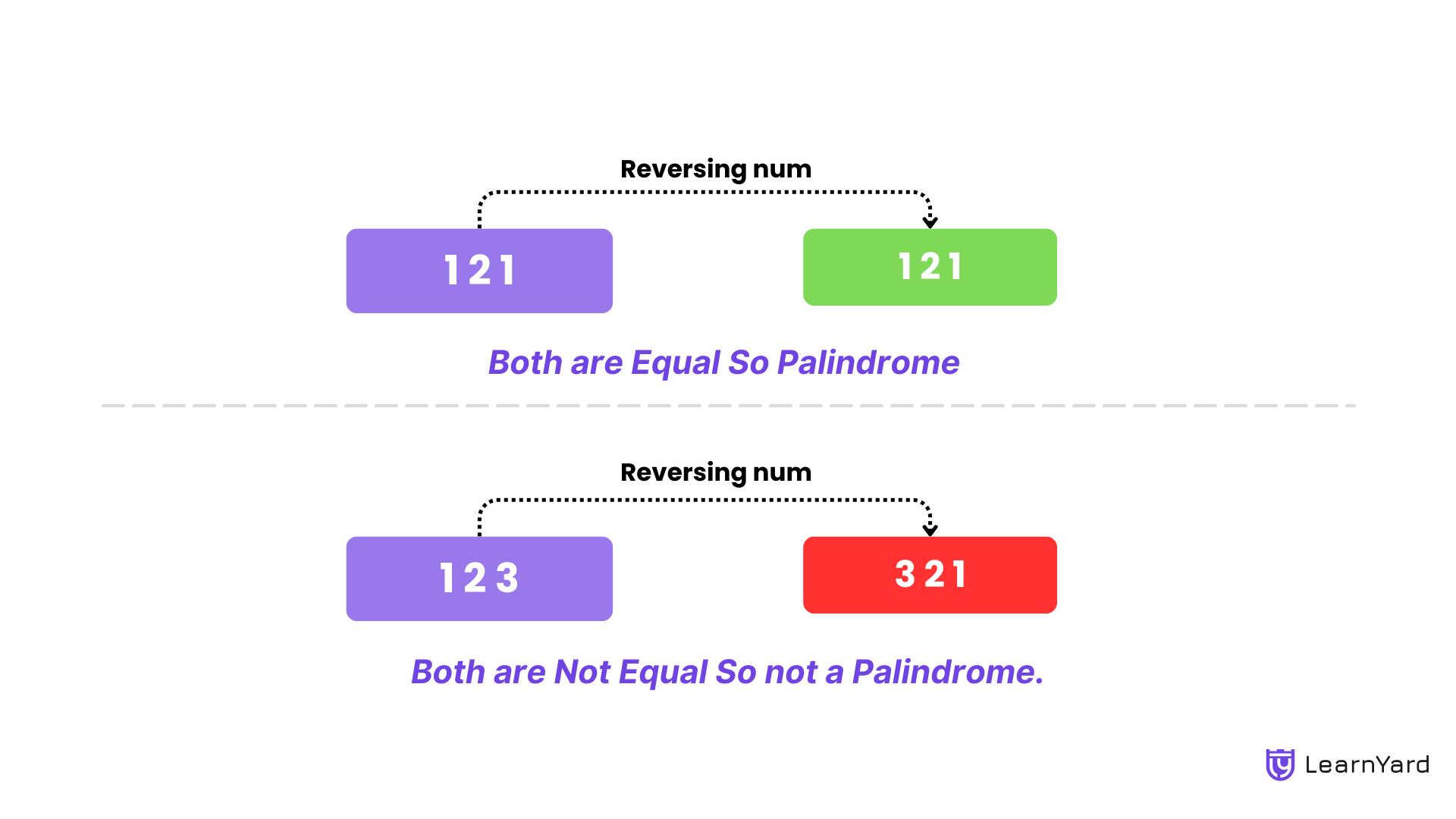
Examples
Input: 121
Output: true
Explanation: The number 121 reads the same forward and backward.
Input: 123
Output: false
Explanation: The number 123 does not read the same backward (321), so it is not a palindrome.
Approach
The idea is to find the reverse of the original number and then compare the reversed number with the original number. If the reversed number is same as the original number, the number is Palindrome. Otherwise, the number is not a Palindrome.
Since we have learned about the concept of extracting the digits from a number and reversing a number let us now understand how those concepts will help with checking whether the given number is palindrome or not.
How to check whether a given number is palindrome or not?
To check whether a given number num is a palindrome, reverse the digits of the number num and store it in another variable. Check if the original number is equal to the reversed number. If the original and reversed numbers are the same, then the number is a palindrome otherwise, it is not.
Dry Run
Initial values:
num = 121, originalNumber = 121, and reversedNumber = 0.
First Iteration:
Dividing num by 10, we get quotient = 12 and remainder = 1 (last digit of 121).
Now, num = 12 and reversedNumber = reversedNumber × 10 + 1 = 0 × 10 + 1 = 1.
Second Iteration:
Dividing num by 10, we get quotient = 1 and remainder = 2 (last digit of 12).
Now, num = 1 and reversedNumber = reversedNumber × 10 + 2 = 1 × 10 + 2 = 12.
Third Iteration:
Dividing num by 10, we get quotient = 0 and remainder = 1 (last digit of 1).
Now, num = 0 and reversedNumber = reversedNumber × 10 + 1 = 12 × 10 + 1 = 121.
Final Check:
Since reversedNumber (121) == originalNumber (121), the number is a Palindrome.
Output: 121 is a Palindrome
Code Implementation
C++ Code Try on Compiler!
Java Code Try on Compiler!
Python Code Try on Compiler!
Javascript Code Try on Compiler!
Time Complexity : O(logn)
The time complexity arises from the number of digits in the number. The number of times the while loop runs depends on the number of digits in the given number num.
For a given number num in a decimal representation, the number of digits is approximately equal to log10(num). This is because each digit represents a power of 10.
The loop executes once for each digit in num.
Therefore, the loop runs log10(num) times, which simplifies to O(logn).
Space Complexity : O(1)
We are using only variables like reversedNumber, and originalnumber which use constant space.
Since the space required by these variables does not depend on the size of the input number num, the auxiliary space is O(1).
Now since we have learned about how to extract digits from a number, let's solve another problem that uses the concept of digit extracting.
Similar Problems
Given an integer num, repeatedly add all its digits until the result has only one digit, and return it.
Given a signed 32-bit integer x, return x with its digits reversed, with some conditions.
Join Our Course
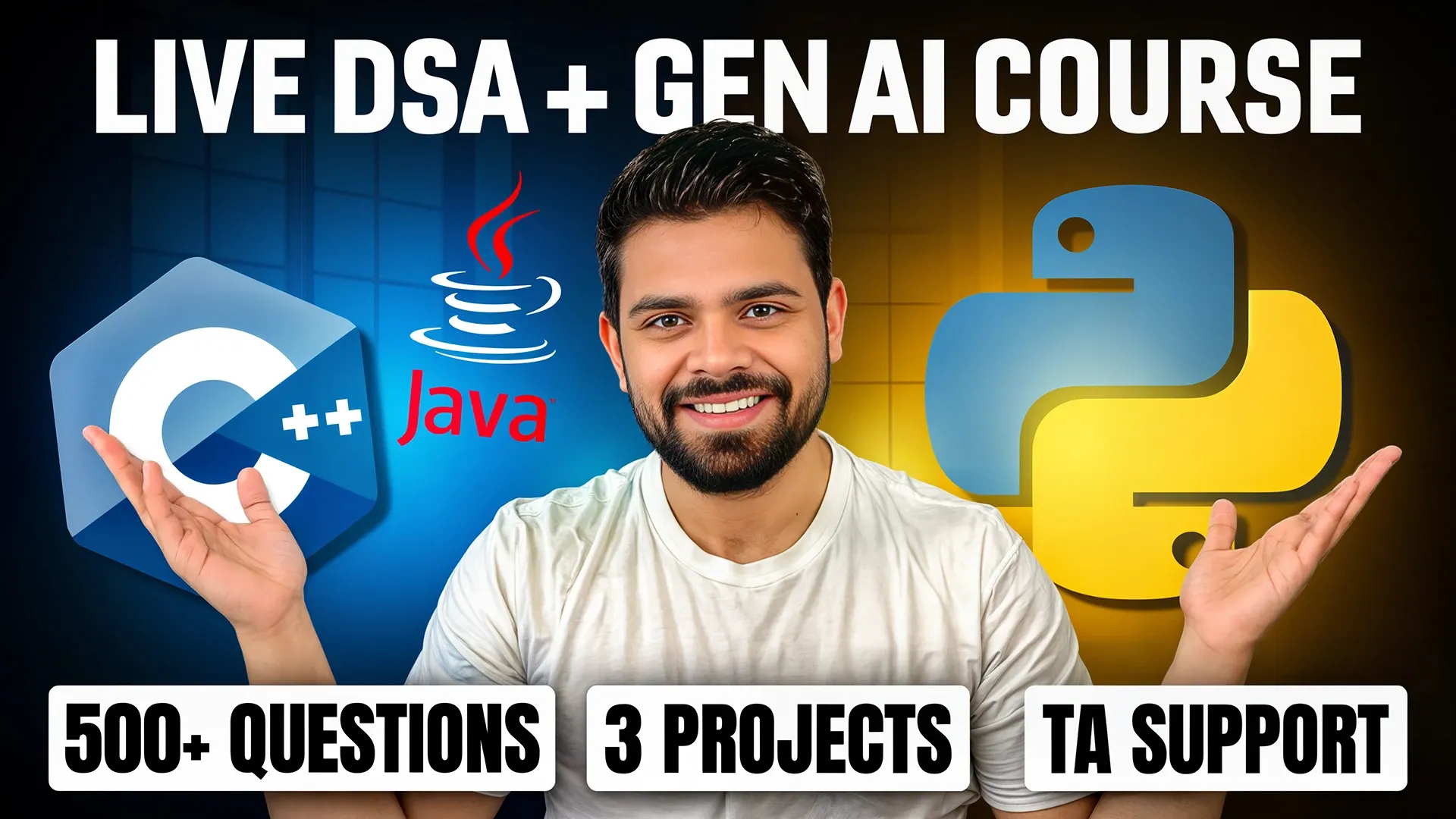
Offer ends in:
Days
Hours
Mins
Secs