Introduction Functions in Javascript - Functions 1
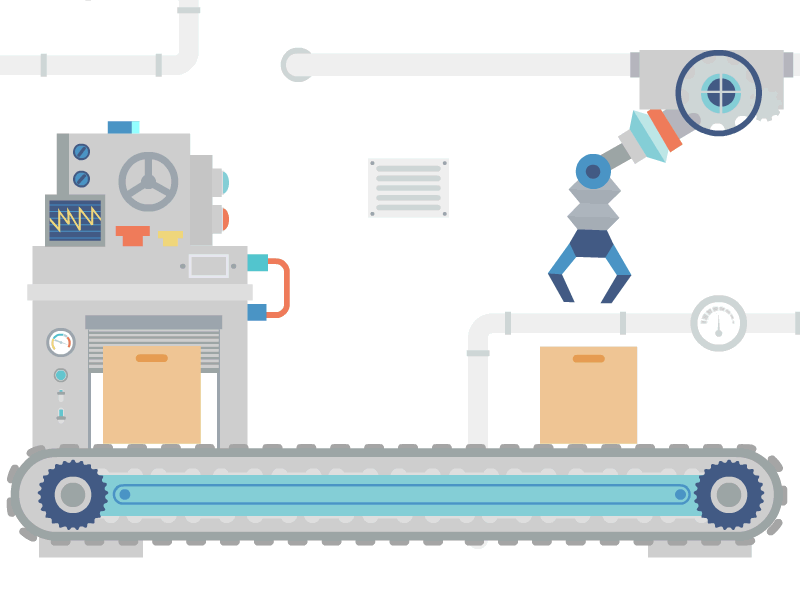
In the world of JavaScript, functions are the main building blocks. They allow you to perform tasks and calculations without repeating code. Functions can be used to encapsulate code for reuse, making your programs more modular and maintainable. Let's dive into the essentials of JavaScript functions, excluding arrow functions for now—we’ll cover those in a separate article.
Function Declaration
To create a function, we use a function declaration. Here’s how it looks:
function showMessage() {
alert('Hello everyone!');
}
The keyword function
is followed by the name of the function, a list of parameters (which can be empty), and the function body enclosed in curly braces. The function can then be called by its name:
showMessage(); // Alerts "Hello everyone!"
showMessage(); // Alerts "Hello everyone!" again
Here’s what’s happening:
- function: This keyword tells JavaScript, "Hey, I’m about to define a function!"
- showMessage: This is the name of your function. You can name it whatever makes sense for the task at hand.
- (): The parentheses are where you’d put any inputs (more on this later).
- {}: The curly braces contain the magic—this is where the actual task happens.
This approach avoids code duplication. If you need to change the message or its format, you only need to update the function.
Let's imagine functions like this - you’re building a robot—let's call it "LearnYardBot." This bot needs to do several tasks: greet you, tell the time, maybe even make a cup of coffee (if only!). Instead of building a whole new bot every time you want it to do something, you’d give it a set of instructions—tasks it can perform whenever you need. That’s what functions are like in JavaScript. They’re your Bot’s instructions, ready to be called into action whenever you need them. Got it?
Avoiding Code Repetition
Think about it. If you had to repeat the code for every single task our bot can do, you’d lose your mind. What if you want to change the greeting message? You’d have to update it in every single place. But with a function, you just change it in one spot:
function greetUser() {
alert('Greetings hooman!');
}
greetUser(); // Alerts "Greetings hooman!"
Just like that, our robot is now a bit more sophisticated.
Local Variables
When our bot performs a task, it might need to remember things—like the name of the person it’s greeting. But you don’t want these details spilling over into other tasks. That’s where local variables come in. They’re like LearnYardBot's private diary, if I may put it that way, only visible while it’s doing that specific task:
function greetUser() {
let userName = 'Shrikanth'; // Local variable
alert('Hello, ' + userName + '!');
}
greetUser(); // Alerts "Hello, Shrikanth!"
If you try to access userName
outside of greetUser
, JavaScript will give you an error. It’s like trying to read someone else’s diary—it’s not allowed (unless it's your siblings', then you have to!)
Outer Variables
Now, what if you need to share some info across tasks? Like a global setting for LearnYardBot—maybe it needs to know what language to use for all its greetings. That’s where outer variables come in. These are like public notes that any function can read or update:
let language = 'English';
function greetUser() {
let message = (language === 'English') ? 'Hello!' : 'Namaskar!';
alert(message);
}
greetUser(); // Alerts "Hello!"
And if you change that outer variable, LearnYardBot will adjust accordingly:
language = 'Hindi';
greetUser(); // Alerts "Namaskar!"
Parameters: Customizing LearnYardBot's Actions
Remember those parentheses in the function declaration? That’s where parameters come in. They’re like inputs you give to Bot to customize its tasks. Let’s say you want the bot to greet different users:
function greetUser(userName) { // userName is a parameter
alert('Hello, ' + userName + '!');
}
greetUser('Shrikanth'); // Alerts "Hello, Shrikanth!"
greetUser('Fraz'); // Alerts "Hello, Fraz!"
You can think of parameters as blank spaces in your instructions, waiting to be filled in when the function is called.
Default Parameters
But what if you call a function without giving it all the info it needs? No worries—JavaScript lets you set default parameters. It’s like giving LearnYardBot a backup plan
function greetUser(userName = 'guest') {
alert('Hello, ' + userName + '!');
}
greetUser(); // Alerts "Hello, guest!"
greetUser('Shrikanth'); // Alerts "Hello, Shrikanth!"
This way, even if you don’t give LearnYardBot a name, it still knows what to do.
Returning Values: Getting Something Back
Sometimes, you’ll want your function to give something back like a calculator giving you the result of a calculation. Our Bot can do that too, using return
:
function add(a, b) {
return a + b;
}
let sum = add(3, 4);
alert(sum); // Alerts "7"
When the return
the statement is hit, the function stops and sends the result back to wherever it was called.
Naming Functions: Keep It Simple, Keep It Smart
When naming your functions, think of it like labelling buttons in the control panel of a drink vending machine. You want the names to be clear and to the point, you wouldn't want to get Tea when pressing the coffee button or get water when pressing milk, right? It is always a good idea to "Label" things correctly, it not only makes your code simpler for you but also for all the fellow developers working with you.
A few examples of good naming convention could be:
- getAge(): Returns the age.
- calculateSum(): Adds up numbers.
- showMessage(): Displays a message.
The idea is that, at a glance of the function name one should be able to guess what the function might be doing.
Smaller functions - Cutting it down into smaller chunks
Sometimes, breaking your tasks into smaller functions isn’t just about reusability—it’s also about making your code easier to read. Imagine you’re building a complex machine. Instead of a giant, messy blueprint, you break it down into clear, labeled sections. That’s what functions do for your code:
function isPrime(num) {
for (let i = 2; i < num; i++) {
if (num % i === 0) return false;
}
return true;
}
function showPrimes(n) {
for (let i = 2; i < n; i++) {
if (isPrime(i)) {
alert(i);
}
}
}
showPrimes(10); // Alerts "2", "3", "5", "7"
By splitting out isPrime
, the showPrimes
function becomes much easier to read and understand. It’s like labeling the steps in an instruction manual.
Great, are we clear with functions now? Let me know in the feedback portal! Oh if you are new to coding, ensure you take down notes to help you remember later, no one likes to read things again and again, right? Unless it's my article (😉) where we make things easy for you!