Check if a Number is an Armstrong Number
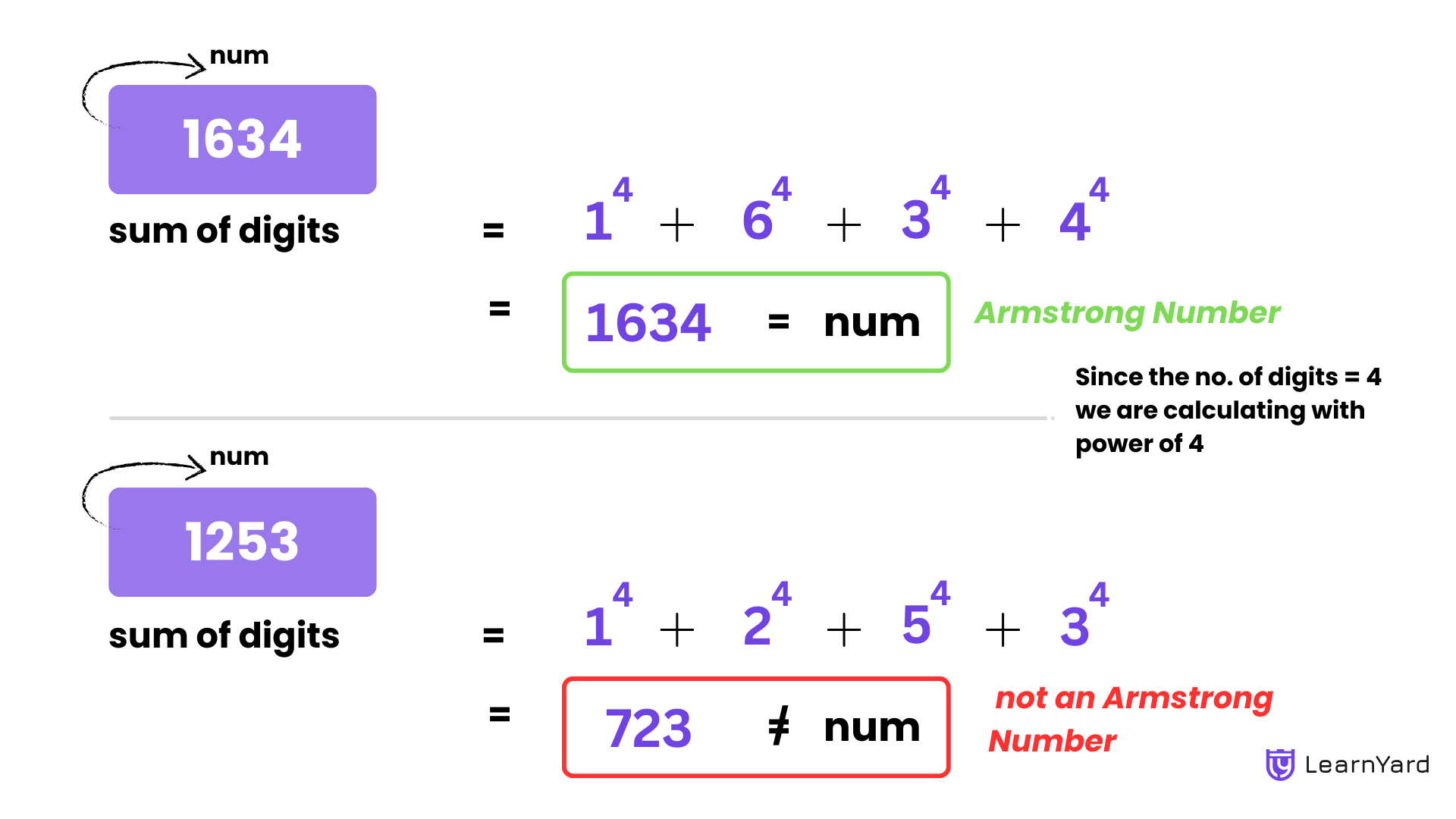
Given a non-negative integer num, check whether the given number is an Armstrong number or not.
An Armstrong number is a number that is equal to the sum of its digits each raised to the power of the number of digits in the number.
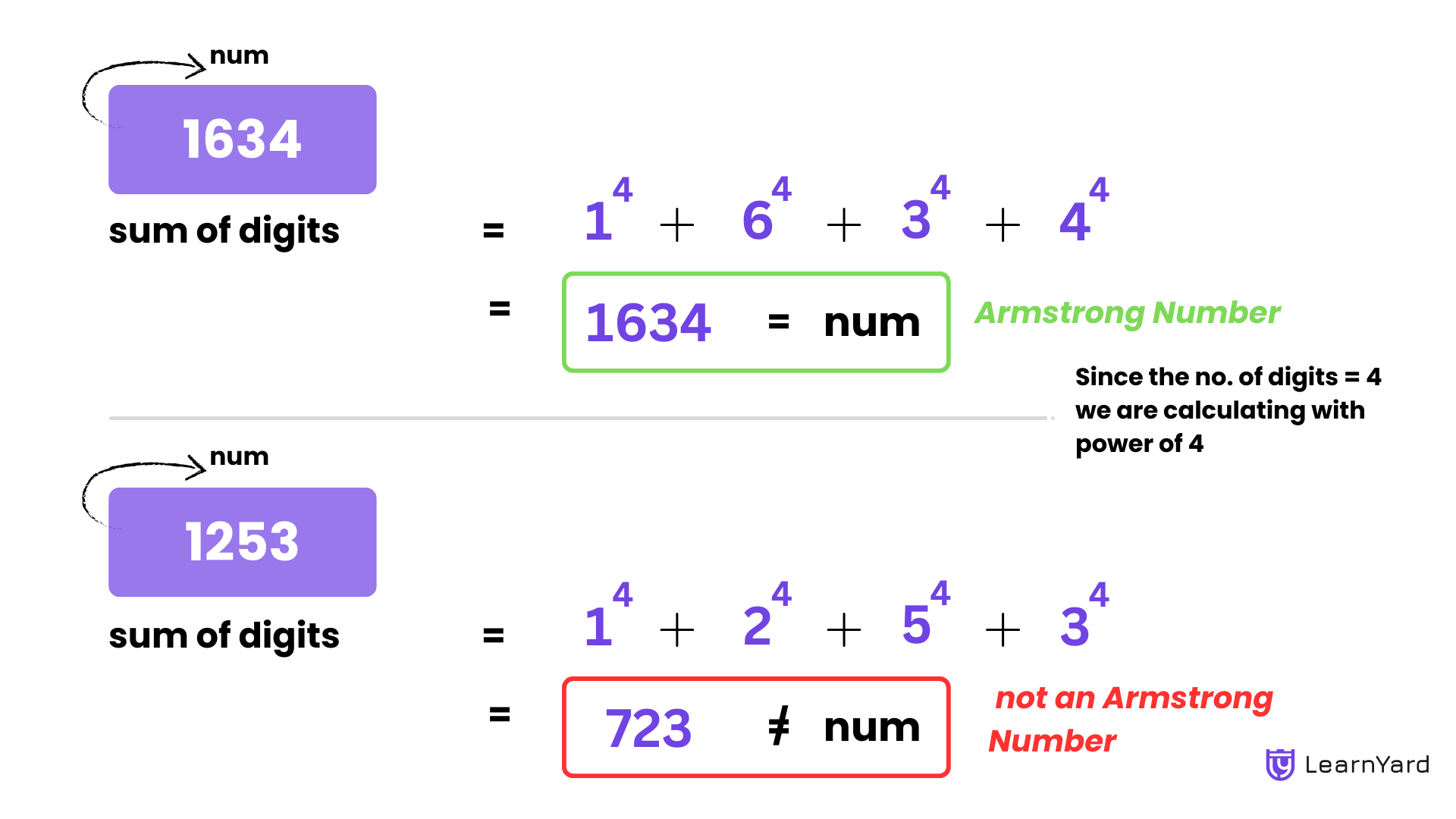
Examples :
Input: 1634
Output: true
Explanation: Yes, 1^4 + 6^4 + 3^4 + 4^4 = 1634.
Input: 1253
Output: false
Explanation: As 1^4 + 2^4 + 5^4 + 3^4 is not equal to 1253, it is not an Armstrong number.
From the definition of the Armstrong number, we know that the Armstrong number is a number that is equal to the sum of its digits each raised to the power of the number of digits(let's say k) in the number.
Let a number 1634 have k=4 digits in it, so to check whether that number is an Armstrong number or not we have to add all those digits raised to the power k and sum up them. But before doing this operation, we must count the number of digits(k) in the given number. Then proceed with the operations discussed above.
Intuition
The idea here is to first calculate the number of digits present in the number, and then we raise each digit to the power of the total digit count and then compare it with the original number.
Approach
- Count the number of digits → First, determine how many digits (k) the given number has. This is essential because each digit will be raised to this power.
- Extract digits and compute the sum → Iteratively extract the last digit using num % 10, raise it to the power k, and add it to a sum variable. Remove the last digit using num = num / 10.
- Compare the sum with the original number → If the computed sum equals the original number, return true; otherwise, return false.
Dry Run
Input: num = 1634
- Count the number of digits (k):
- num = 1634 has 4 digits, so k = 4.
- Extract each digit and compute the sum:
- Extract last digit: 4, raise it to power 4: 4^4 = 256.
- Extract next digit: 3, raise it to power 4: 3^4 = 81.
- Extract next digit: 6, raise it to power 4: 6^4 = 1296.
- Extract next digit: 1, raise it to power 4: 1^4 = 1.
- Calculate the final sum:
- 256 + 81 + 1296 + 1 = 1634.
- Compare with original number:
- Since 1634 == 1634, it is an Armstrong number
Code Implementation
C++ Code Try on Compiler!
Java Code Try on Compiler!
Python Code Try on Compiler!
Javascript Code Try on Compiler!
Time Complexity : O(logn)
The time complexity arises from the number of digits in the number. The number of times the while loop runs depends on the number of digits in the given number num.
For a given number num in a decimal representation, the number of digits is approximately equal to log10(num). This is because each digit represents a power of 10.
The loop executes the first time to find the number of digits O(logn), and then another time to check for the Armstrong number O(logn).
Therefore, the loop runs 2*log10(num) times, which simplifies to O(logn).
Space Complexity : O(1)
We are using only variables like n, sum, and originalnumber which use constant space.
Since the space required by these variables does not depend on the size of the input number num, the auxiliary space is O(1).
Join Our Course
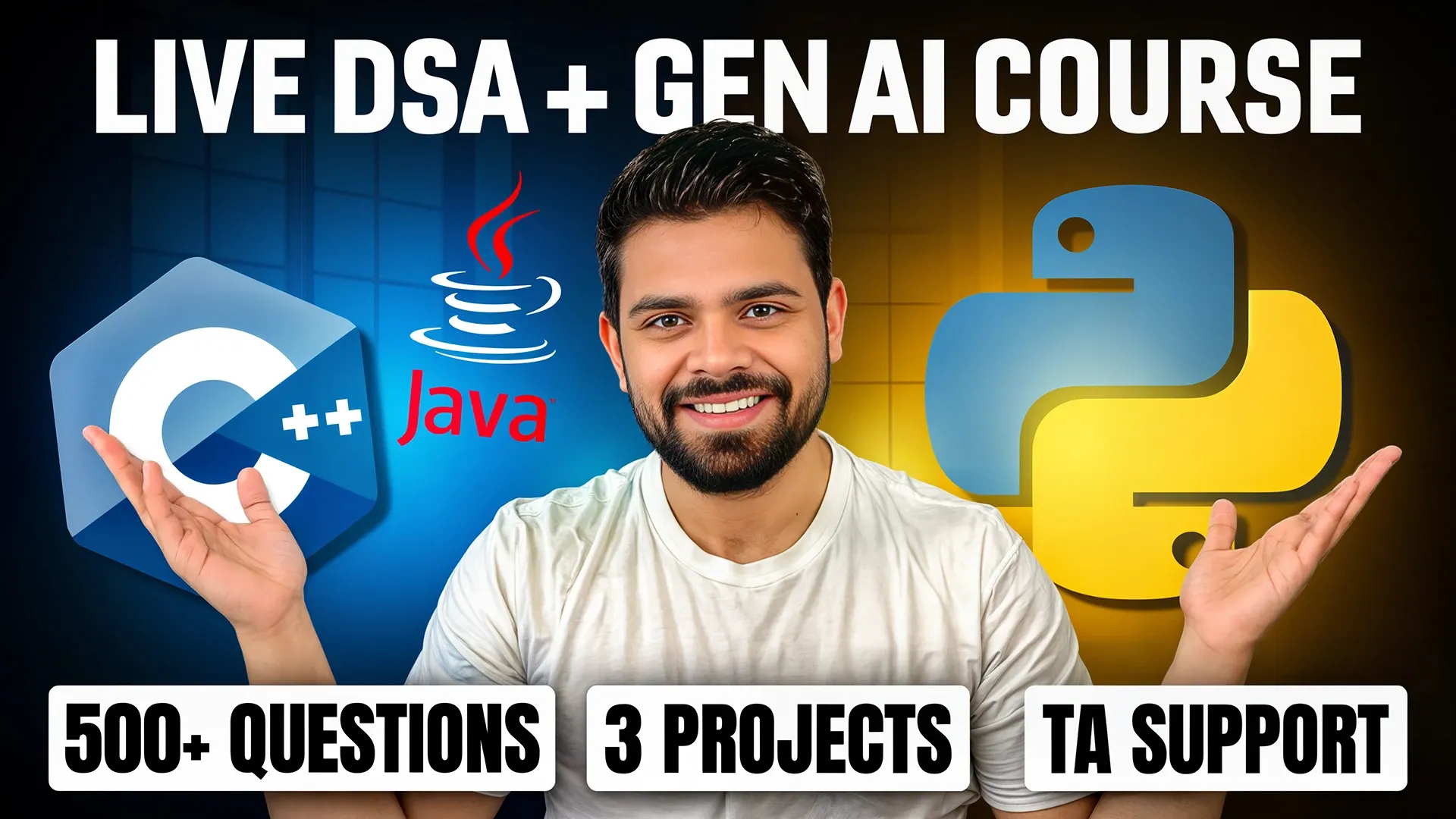
Offer ends in:
Days
Hours
Mins
Secs