Other Loops in Javascript
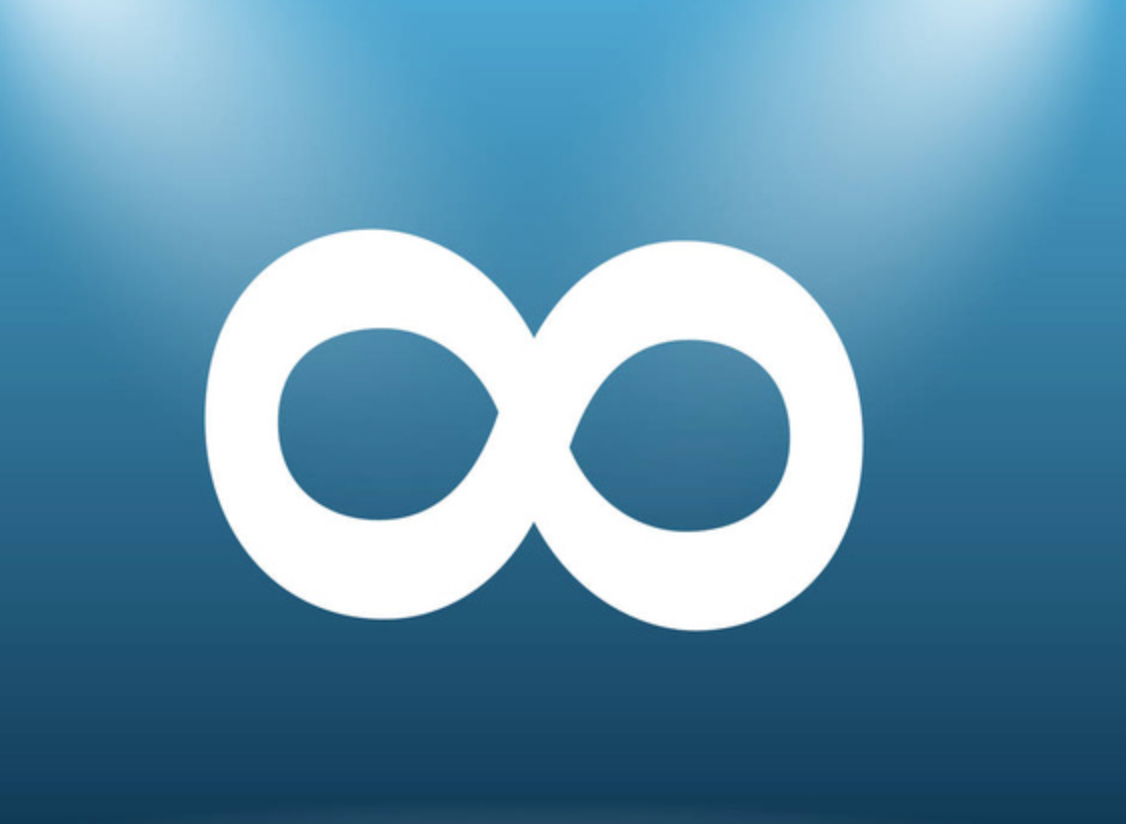
In the world of programming, loops are like the recurring events in our lives—whether it’s your daily cup of chai or the annual Diwali cleaning day. Just as these events repeat themselves in different forms, loops in JavaScript allow you to repeat actions in your code. Today, we’re diving deep into for...in
, for...of
, and nested loops in JavaScript. We’ll explore how these loops work and how you can use them effectively by drawing parallels with our day-to-day activities.
for...in
: Iterating over object properties
Let’s start with the for...in
loop. Imagine you’re managing an Indian wedding, and you’ve got a list of all the tasks that need to be completed, from sending invitations to arranging the catering. Each task has a person responsible for it, much like how each property in a JavaScript object has a key-value pair. The for...in
loop is your go-to tool for iterating over these properties.
Understanding the for...in
Loop Syntax
The for...in
loop is used to iterate over the properties of an object. Here’s the syntax:
for (key in object) {
// Code to execute
}
In this loop:
key
represents the property name (or key) of the object.object
is the object whose properties you want to iterate over.
Example of a Basic for...in
Loop
Let’s bring this to life with our Indian wedding example. Suppose you have an object that lists the key tasks of the wedding, along with the family members responsible for each:
let weddingTasks = {
invitations: "Uncle",
decorations: "Cousin",
catering: "Father",
photography: "Brother",
music: "Friend"
};
for (let task in weddingTasks) {
console.log(`${task}: Handled by ${weddingTasks[task]}`);
}
Note: I know we haven't learned about Objects in detail yet, but hey - you just created your first object above, let's learn more about objects in the Objects section
In this example, the for...in
loop iterates over each task in the weddingTasks
object and prints out who is responsible for it.
Managing Wedding Tasks
Now, let’s expand on this example. Imagine you’re the head of the family, and you need to ensure that each task is progressing smoothly. You decide to check in on each family member responsible for a task:
let weddingTasks = {
invitations: "Uncle",
decorations: "Cousin",
catering: "Father",
photography: "Brother",
music: "Friend"
};
for (let task in weddingTasks) {
console.log(`Checking on ${weddingTasks[task]} for the ${task} task.`);
// Assume each task is progressing well
console.log(`${task} is on track!`);
}
Here, the for...in
loop allows you to iterate over each task, ensuring that everything is proceeding as planned for the big day.
for...of
: Iterating over Iterable objects
While the for...in
loop is great for iterating over object properties, the for...of
loop is your go-to when dealing with iterable objects like arrays, strings, or maps. Imagine you’ve got a list of items to be included in the wedding feast, and you want to iterate through this list to confirm everything is ordered.
Syntax
for (element of iterable) {
// Code to execute
}
In this loop:
element
represents the current element of the iterable.iterable
is the object you want to iterate over (like an array).
Example of a basic for...of
Loop
Let’s stick with our wedding example. Suppose you have an array of items that need to be included in the wedding feast:
let feastItems = ["Paneer Butter Masala", "Biryani", "Naan",
"Gulab Jamun", "Lassi"];
for (let item of feastItems) {
console.log(`Ordering ${item} for the wedding feast.`);
}
In the above example, the for...of
loop iterates over each item in the feastItems
array, ensuring everything is ordered for the wedding feast.
Double-Checking the Feast List
To ensure that no item is missed, you decide to double-check the list by iterating over it again, perhaps even appending a "Checked" next to each item:
let feastItems = ["Paneer Butter Masala", "Biryani", "Naan", "Gulab Jamun", "Lassi"];
for (let item of feastItems) {
console.log(`Rechecking: ${item} - Checked`);
}
Here, the for...of
loop is perfect for iterating over each item in the list, confirming that every dish will make it to the wedding table.
Nested Loops
Now that we’ve covered the basics of for...in
and for...of
, let’s dive into nested loops—loops within loops. Imagine the Indian wedding scenario again.
You’re not only organizing the wedding tasks but also managing multiple sub-tasks for each main task. Nested loops come in handy when dealing with such complex, multi-layered tasks.
Let’s say that each main task of the wedding has several sub-tasks. For example, the decorations
task might involve choosing a theme, selecting flowers, and setting up the venue. Here’s how you could represent this scenario using nested loops:
let weddingTasks = {
invitations: ["Design invites", "Print invites", "Send invites"],
decorations: ["Choose theme", "Select flowers", "Set up venue"],
catering: ["Select menu", "Hire caterer", "Arrange tasting"],
photography: ["Hire photographer", "Choose photo spots"],
music: ["Select playlist", "Book DJ"]
};
for (let task in weddingTasks) {
console.log(`Main Task: ${task}`);
for (let subTask of weddingTasks[task]) {
console.log(` Sub-task: ${subTask}`);
}
}
In the above example:
- The outer
for...in
loop iterates over each main task. - The inner
for...of
loop iterates over the sub-tasks for each main task.
Imagine it’s the night before the wedding, and you’re doing a final check of all the tasks and sub-tasks. You want to ensure that nothing has been overlooked:
let weddingTasks = {
invitations: ["Design invites", "Print invites", "Send invites"],
decorations: ["Choose theme", "Select flowers", "Set up venue"],
catering: ["Select menu", "Hire caterer", "Arrange tasting"],
photography: ["Hire photographer", "Choose photo spots"],
music: ["Select playlist", "Book DJ"]
};
for (let task in weddingTasks) {
console.log(`Final check on: ${task}`);
for (let subTask of weddingTasks[task]) {
console.log(` Sub-task done: ${subTask} - checked`);
}
}
Here, the nested loops allow you to systematically go through each task and sub-task, ensuring everything is in place for the big day.
Comparing for...in
and for...of
: When to use which?
You might wonder when to use for...in
and when to use for...of
. The answer lies in understanding the type of data you’re working with.
- Use
for...in
when you need to iterate over the properties of an object. It’s perfect for scenarios where you want to work with the keys of an object, such as listing all the wedding tasks and who’s responsible for them. - Use
for...of
when you need to iterate over the values of an iterable object, like an array or a string. It’s ideal for scenarios where you’re dealing with lists or collections, such as ordering items for a wedding feast.
Let’s say you have a list of wedding guests, and you want to print out their names along with their seating arrangements. You could use for...of
to iterate over the guest names and for...in
to iterate over the seating arrangements:
let guests = ["Ramesh", "Suresh", "Meena", "Pooja", "Ajay"];
let seatingArrangement = {
Ramesh: "Table 1",
Suresh: "Table 1",
Meena: "Table 2",
Pooja: "Table 2",
Ajay: "Table 3"
};
console.log("Guest List:");
for (let guest of guests) {
console.log(`Guest: ${guest}`);
}
console.log("\nSeating Arrangement:");
for (let guest in seatingArrangement) {
console.log(`${guest} is seated at ${seatingArrangement[guest]}`);
}
In this example:
for...of
is used to iterate over the list of guests.for...in
is used to iterate over the seating arrangements.
Loops are the backbone of programming, just like rituals and traditions are the backbone of an Indian wedding. Whether you’re iterating over wedding tasks, guest lists, or feast items, understanding for...in
, for...of
, and nested loops will make your JavaScript code more efficient and organized.
Did you understand all the concepts well? If yes, send us a feedback, else, let us know how we can help you better, we here at LearnYard are dedicated to help you become the developer you deserve to be!