Find Maximum Element in Array
In various problem-solving scenarios, we often need to identify key characteristics of a dataset, such as determining the most significant value. For instance, finding the maximum element in an array is a fundamental task that helps us locate the highest point of interest, whether it's the largest number, highest score, or maximum profit. Let’s delve into how this can be accomplished.
Given an array of integers, we need to find the maximum element in the array. The function should print the maximum value found in the array.
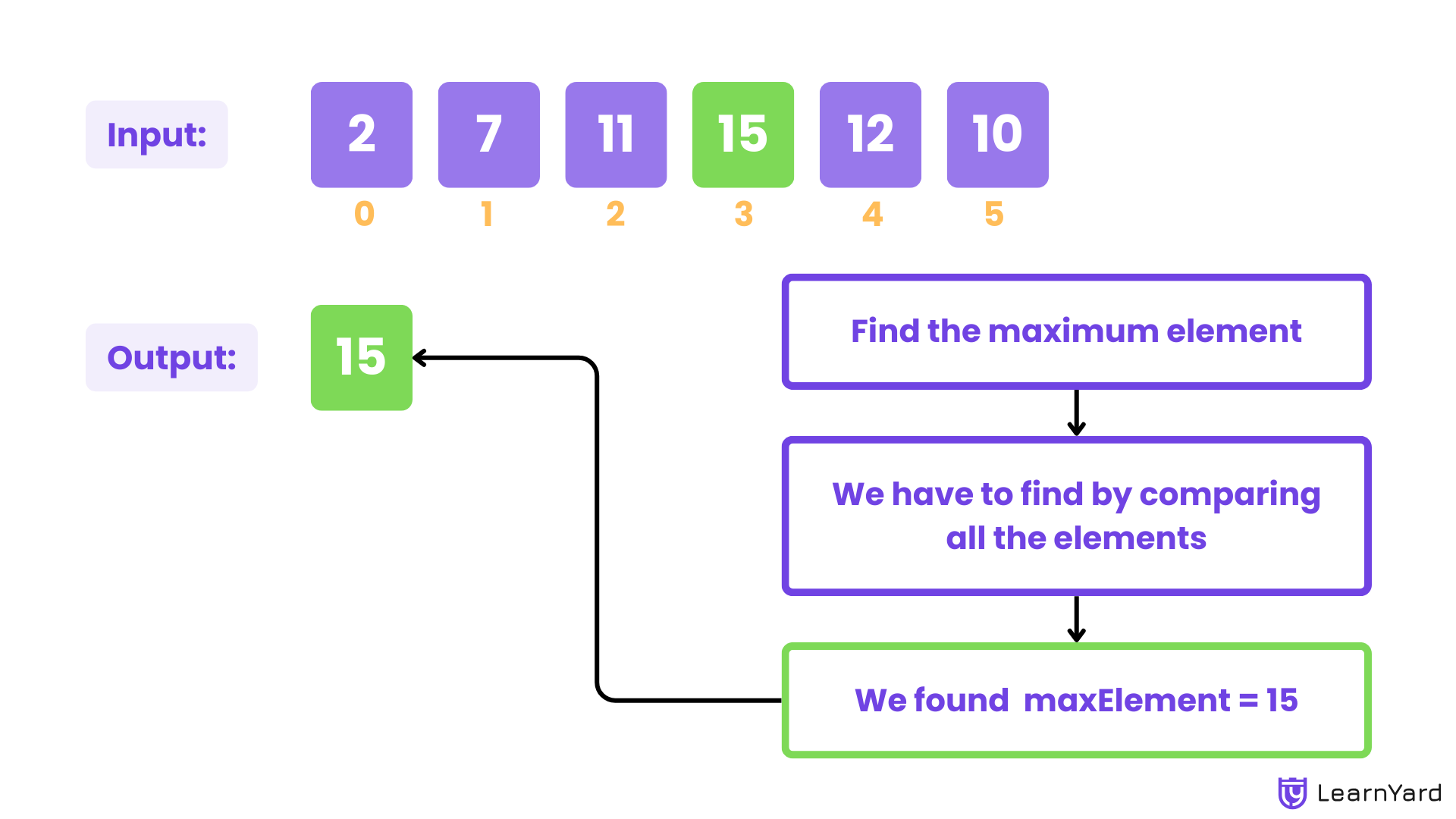
Example
Input: nums = [2, 7, 11, 15, 12, 10]
Output: 15
Explanation: The maximum element in the array is 15.
Input: nums = [3, 4, 1, 5, 2, 5, 1, 10]
Output: 10
Explanation: The maximum element in the array is 10.
Approach
Imagine you're standing in a queue of people, and your goal is to find the tallest person in the line. You start by assuming that the first person you see is the tallest. As you walk down the line, you compare each person with the tallest one you've seen so far. If you find someone taller, you update your "tallest" to that person. By the time you reach the end of the queue, the last person you identified as the tallest is indeed the tallest person in the entire line. This is exactly how the algorithm for finding the maximum element in an array works.
We will use this logic to find the maximum number of a given array too…!
To solve this problem, we need to traverse the entire array(same we walked through the entire queue) and keep track of the maximum element(tallest person) encountered during the traversal. We start by assuming that the first element in the array is the maximum.
Why assume maximum as the first element(nums[0]) in the beginning?
Assuming the first element to be the maximum initially provides a reference point for comparison. As we iterate through the array, we compare each element with this assumed maximum, updating it whenever we find a larger value. This method simplifies finding the maximum by ensuring we always have the largest value encountered so far.
Let's say our array is nums = [2, 7, 11, 15, 12, 10]. Initially, we assume the maximum element is 2 (the first element).
We then iterate through the array, starting from the second element. For each element, we compare it with the current maximum element. If the current element is greater than the current maximum, we update the maximum element to be the current element.
Comparison Process
- First Comparison:
- We compare 7 (the second element) with 2 (the current maximum).
- Since 7 > 2, we update the maximum to 7.
- Second Comparison:
- We then compare 11 (the third element) with 7 (the current maximum).
- Since 11 > 7, we update the maximum to 11.
- Third Comparison:
- Next, we compare 15 (the fourth element) with 11 (the current maximum).
- Since 15 > 11, we update the maximum to 15.
- Fourth Comparison:
- We compare 12 (the fifth element) with 15 (the current maximum).
- Since 12 < 15, we do not update the maximum.
- Fifth Comparison:
- Finally, we compare 10 (the sixth element) with 15 (the current maximum).
- Since 10 < 15, we do not update the maximum.
After completing the traversal of the array, the value of the maximum element will be the largest number in the array. In this case, the maximum value is 15.
Dry Run
Input Details
- Array: nums[] = {2, 7, 11, 15, 12, 10}
- Size of the array (n): 6
Initialization
- The function findMaxElement is called with the input array nums[] and its size n = 6.
- Variable maxElement is initialized to the first element of the array, which is 2.
Iteration Through the Array
First Iteration (i = 1)
- Current element: nums[1] = 7
- Comparison: Is nums[1] > maxElement? (Is 7 > 2?)
- Yes, so maxElement is updated to 7.
Second Iteration (i = 2)
- Current element: nums[2] = 11
- Comparison: Is nums[2] > maxElement? (Is 11 > 7?)
- Yes, so maxElement is updated to 11.
Third Iteration (i = 3)
- Current element: nums[3] = 15
- Comparison: Is nums[3] > maxElement? (Is 15 > 11?)
- Yes, so maxElement is updated to 15.
Fourth Iteration (i = 4)
- Current element: nums[4] = 12
- Comparison: Is nums[4] > maxElement? (Is 12 > 15?)
- No, so maxElement remains 15.
Fifth Iteration (i = 5)
- Current element: nums[5] = 10
- Comparison: Is nums[5] > maxElement? (Is 10 > 15?)
- No, so maxElement remains 15.
Completion
- The loop ends after checking all elements in the array.
- The value of maxElement, which is 15, is printed as the maximum element.
Output
The output is: The maximum element is: 15
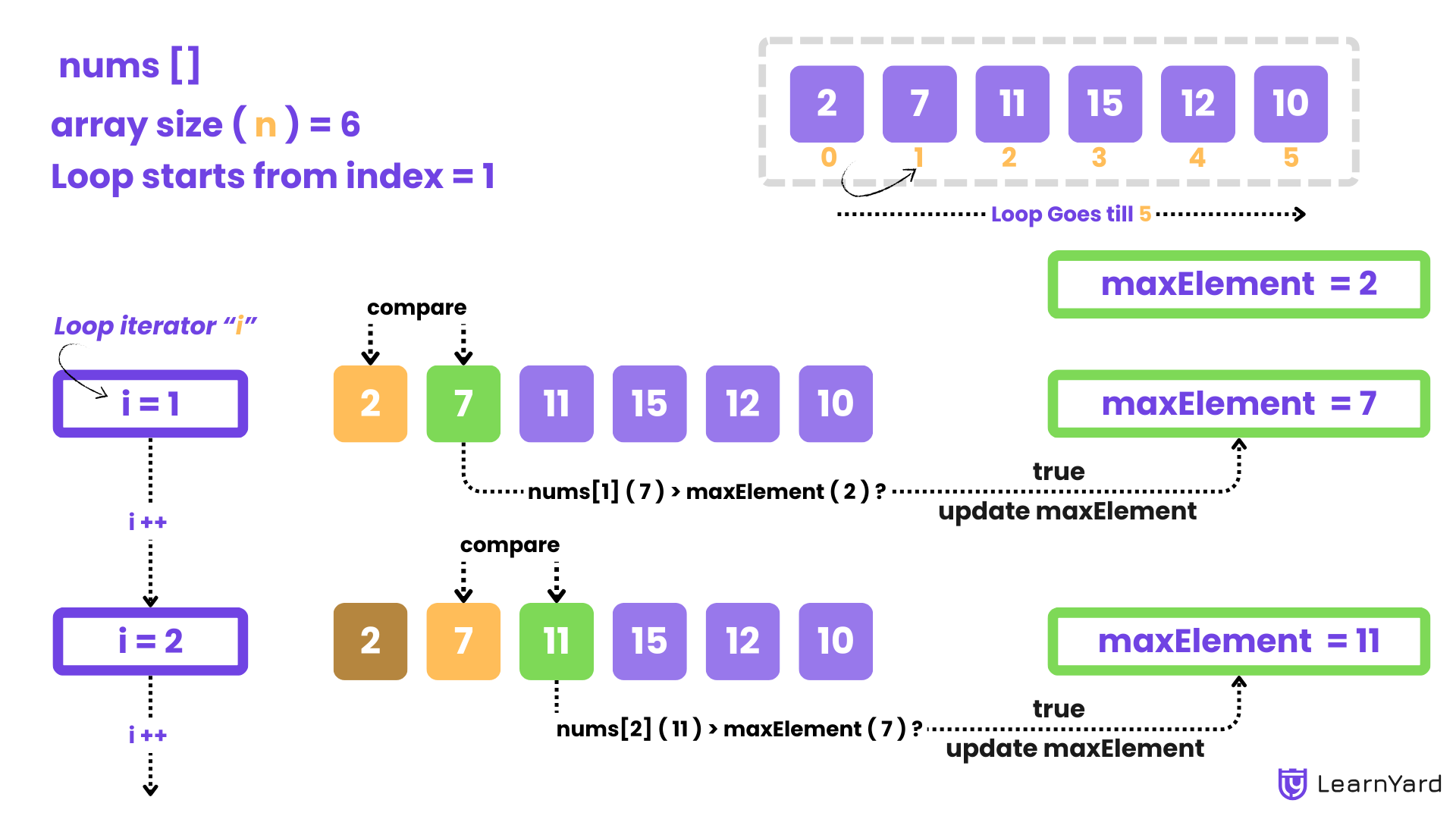
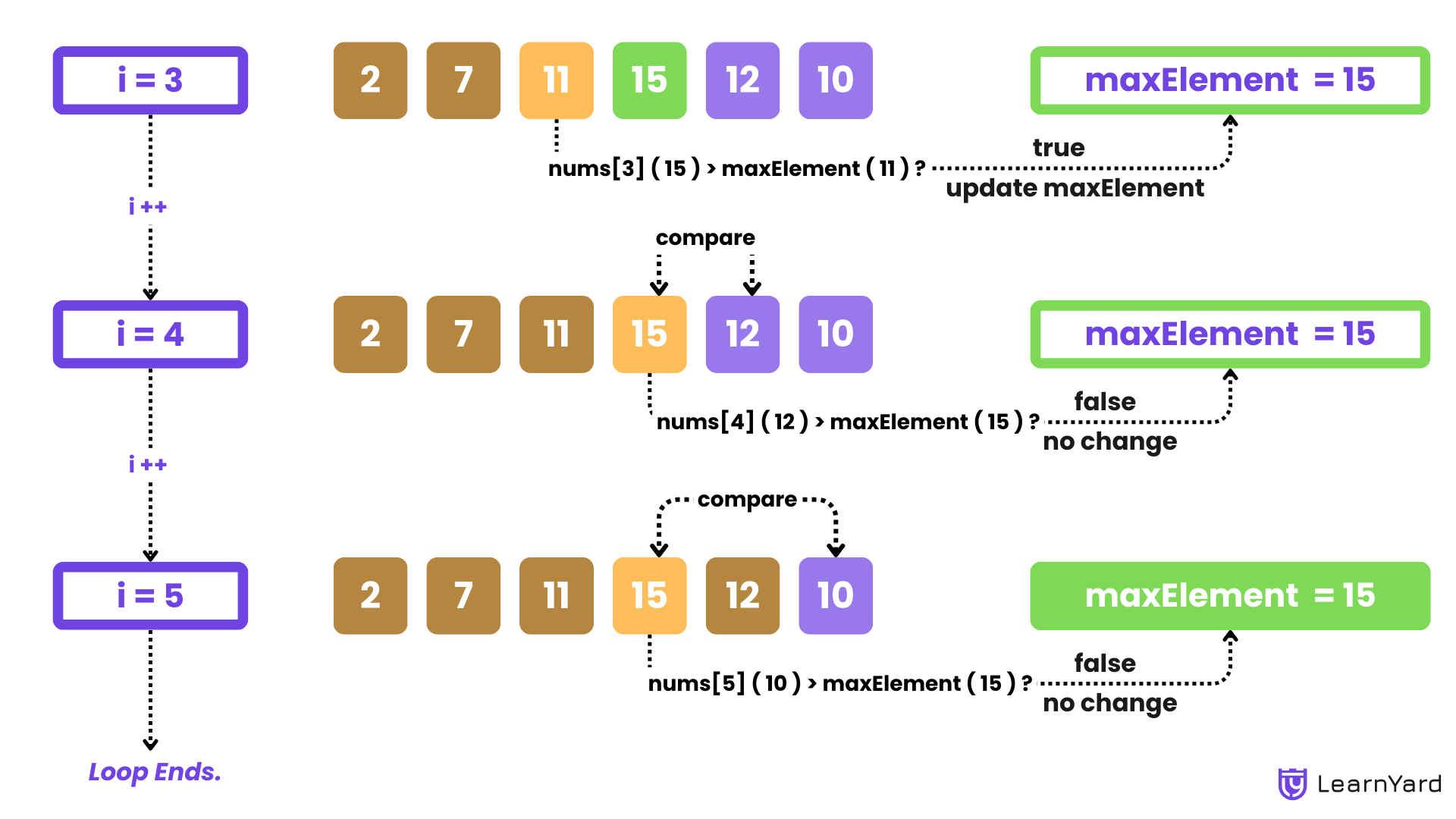
Code for All Languages
C++
#include <iostream>
using namespace std;
// Function to find the maximum element in the array
void findMaxElement(int nums[], int n) {
// Initialize the maximum with the first element of the array
int maxElement = nums[0];
// Iterate through the array starting from the second element
for (int i = 1; i < n; ++i) {
// Compare the current element with the maximum element
if (nums[i] > maxElement) {
// Update the maximum element if the current element is larger
maxElement = nums[i];
}
}
// Print the maximum element found in the array
cout << maxElement << endl;
}
Java
import java.util.Scanner;
public class LearnYard {
// Function to find the maximum element in the array
public static void findMaxElement(int[] nums, int n) {
// Initialize the maximum with the first element of the array
int maxElement = nums[0];
// Iterate through the array starting from the second element
for (int i = 1; i < n; i++) {
// Compare the current element with the maximum element
if (nums[i] > maxElement) {
// Update the maximum element if the current element is larger
maxElement = nums[i];
}
}
// Print the maximum element found in the array
System.out.println(maxElement);
}
}
Python
# Function to find the maximum element in the array
def find_max_element(nums, n):
# Initialize the maximum with the first element of the array
max_element = nums[0]
# Iterate through the array starting from the second element
for i in range(1, n):
# Compare the current element with the maximum element
if nums[i] > max_element:
# Update the maximum element if the current element is larger
max_element = nums[i]
# Print the maximum element found in the array
print(max_element)
Javascript
// Function to find the maximum element in the array
function findMaxElement(nums, n) {
// Initialize the maximum with the first element of the array
let maxElement = nums[0];
// Iterate through the array starting from the second element
for (let i = 1; i < n; i++) {
// Compare the current element with the maximum element
if (nums[i] > maxElement) {
// Update the maximum element if the current element is larger
maxElement = nums[i];
}
}
// Print the maximum element found in the array
console.log(maxElement);
}
Time Complexity
The time complexity of this solution is linear, O(n), because we need to traverse all n elements in the array exactly once to determine the maximum.
Space Complexity
The total space complexity is O(n) because the input array takes up O(n) space.
The auxiliary space complexity is O(1) since we only need a single variable to keep track of the maximum element, which does not depend on the size of the array.