Why does the Browser Console Return "undefined"?
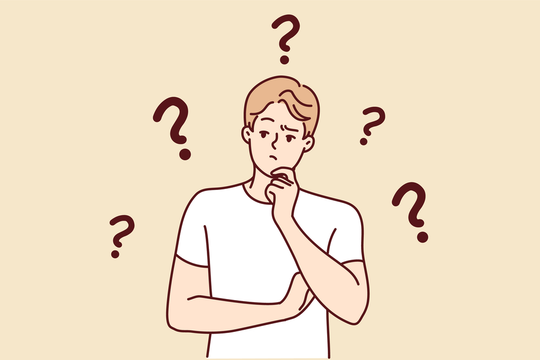
Console is one of the most useful tools to help build Javascript applications and websites. Do you remember what a console is? If you are a continuous reader of this series, we have discussed in detail about what a console is, and how to open it in the browser, if not, let's take a quick diversion to understand it now.
What is a console?
A browser console is a tool that provides information about a browser's activity, including errors, warnings, and requests. It's similar to a command-line interface (usually referred to as a CLI ) but for the entire browser instead of just a single tab. You can use the browser console to debug web pages, view logged messages, and run JavaScript.
In this article though, we are not going to explore the console again, for that as we discussed, we already have an article waiting for you to read! But we are going to discuss an interesting topic that very few people have explained in detail on the Internet! That is?
First, let's understand the topic!
Have you run any code of Javascript on the console before? If yes, that is great if not let's quickly just declare a simple variable in the console.
So, in the console of the browser, I declared a new variable called as name
with a value as "Shrikanth", but the moment I hit enter to run the code, undefined
is printed!

But wait, clearly, we have given the value of the variable to be Shrikanth
, right? Then why does undefined get printed? Did we do something wrong? Did we not declare the variable as it is intended? Is using const
the wrong choice? What is the actual reason? Yep, this is the question we are going to be answering in this article! So buckle up for an exciting topic! ( You are going to love this! )
But wait, one last thing to remember before we proceed, remember undefined
?
Undefined is the value of a variable once it is declared, notnull
, but is called undefined
. It is pretty much what it means, it means that it is declared but is not defined yet. Like a newborn baby, it exists, but does not have a name yet!
If you are not familiar with undefined, I would highly suggest reading the article on Datatypes to learn about undefined where I have explained everything you will need in detail.
Let's now understand REPL to understand how browser console works, this should help us understand why we see undefined
!
REPL? A new IPL team? No, Read-Eval-Print Loop (REPL) methodology is a way in which the code is executed in the browser console. It is not exactly REPL though, but shares similar properties of REPL.
This is different from how we usually run our code, the normal ( if I may put it that way ) way of coding would be to create a file, write the code, drink a cup of coffee, add some more code, and then save the file, and then compile if necessary and then run the code right? But that is not how REPL works, let's understand what the terms mean in REPL.
Read: The code that is written in the console is read, if the syntax is correct and is valid Javascript, the code passes the Read phase.
Eval: This is where the code given by read is evaluated, like the expressions are evaluated and the system understands what the code is trying to perform and the results are generated.
Print: Someone has to print the output so that we as developers can get back the result from the browser right? We need to be able to see the results of our code/ expressions, hence whatever was generated in the Eval phase is printed in this step.
Loop: So, once the print phase is also done, we need to go back to the Read phase and be ready to consume more data - rinse, repeat! That is what the loop helps with.
Now let's understand what is up with undefined
.
Understanding the reason behind Undefined
( Finally! )
Now we know that the code that we enter and hit Enter
takes 3 main steps to get executed, Read, Evaluate and Print, when the print step is reached it needs to decide what to print, let's understand a few scenarios that might help us understand why undefined
was printed!
console.log()
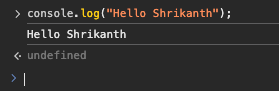
In the above example, we added a console.log()
statement, this is a statement to simply print anything on the screen, this is equivalent to the print statements in other languages.
It did print "Hello Shrikanth" but why did it print undefined after? weird must be a bug right? Let's just let the builders of Google Chrome know?
Not really! In Javascript, every function returns a value even if there is no explicit return statement. What does it return then? Yes, it returns undefined
, hence when we do print the value of "Hello Shrikanth", it first prints a value, but then right after from the console where the console.log()
is called gets back undefined and it gets printed from the REPL print step.
function returns
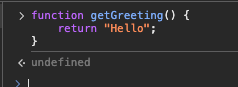
In here we have created a function:
- The function is named
getGreeting
- The function returns a value called "Hello"
But we for some God-forsaken reason still get undefined
?
Let's understand how the code runs here, the function
is created, rather in technical terms, defined, but it is not executed/called yet. Hence at this time the print step has nothing to print and hence like before we print undefined
. But now let's call the function.
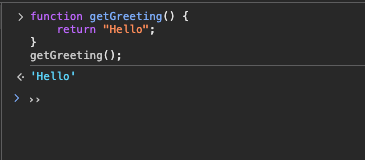
Here we are explicitly running the function too, hence there is a value that was indeed returned, hence we now print Hello
, makes sense?
Expressions
Simple-simple, when an expression is written, a value is generated and hence there is a value to be printed when we reach the REPL - print step, hence we do not see undefined when running the expressions.
Let's understand with a few examples.
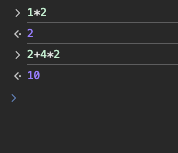
That was easy, right?
Variables
Now let us go back to the first example, why did const name = "Shrikanth"
return undefined
? It surely had the value of "Shrikanth" there!
Let's visualize this step by step on how the code runs.
In JavaScript, when you declare a variable using const
, let
, or var
, the statement itself does not return a value. Instead, it returns undefined
. The console shows this undefined
to indicate that the execution of that statement did not produce a value.
The below steps are performed:
- Code Execution: When you run
const name = "Shrikanth";
, the JavaScript engine declares the variablename
and assigns it the value"Shrikanth"
. - Result of the Statement: The statement itself doesn't return a value. JavaScript functions or expressions can return values, but a variable declaration like this does not.
- Console Output: The console automatically prints the result of the statement you executed. Since variable declarations return
undefined
, that's what you see in the console.
I hope you enjoyed reading this topic, now with the understanding of how console runs Javascript, time to move on to the next topic. I have a ton of articles written just for you about everything on the front end, what are you waiting for, bookmark this for later and keep learning!