Git: Crash course
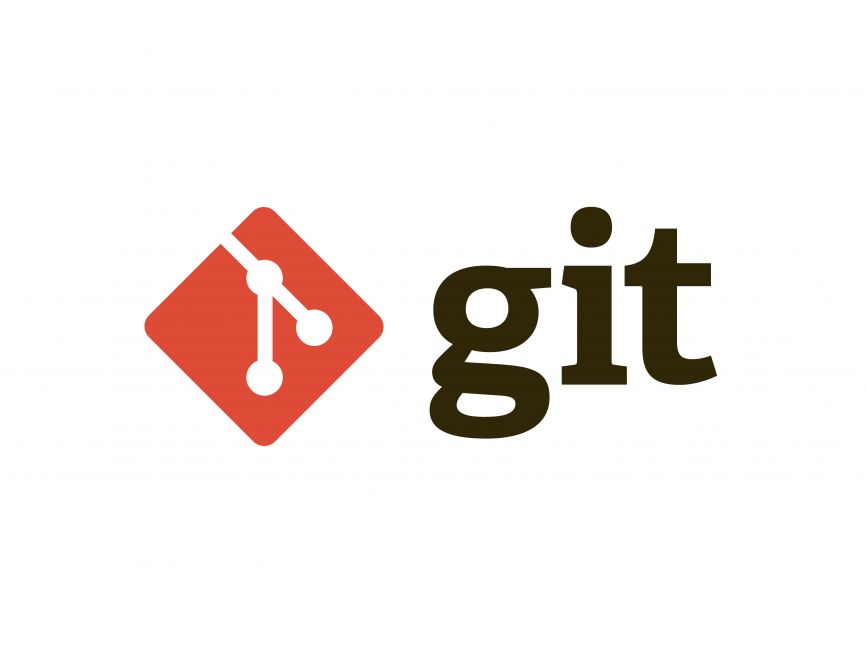
Version control is a system that manages changes to files and code over time, allowing multiple people to collaborate on a project and track modifications. It helps you keep a history of changes, revert to previous versions, and resolve conflicts when multiple people make changes simultaneously.
Imagine a PC game, just like you can save changes, try previous mission, or any of the unlocked missions or reset to the initial settings, in git as well, we can revert to previous "commits".
The "Why"
Let's quickly understand why we need something like a version control system (git).
Key features of version control systems include:
- Tracking Changes: Record who made changes, what was changed, and why.
- Branching and Merging: Work on different features or fixes in separate branches and then merge them back into the main project. A branch is like a particular version of the code, imagine a tree in which there are two main branch next to each other, they are equally long and take the same space, look the same too perhaps but are different, they might have different fruits and different number of leaves. To help you imagine better, imagine there is a chapter in a notebook, now it has a certain amount of content, and now you and your friend copy it into another page, you start adding more to this content, say a story. and your friend too adds more to it as per his need or imagination. In git, the "master" or "main" branch is like this initial story that you both got and the two improved versions of it are your "branches".
- Reverting Changes: Roll back to previous versions if needed. Now if the story you added is not turning out to be an interesting read, you might want to get back to the initial version, that is equivalent to reverting the change.
- Collaboration: Enable multiple developers to work on the same project without overwriting each other's work.
What is a git commit?
Imagine you’re working on a school project and you’ve made some changes to your report. You don’t want to lose these changes, and you want to keep track of what you’ve done so far. So, you take a snapshot of your report at this point and write a note about what changes you made. This snapshot and note help you remember what your project looked like and what you did at that moment.
In the world of coding, a "git commit" is like taking that snapshot and writing a note about it. Git is a tool that helps you keep track of your code changes. When you "commit" in Git, you’re saving a snapshot of your code at a particular point in time along with a message that describes what changes you made.
Just like with your school project, if you need to go back and see what your code looked like before a change, you can look at the commits to find that snapshot. And if you make a mistake, you can revert to a previous commit to undo the changes.
Types of Version Controls
There are two main types of version control systems:
- Local Version Control Systems: These keep track of changes on a single computer. Examples include tools like RCS (Revision Control System). They’re suitable for individual projects but don't support collaboration.
- Distributed Version Control Systems (DVCS): These manage changes across multiple computers and users, providing a complete history of all changes and facilitating collaboration. Popular examples include Git, Mercurial, and Bazaar. Git is widely used, especially with platforms like GitHub, GitLab, and Bitbucket.
The "How"
Let's understand the most popular git commands, please refer to this documentation to understand all the commands in git.
1. Initialize a Git Repository (if you haven’t already)
If you’re starting a new project, you first need to initialize a Git repository. Navigate to your project directory and run:
git init
2. Check the Current Status
Before making changes, it’s a good idea to see what’s happening in your repository:
git status
3. Make Changes to Your Files
Edit or add files to your project as needed.
git status
Now you should see the changed files in red.
4. Stage the Changes
Staging means preparing your changes to be committed. You can stage specific files or all changes. To stage all changes:
git add .
Or stage individual files
git add filename
5. Commit the Changes
Now, commit the staged changes with a descriptive message:
git commit -m "Your commit message here"
6. Create a New Branch
To create a new branch and switch to it:
git checkout -b new-branch-name
7. Push the Commit to a Remote Repository
Assuming you have a remote repository set up (e.g., on GitHub), you need to push your changes. First, make sure you’re on the correct branch (you can check with git branch
). Then push the commit:
git push origin new-branch-name
Note: If you do not have a repository follow these steps.
It's time to move on! See you in the next one.