Array Methods
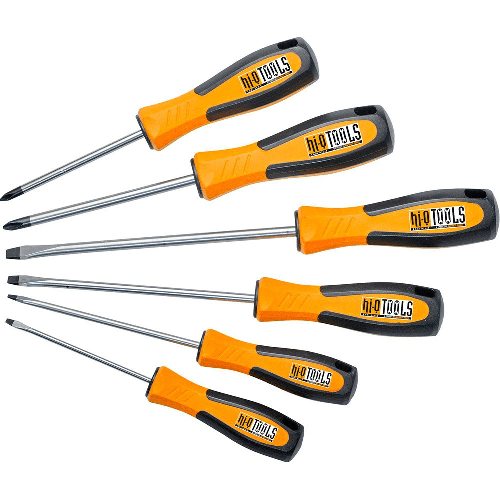
Imagine you're preparing for a family function — let's say, a grand Indian wedding. You’ve got your guest list, which, of course, includes everyone from your childhood neighbors to your mom's best friend’s cousin's maid! Now, organizing this list, making edits, checking RSVPs (Who has agreed to come and not in short ), or even finding out which guests are vegetarians becomes essential. In JavaScript, arrays are your go-to structure for managing all this data.
But what makes arrays truly versatile is their wide range of methods. These methods are like your event planner, caterer, and DJ all rolled into one — they help you add, remove, modify, search, and transform your array items effortlessly.
So in this article, we are going to understand each of these methods with examples, note that these come in very handy during development, so I would suggest you code along with this article and practice, I can't stress this more!
The important array methods are:
- push
- pop
- shift
- unshift
- slice
- splice
- map
- filter
- reduce
- find
- sort
1. push()
and pop()
push()
and pop()
, the tools for adding and removing items from the end of an array. Imagine you're adding last-minute guests to your event or removing those who can't attend at the last minute. These methods are perfect for handling such situations.
push()
The push()
method adds one or more elements to the end of an array. Think of it as adding more people to the last row of chairs at your event
let guestList = ["Aunt Meera", "Uncle Ravi", "Cousin Neha"];
guestList.push("Friend Shubham", "Neighbor Rajesh");
console.log(guestList);
// Output: ["Aunt Meera", "Uncle Ravi", "Cousin Neha", "Friend Shubham", "Neighbor Rajesh"]
This is how you push elements to the end of the Array.
pop()
But oh no! Last-minute cancellations. Your neighbor Rajesh has decided not to come (kuch urgent tha!). pop()
lets you remove the last element from your array.
guestList.pop();
console.log(guestList);
// Output: ["Aunt Meera", "Uncle Ravi", "Cousin Neha", "Friend Shubham"]
Just like that, we have learned two array methods now.
2. shift()
and unshift()
If push()
and pop()
work on the end of the array, then shift()
and unshift()
are the managers of the beginning. Think of them as handling those who are either the first to arrive or leave early.
unshift()
Your grandparents arrived early. Use unshift()
to add them at the start of your guest list:
guestList.unshift("Grandpa", "Grandma");
console.log(guestList);
// Output: ["Grandpa", "Grandma", "Aunt Meera", "Uncle Ravi", "Cousin Neha"]
shift()
On the flip side, let’s say Grandpa and Grandma decided to leave early. You can use shift()
to remove the first element from the array:
guestList.shift();
console.log(guestList);
// Output: ["Grandma", "Aunt Meera", "Uncle Ravi", "Cousin Neha"]
3. slice()
What if we wanted to extract a part of the guest list? Say, you want to arrange a fun game with 4 groups without cousins and friends, hence you need to divide the guestlist into 4, what would you use?
The slice()
method is the perfect tool to extract a subset of an array, without modifying the original one.
let youngGuests = guestList.slice(2, 4);
console.log(youngGuests);
// Output: ["Uncle Ravi", "Cousin Neha"]
The numbers in slice(2, 4)
represent the start index (2) and the end index (4, non-inclusive).
4. splice()
(Important)
But wait, Aunt Meera needs to be moved from the seating plan. Or maybe you want to replace her with Cousin Priya since there was a change of plan let's say. For that, we use splice()
. This method lets you add, remove, or replace elements in an array. It’s like having full control over your guest list!
Removing Elements with splice()
If Aunt Meera suddenly can’t make it, you can remove her with splice()
:
guestList.splice(1, 1); // Starts at index 1 and removes 1 element
console.log(guestList);
// Output: ["Grandma", "Uncle Ravi", "Cousin Neha"]
Adding Elements with splice()
Need to add Cousin Priya to the list? Easy:
guestList.splice(1, 0, "Cousin Priya");
console.log(guestList);
// Output: ["Grandma", "Cousin Priya", "Uncle Ravi", "Cousin Neha"]
Notice that this doesn’t delete anything, but instead inserts Priya at index 1.
Replacing Elements with splice()
Now let’s say you want to replace Cousin Priya with Uncle Raj. You can do both at once!
guestList.splice(1, 1, "Uncle Raj");
console.log(guestList);
// Output: ["Grandma", "Uncle Raj", "Uncle Ravi", "Cousin Neha"]
A versatile tool, splice()
gives you complete control over additions and deletions in your array.
5. map()
Now that your seating is in order, let’s personalize those invitations. Imagine you need to add a formal title to each guest's name. map()
is the perfect way to transform each item in the array and return a new array. Say something like "Dear Guestname", it adds a personal touch to the invitation.
let formalInvitations = guestList.map(guest => `Dear ${guest}`);
console.log(formalInvitations);
// Output: ["Dear Grandma", "Dear Uncle Raj", "Dear Uncle Ravi", "Dear Cousin Neha"]
The beauty of map()
is that it doesn’t modify the original array — it returns a new one with the transformed data.
6. filter()
Now let’s say your caterer needs to know which guests are vegetarians. You already have an array of guests with their dietary preferences, and you can use filter()
to return only those who are vegetarian.
let guests = [
{ name: "Grandma", vegetarian: true },
{ name: "Uncle Raj", vegetarian: false },
{ name: "Uncle Ravi", vegetarian: true },
{ name: "Cousin Neha", vegetarian: false }
];
let vegetarianGuests = guests.filter(guest => guest.vegetarian);
console.log(vegetarianGuests);
// Output: [{ name: "Grandma", vegetarian: true }, { name: "Uncle Ravi", vegetarian: true }]
filter()
is like your trusted bouncer, letting in only those who meet the criteria.
7. reduce()
Finally, it’s time to tally up your RSVPs and calculate how many guests are coming. reduce()
allows you to accumulate a single value (like a total) by applying a function to each item in the array.
let rsvps = [1, 1, 0, 1]; // 1 means RSVP’d, 0 means not coming
let totalGuests = rsvps.reduce((total, rsvp) => total + rsvp, 0);
console.log(totalGuests);
// Output: 3
Here, reduce()
adds up the values in the array and returns the total number of RSVPs. Think of it as your tally counter at the door.
8. find()
Now, let’s say you're looking for a specific guest, like your VIP guest Uncle Raj. find()
helps you search an array and return the first element that matches your condition.
9. sort()
Time to organize your seating alphabetically. Use sort()
to arrange your guest list in alphabetical order. Be cautious with numbers, though, as they are sorted lexicographically by default (more on that later).
guestList.sort();
console.log(guestList);
// Output: ["Cousin Neha", "Grandma", "Uncle Raj", "Uncle Ravi"]
This is a simple way to keep things in order.
These are the most important array methods you will work with although there are some more, you can check the mdn documentation for the whole list of array methods. But I would suggest you to run all the code we have shared here and try to make changes to learn by doing.