Find Number of Times Target Appear in Array
When working with arrays, a common task is to count how many times a particular element appears in the array. This is often needed in scenarios where we want to analyze the frequency of specific values or simply check the occurrence of a target element. Let’s explore how to achieve this by writing a program that takes an array and a target number as input and counts the number of times the target number appears in the array.
Write a program that takes an array and a target number as input and counts the number of times the target number appears in the array.
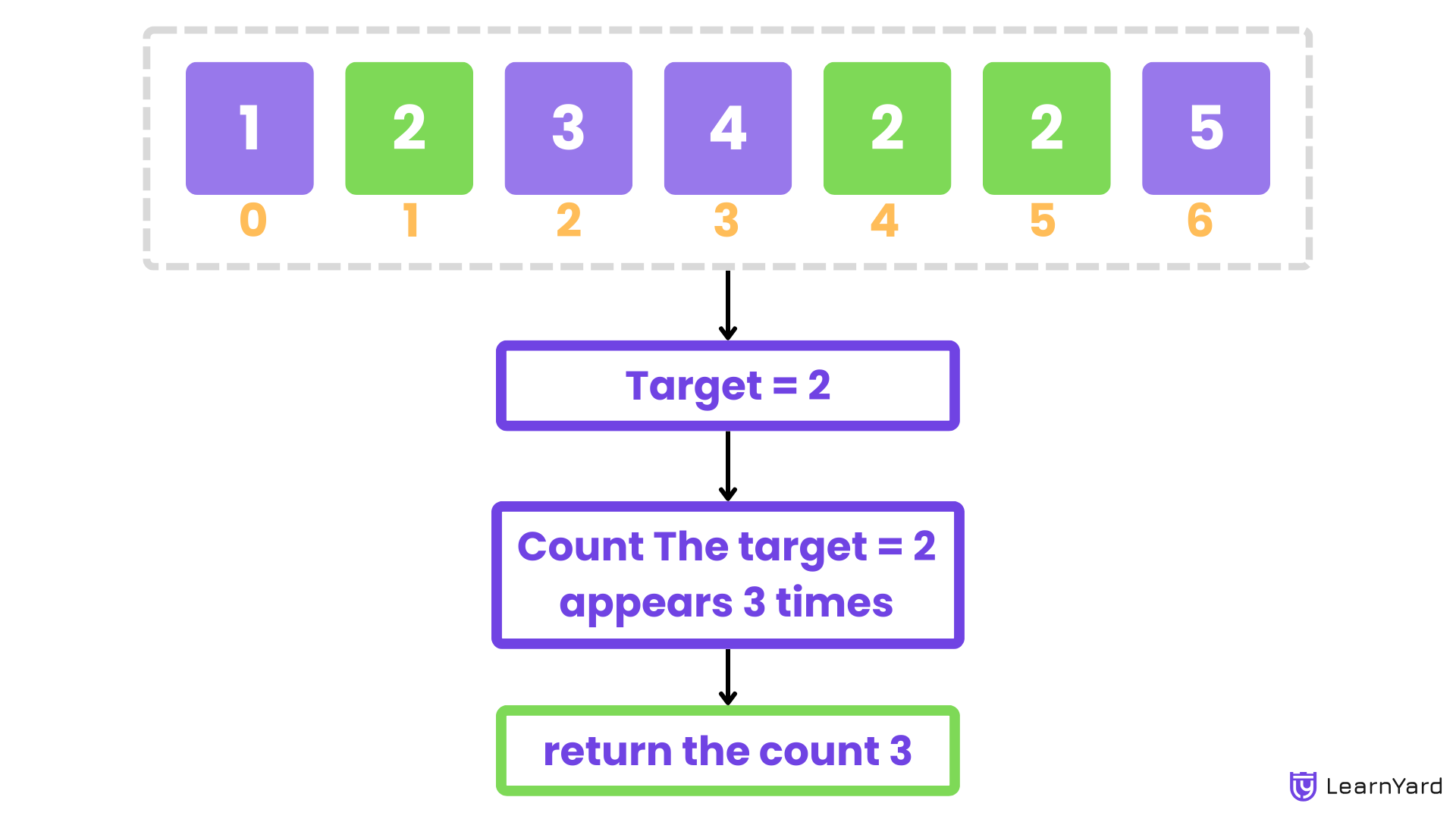
Example
Input: nums = [1, 2, 3, 4, 2, 2, 5], target = 2
Output: Count: 3
Explanation: The number 2 appears 3 times in the array [1, 2, 3, 4, 2, 2, 5].
Input: nums = [5, 6, 7, 8, 5, 9], target = 5
Output: Count: 2
Explanation: The number 5 appears 2 times in the array [5, 6, 7, 8, 5, 9].
Approach
In this problem, our task is to count how many times the target number appears in an array. So first we create a variable “count” and set it to 0 this is our counter. We need a counter to keep track of how many times the target number appears in the array. Without a counter, we would not be able to record the events as we traverse the array. Now use a loop to iterate over each element in the array. For each element, we need to check whether the element corresponds to the target number. If so, increment the count by 1. After the loop checks all the elements, the count variable contains the number of times the target number appeared in the array. Output or return this value.
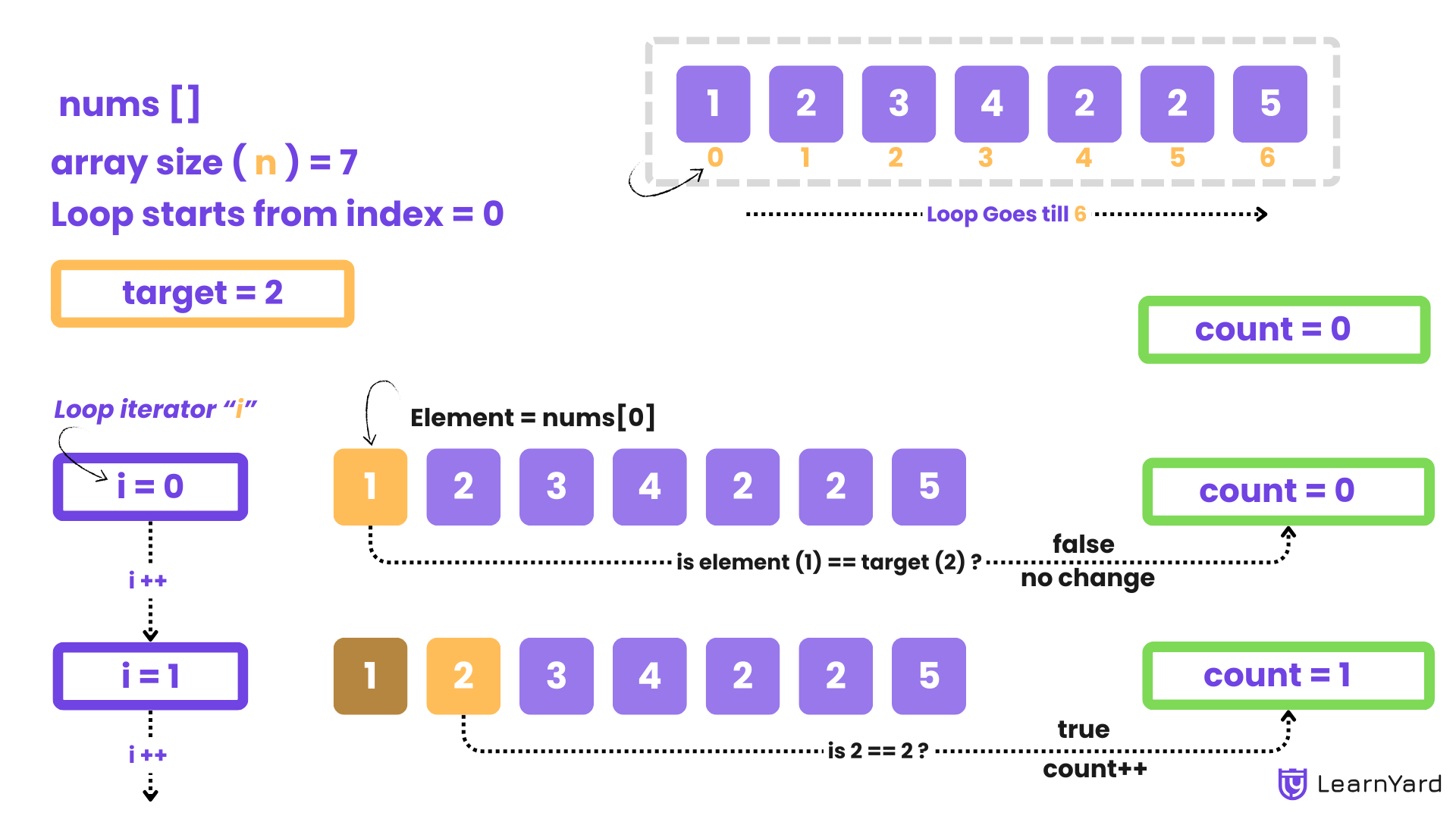
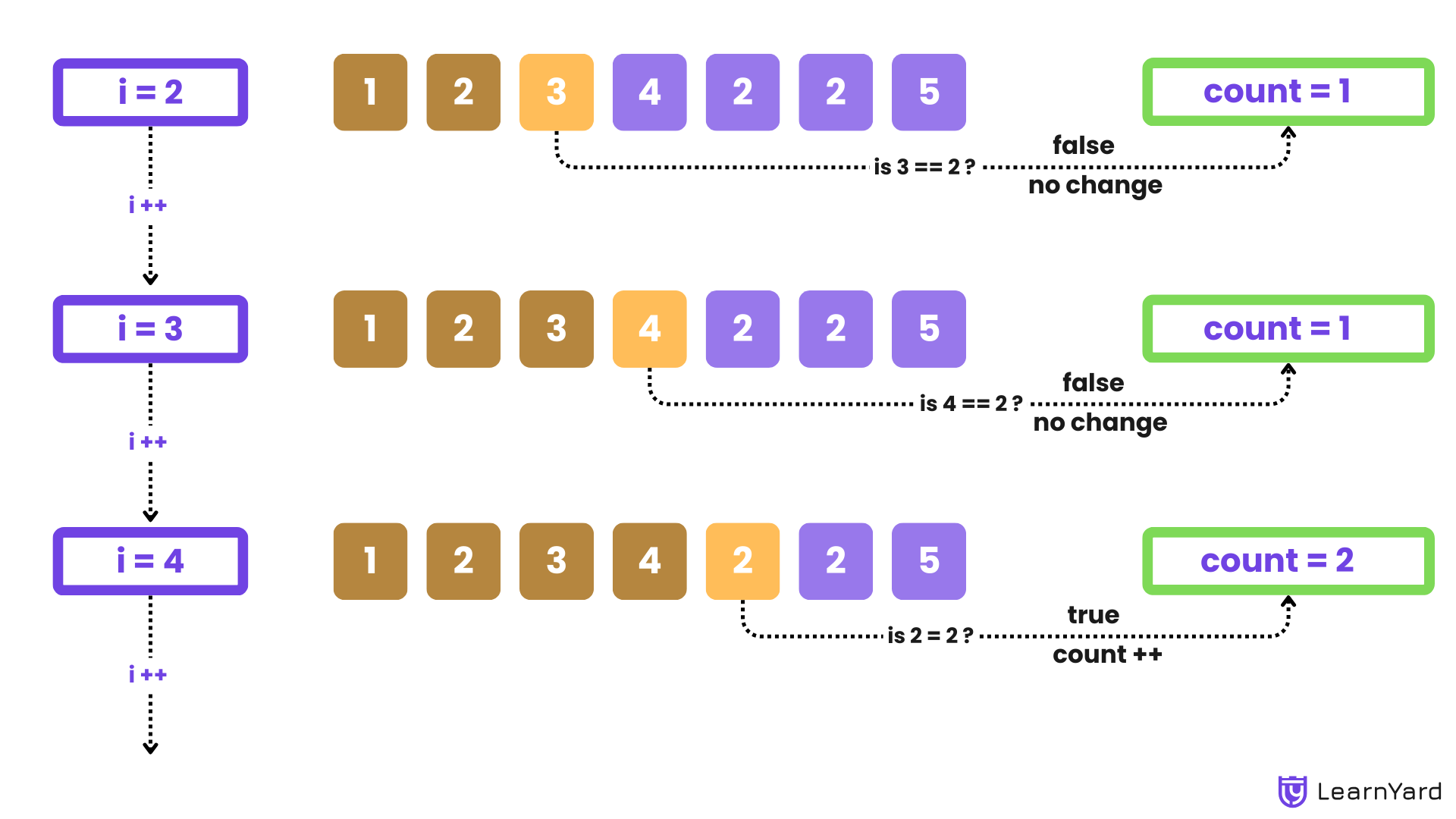
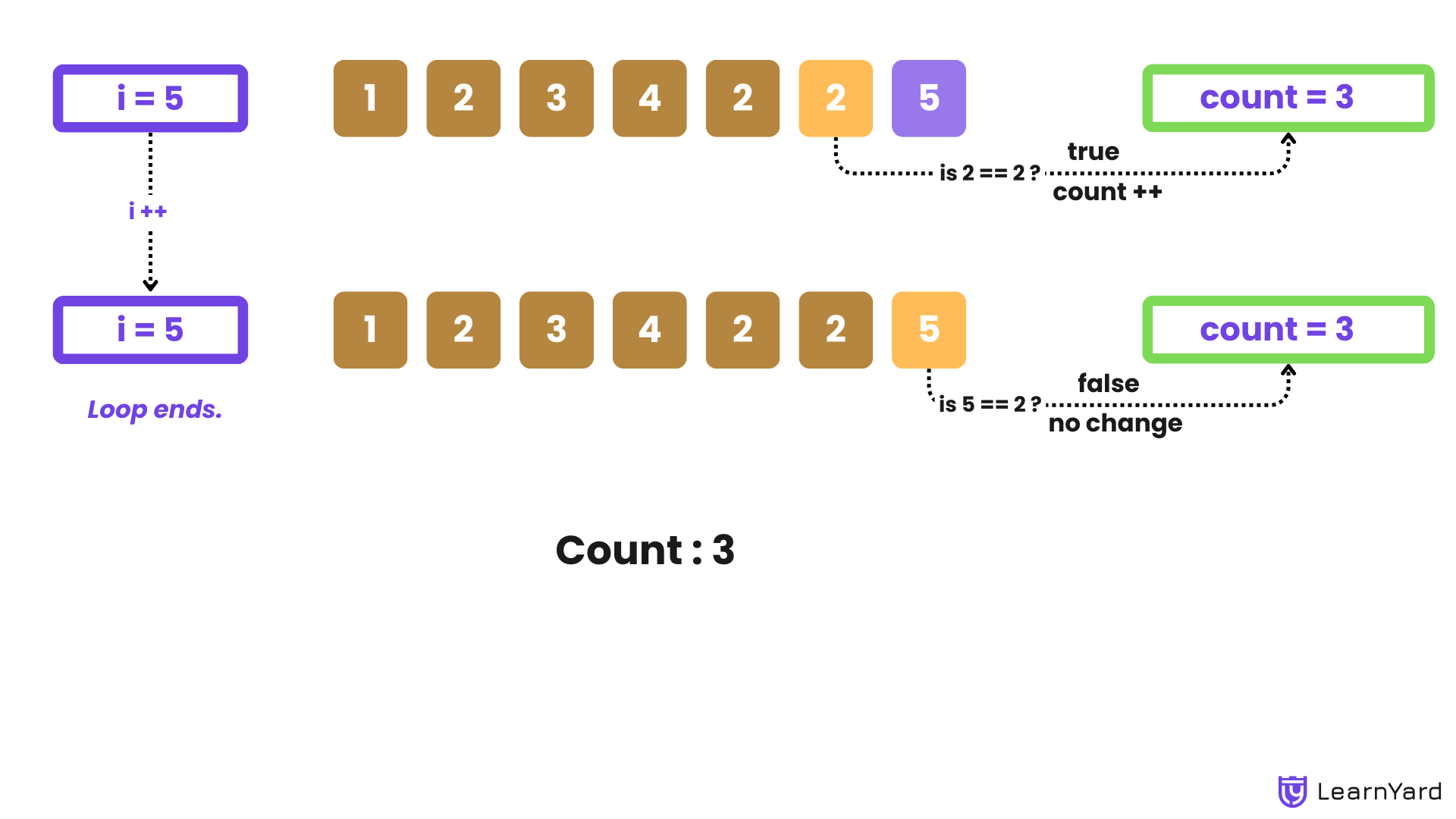
Code for All Languages
C++
#include <iostream>
using namespace std;
// Function to count occurrences of the target number in the array
int countOccurrences(int nums[], int size, int target) {
// Initialize a counter to keep track of occurrences
int count = 0;
// Loop through the array to count occurrences of the target number
for (int index = 0; index < size; ++index) {
// If the current element matches the target, increment the count
if (nums[index] == target) {
++count;
}
}
// Return the final count
return count;
}
int main() {
// Read the size of the array
int size;
cin >> size;
// Declare the array
int nums[size];
// Read array elements
for (int i = 0; i < size; ++i) {
cin >> nums[i];
}
// Read the target value
int target;
cin >> target;
// Call the function and output the result
cout << countOccurrences(nums, size, target);
return 0;
}
Java
import java.util.Scanner;
public class CountOccurrences {
// Function to count occurrences of the target number in the array
static int countOccurrences(int[] nums, int target) {
// Initialize a counter to keep track of occurrences
int count = 0;
// Loop through the array to count occurrences of the target number
for (int num : nums) {
// If the current element matches the target, increment the count
if (num == target) {
count++;
}
}
// Return the final count
return count;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// Read the size of the array
int size = sc.nextInt();
// Declare the array
int[] nums = new int[size];
// Read array elements
for (int i = 0; i < size; i++) {
nums[i] = sc.nextInt();
}
// Read the target value
int target = sc.nextInt();
// Call the function and print the result
System.out.println(countOccurrences(nums, target));
}
}
Python
# Function to count occurrences of the target number in the array
def count_occurrences(nums, target):
# Initialize a counter to keep track of occurrences
count = 0
# Loop through the array to count occurrences of the target number
for num in nums:
# If the current element matches the target, increment the count
if num == target:
count += 1
# Return the final count
return count
# Read the size of the array
size = int(input())
# Read the array elements
nums = list(map(int, input().split()))
# Read the target value
target = int(input())
# Call the function and print the result
print(count_occurrences(nums, target))
Javascript
// Function to count occurrences of the target number in the array
function countOccurrences(nums, target) {
// Initialize a counter to keep track of occurrences
let count = 0;
// Loop through the array to count occurrences of the target number
for (let num of nums) {
// If the current element matches the target, increment the count
if (num === target) {
count++;
}
}
// Return the final count
return count;
}
// Read input data
const size = parseInt(prompt());
const nums = Array.from({ length: size }, () => parseInt(prompt()));
const target = parseInt(prompt());
// Call the function and log the result
console.log(countOccurrences(nums, target));
Time Complexity: O(n)
The loop iterates through each element in the array exactly once, checking if it matches the target number. Since this is a linear scan, the time complexity is O(n), where n is the number of elements in the array.
Space Complexity: O(1)
Auxiliary Space Complexity: This refers to any extra space used by the algorithm that is independent of the input space and output space. In this case, we only use a constant amount of extra space, specifically for the variables. These variables do not depend on the size of the array and therefore take up constant space. so the auxiliary space complexity is O(1).
Total Space Complexity: This includes the space required for the input, output and extra space used by the algorithm as well. The input array nums[] is of size n, So the space required for input space is O(n). No output space is used. Also, the algorithm takes only constant extra space.
Total Space Complexity = O(n) + O(1) + O(1) = O(n)