Introduction to Loops
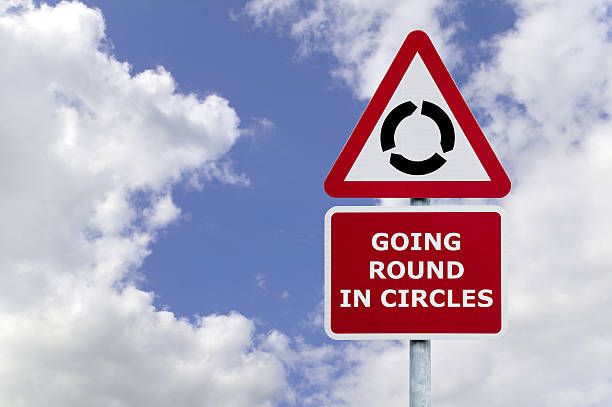
Learning to code can feel like learning a new language, and in a way, it is. Just like mastering any skill, it takes practice, patience, and understanding. Today, we're diving into an essential concept that you'll find yourself using again and again in JavaScript: loops. Loops are like the "horses" of programming—they let you do repetitive tasks without getting bored, because hey, computers don’t mind doing the same thing over and over (Lazy toh hum hai!)
What are Loops?
In everyday life, you might loop through tasks without even realizing it. Imagine you're preparing a batch of cookies. You mix the ingredients, shape the dough into cookies, and then bake them, right? You don’t just make one cookie! You repeat the process until all the dough is used. It is similar to making that tasty and crispy dosa, right? Similarly, loops allow you to repeat a block of code multiple times in coding. This is particularly useful when you need to perform the same operation on multiple items, such as processing each element in an array or running a function a set number of times.
In summary, a loop is nothing but running a set of instructions or just a single instruction a set number of times or till a condition is met.
Types of Loops in JavaScript
JavaScript offers several types of loops to suit different needs. Let’s explore each one in brief in this article, there are separate articles discussing about each of them in detail, I would suggest going through them too.
- for Loop: The
for
loop is the most basic loop there is; it's versatile and can handle most looping tasks. It consists of three parts: initialization, condition, and iteration. This loop runs as long as the condition is true. Keep making a certain number of cookies, say we need 10 cookies, we repeat the steps till we are done making as many cookies. - while Loop: The
while
loop is like an engine that never stops itself! —it keeps going until you tell it to stop. It’s ideal when you don’t know beforehand how many times you need to loop but there is an exit condition. This is like we keep making cookies till some condition - like the power goes off, we run out of dough or mom just said "Hat jao kitchen say ab!" - do...while Loop: The
do...while
loop is similar to thewhile
loop, but it guarantees that the code block runs at least once. It’s like testing the dough before you decide if you have enough to make another cookie. - for...in Loop: This loop is particularly useful for iterating over the properties of an object. It’s like checking the ingredients in your pantry.
- for...of Loop: This loop is handy when you want to loop through values of an iterable object, like an array. Think of it as tasting each cookie to ensure it's perfect. Don't do that in real life, please!
- forEach Method: The
forEach
method is a more modern and convenient way to loop through arrays. It's like having a conveyor belt that automatically processes each cookie without you having to manage the process manually. - map Method: The
map
method is similar toforEach
, but with a twist: it creates a new array by transforming each element in the original array. It’s like starting with plain dough and ending up with a tray of perfectly shaped cookies.
Each loop has its place in JavaScript. The for
loop is great for when you know how many times you need to repeat an action. The while
loop shines when the number of iterations isn’t predetermined. The do...while
loop is your go-to when you want the loop to execute at least once. for...in
and for...of
loops are excellent when working with objects and arrays, respectively (We will learn more about them later).
A word of caution: Be careful with loops, especially while
loops. If the condition never becomes false, the loop will keep running indefinitely—like trying to bake cookies with an endless supply of dough! This can crash your program or browser. Always make sure there’s a way to exit the loop. Because just like you the computer also gets tired after a while!
Conclusion
Loops are an indispensable part of programming in JavaScript. They help automate repetitive tasks, making your code more efficient and easier to manage. With practice, you’ll become more comfortable choosing the right loop for the job.
So are you ready to start learning more about each of the loops, see you in the next article!