Objects and "this"
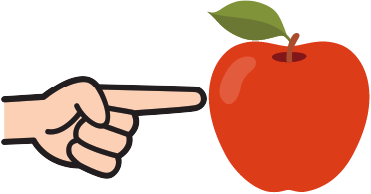
Objects are used to represent entities in the real world. When we need to create entities such as an animal object
let animal = {
name: "Rabbit",
legs: 4
};
Here the object represents the entity "Rabbit" which is an animal. And the properties of the object represent the properties of that entity, here a rabbit. Hence the property name
is the name of the animal, which here is the "Rabbit" and as a Rabbit has 4 legs, the property legs
represents the same.
In real life the object or entity can have actions, that is a rabbit can eat, sleep, etc. A person object if we had one would have a name, age, speak, etc. right?
The actions in the object are called methods. A method is nothing but a function within the object.
Let's understand this with the help of an example:
let animal = {
name: "Rabbit",
legs: 4,
};
animal.eat = function() {
alert("I eat!");
}
Here, now the rabbit can eat.
We can call the method inside an object like
let animal = {
name: "Rabbit",
legs: 4,
};
animal.eat = function() {
alert("I eat!");
}
console.log(animal.eat()); // I eat
The above code would execute the method eat
inside of the object animal
.
Use have used a function expression here and assigned it to the property "eat" inside of the object "animal" and then we called the method.
We could also pre-define the method and then use it inside the object
let animal = {
name: "Rabbit",
legs: 4,
};
function() eat {
alert("I eat!");
}
animal.eat = eat;
console.log(animal.eat()); // I eat
This does the exact thing as before.
Here we created a method called as eat
. We then assigned it to a property called eat
again inside of the object animal and then called it using the property.
Intro to Object-Oriented Programming ( OOP )
Let's take a quick diversion to understand what Object-oriented programming is.
The style of writing code in which we use objects to represent entities is called Object Oriented programming, also called OOPs/ OOP - abbreviating to Object Oriented Programming System or simply Object Oriented Programming.
OOP is a complete concept of its own, it has a science of its own if I may put it that way and we will cover it in detail in their own articles.
But know that, it's about choosing the right entities, organizing them, managing the interactions between them, and much more. There is a lot more to cover about this, but I will take up the topic in detail in multiple articles and you will find them under the Javascript section in the LearnYard read section. ( The same as the current article ).
Moving on!
Short Hands
We learned two ways in which we can add new methods to the object entities. One is using the function expression way and the other is creating a function and then assigning it to a property inside of the object.
But since we know it is redundant to add the function expressions using the function keyword, we can just add new properties using the shorthand.
let animal = {
name: "Rabbit",
legs: 4,
eat {
alert("I eat!");
}
};
console.log(animal.eat()); // I eat
Here as we can see, we added the eat
method using the shorthand. Got it?
The shorthand way of adding methods inside the object is the most popular way of adding methods inside objects.
Assignment: Create an object called user and add the properties called "name" inside it and then add a method called "greet" to say "Hi" using an alert.
Solution:
let user = {
name: "Shrikanth",
};
user.greet = function() { alert("Hi!);}
Now, can you try doing the same with short hands?
Try it out yourself before reading the solution below:
Did you try it yourself?
We could do the same as the below:
let user = {
name: "Shrikanth",
greet() {
alert("Hi!");
}
}
Also, we could use an existing function like:
function greet() {
alert("Hi!");
}
let user = {
name: "Shrikanth",
greet: greet
}
"this" and methods
Imagine, you are chatting with a friend on WhatsApp. Say your friend's name is Rahul. when you click on the input box ( the place where you type the message ), it is obvious that the message is for Rahul and not anyone else, right?, it is the context, if I can put it that way!
Say you are in your house, and you tell your mom, "Hey, I am in the kitchen", do you refer to the kitchen of the home you are in or the kitchen of the neighbor's house? or any other house for that matter? No, it is the kitchen of the house you are in. "this" house, right?
Similarly, it is very common to have to access the data of the current object when using methods inside the object. For example:
let user = {
name: "Shrikanth",
greet: function() {
console.log(`Hey ${this.name}`);
}
}
user.greet();
In the code above, when we execute the code user.greet()
, the value of this
is nothing but the object user
. As I mentioned above, when you are in a house, it is the current house, remember?
Here since it is the context of the current object which is user
, this
is the user object.
Now, why do we need this?
You might be wondering, since we have the user object, why the extra hassle, why not just perform user.greet()
and your code would work perfectly fine, with no errors but, it is unreliable.
Let's understand that better
let user = {
name: "Shrikanth",
greet: function() {
console.log(`Hey ${user.name}`);
}
}
user.greet();
Now the above code works fine as I mentioned right? But now lets assign user to another variable
alet user = {
name: "Shrikanth",
greet: function() {
console.log(`Hey ${user.name}`);
}
}
let anotherUser = user;
user.greet();
But what if I make a small change and assign user
to null now?
let user = {
name: "Shrikanth",
greet: function() {
console.log(`Hey ${user.name}`);
}
s}
let anotherUser = user;
user = null;
another.greet();
Now this leads to an error
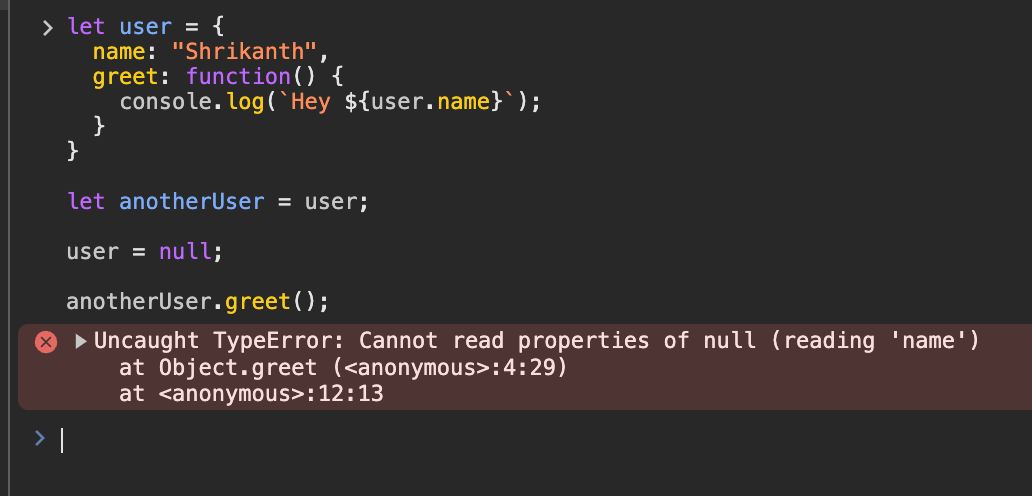
This is why we use this
and don't reference it by the object name. With the use this
instead of user
the code works just fine!
let user = {
name: "Shrikanth",
greet: function() {
console.log(`Hey ${this.name}`);
}
s}
let anotherUser = user;
user = null;
another.greet();
In Javascript, this can be used in normal functions too and the value of this will be evaluated at run time.
function sayName() {
console.log(this.name);
}
This is a valid code!
We can assign the same functions to two different contexts they will work fine too
let user = { name: "Shrikanth" };
let anotherUser = { name: "Vidhathri" };
function sayName() {
console.log(this.name);
}
user.sayName = sayName;
anotherUser.sayName = sayName;
* user.sayName();// Shrikanth
** anotherUser.sayName(); // Vidhathri
In the context of *, the this
is user
and in the context of ** this is anotherUser
for the function sayName
Arrow functions
Arrow functions have no this
, they take the this
of the parent context
let user = {
name: "Shrikanth",
greet: ()=> console.log(this),
};
user.greet();
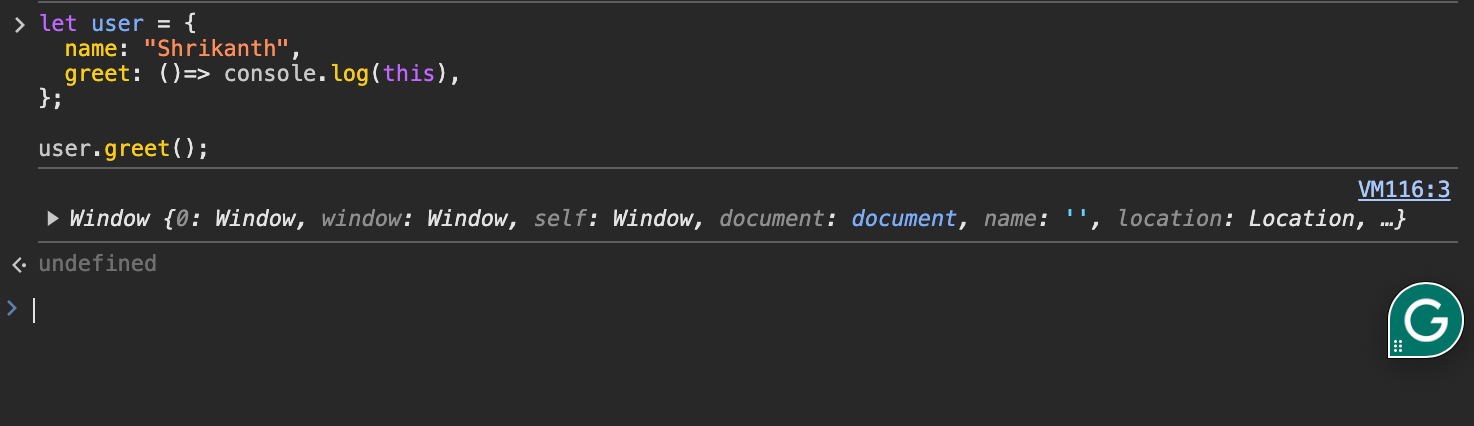
Here if you expect this
to be the user
object, then you are in for a surprise!
We will discuss arrow functions in detail in its own article, but for now, keep the above rule in check!
Happy reading at LearnYard, moving on!