Conditional Statement in JS: If and Else
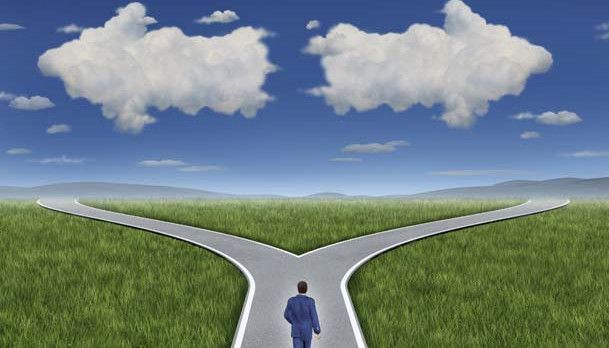
Sometimes in life, you have to make decisions. Do I want pizza or burgers? Should I binge-watch a show or get some sleep? Should I ask her out on a date or should I just let it go? Just like real life, JavaScript also has its decision-making tools, and they’re known as conditional branching. The most common tools in JavaScript for this are the if
statement and the conditional operator ?
, also affectionately called the "question mark" operator.
Let’s break it down.
The if
Statement: Your Code's Decision-Maker
The if
statement is like a fork in the road for your code. You give it a condition to check, and if that condition is true, it’ll execute whatever code you’ve written inside its block. While you are traveling you might see a left and a right and see the board like "Take left for Bangalore" and right for "Mangalore". Here you have to make a decision to move ahead based on what your need is, correct?
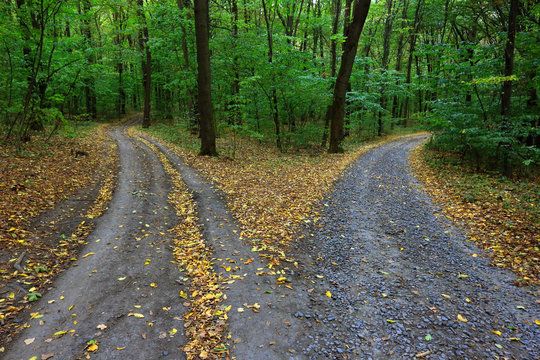
Just like the fork road above.
Here’s a quick example:
let year = prompt('When did India get Independence?', '');
if (year == 1947) {
alert('You are right!');
}
In this case, if the user enters "1947," they’ll get a nice little alert telling them they’re correct. If not, the code inside the if
block just won’t run. It’s that simple.
Pro Tip: Always Use Curly Braces
Even though JavaScript lets you skip the curly braces {}
if your if
block only has one statement, it’s a good idea to use them anyway. It makes your code more readable and helps avoid silly mistakes.
if (year == 1947) {
alert("That's correct!");
alert("You're so smart!");
}
Boolean Conversion: Truthy and Falsy
When JavaScript evaluates the condition in an if
statement, it converts the result into a boolean. This is where the concept of "truthy" and "falsy" values comes into play.
- Falsy values include
0
,""
(empty string),null
,undefined
, andNaN
. These all get converted tofalse
. - Truthy values are everything else. So, basically, if it’s not falsy, it’s truthy.
if (0) { // 0 is falsy, so this block won’t run
// This code won’t execute
}
if (1) { // 1 is truthy, so this block will run
// This code will execute
}
You can also use variables that have already been evaluated to a boolean:
let condition = (year == 1947); // true or false
if (condition) {
// Runs if condition is true
}
The else
Clause: Handling the "Otherwise"
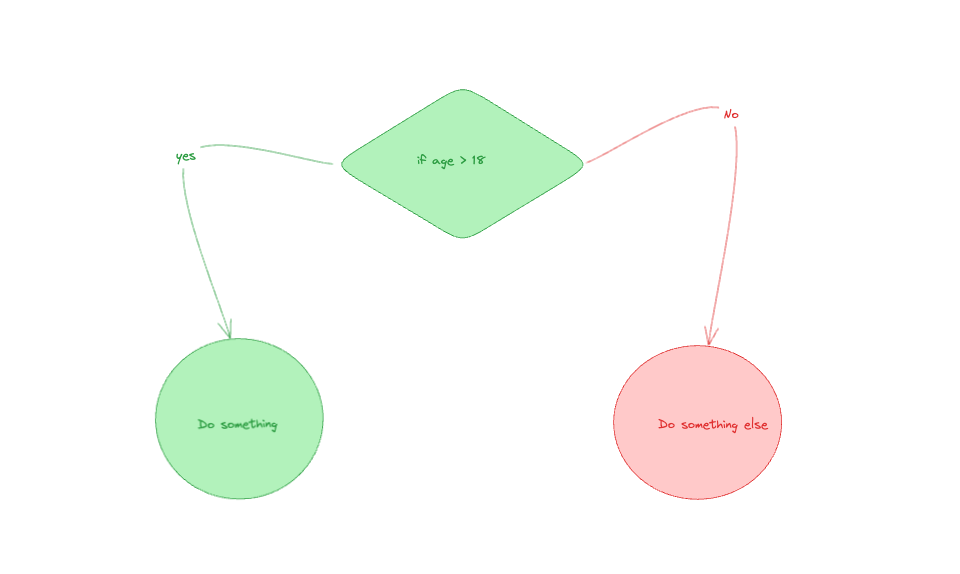
Say I have a milky bar chocolate and a 5-star and I ask my friend - you can choose one, he says, I do not want a milky bar, that automatically means he wants 5 star right?
Let's take another example, say your brother asks you, can you give me 500rs, you check and see that you have 400rs, so it automatically means you cannot give 500rs right?
Just like the else
statement is like the "otherwise", if the if
condition fails, it always runs the else statement regardless if there is an else
statement of course.
Let's get back to our example again shall we?
let year = prompt('When did India get Independence?', '');
if (year == 1947) {
alert('You are right!');
} else {
alert("Not really, try again?");
}
Here if the user enters anything other than 1947, it will not evaluate to true in the if
statement, that means it's false
, that is where it will run the else statement.
Multiple Conditions: else if
Now, what if there are multiple conditions, not just one if
condition?
let year = prompt('When did India get Independence?', '');
if (year < 1947) {
alert('Too early');
} else if(year> 1947) {
alert("Not really, too late);
} else {
alert("Perfect!");
}
Note: Please execute all of the code I provide you in these articles, like a great man once said, you learn the best by writing and teaching, write the code down on your editor, or discuss it with a friend, that is the best way to learn and remember, okay let's move forward!
In this example, the code first checks if the year is less than 1947. If not, it checks if it’s greater than 1947. If neither is true, the final else
block executes.
The Conditional (Ternary) Operator ?
When you need to assign a value based on a condition, the ternary operator ?
is your go-to tool. It’s called "ternary" because it operates on three values: a condition, a value if the condition is true, and a value if it’s false.
Here’s a basic example:
let accessAllowed;
let age = prompt('How old are you?', '');
accessAllowed = (age > 18) ? true : false;
alert(accessAllowed);
This code checks if the user’s age is over 18. If it is, accessAllowed
becomes true
; otherwise, it becomes false
.
Chaining the Ternary Operator
You can also chain multiple ternary operators together to handle more complex scenarios:
let age = prompt('age?', 18);
let message = (age < 3) ? 'Hi, kiddo!' :
(age < 18) ? 'Hello!' :
(age < 100) ? 'Greetings!' :
'Great to meet you!';
alert(message);
This code checks several conditions in sequence, returning a different message for each range of ages.
Non-Traditional Use of ?
Sometimes, developers use the ?
operator as a replacement for if
, but this is generally discouraged because it can make your code harder to read.
let company = prompt('Which company created JavaScript?', '');
(company == 'Netscape') ? alert('Right!') : alert('Wrong.');
While this works, using an if
statement is usually better for clarity:
let company = prompt('Which company created JavaScript?', '');
if (company == 'Netscape') {
alert('Right!');
} else {
alert('Wrong.');
}
In JavaScript, conditional branching is all about making your code react to different conditions. Whether you’re using if
, else
, else if
, or the ternary operator ?
, these tools help you control the flow of your program and make decisions on the fly. Just remember to use the right tool for the job, and always keep readability in mind, that is how you become a great developer!