Return Statements
Overview
Today, we will learn about return statements. ✌️ In the previous articles, we discussed that functions take input and provide output (known as the return value). Until now, we've been using "void" as the return type for our functions, indicating that the functions don't return any specific value.
However, we can introduce a "return" statement inside a function.
The "return" statement:
- Signals the end of a function's execution.
- Sends control back to the point in the program where the function was called.
Note: When a return statement is executed, the function ends immediately, and nothing after this will be executed.
Example
Let's illustrate this with an example. Consider our code for finding the product of two numbers.
Let's add a return statement inside the function definition.
Code
#include <iostream>
using namespace std;
// function definition
int MultiplyNumbers(int number1, int number2) {
int result = number1 * number2;
return result;
}
int main() {
// function call
int result1 = MultiplyNumbers(6,8);
cout<<"Result 1 : "<<result1<<endl;
return 0;
}
Output
Result 1 : 48
You might have noticed that we have used int instead of void in MultiplyNumbers( ). This is because this time, the function returns an integer value ( denoted by the return result; ). When the return result; is encountered, the function terminates and control of the program jumps back to the function call.
Note: When a return statement is executed, the function terminates immediately and the control returns back to the calling function.
Explanation
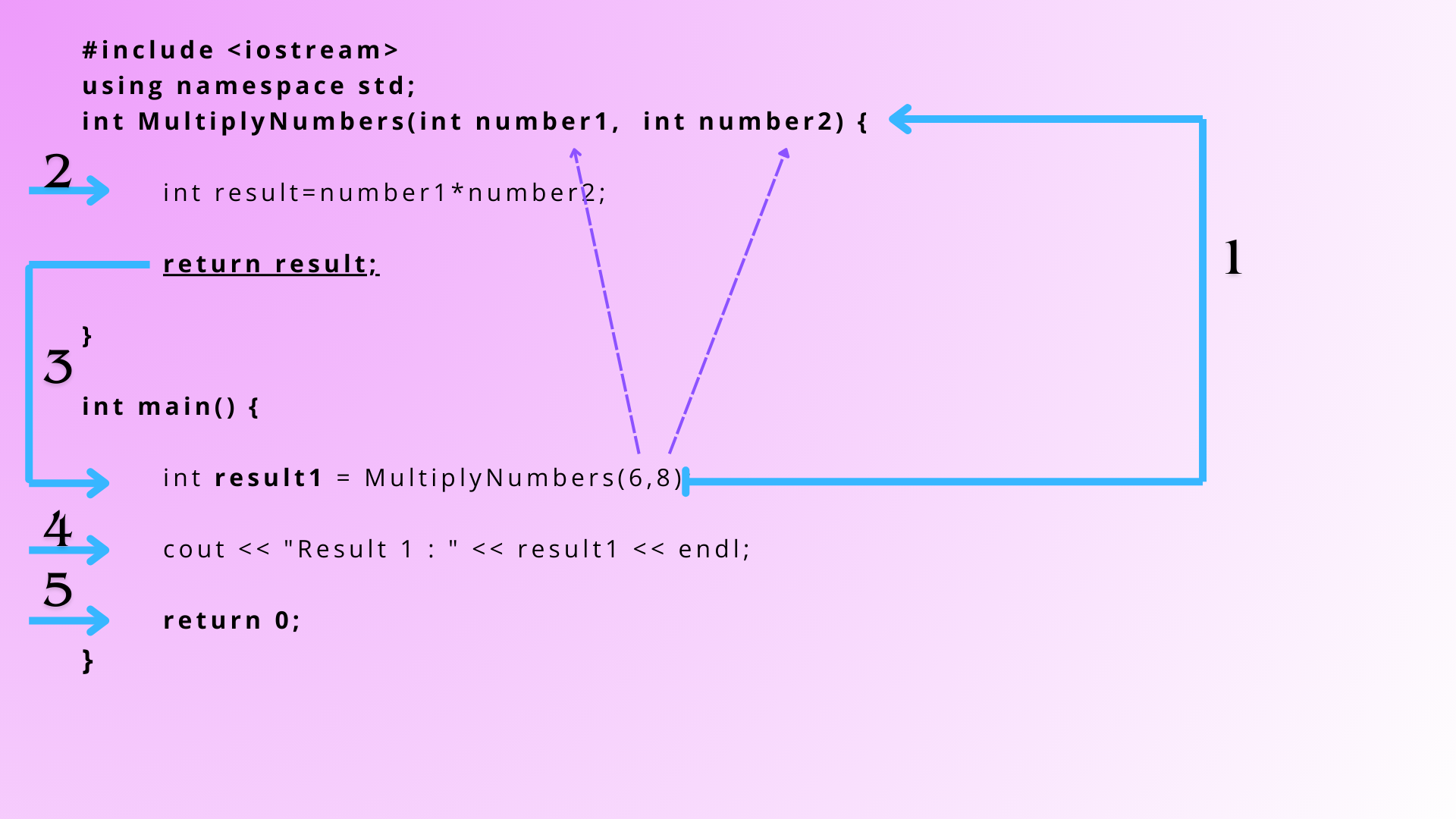
- As I have mentioned in the previous articles many times, in C++, the program always starts from the main function. Then, it executes line by line.
Now, let's understand the flow of the program.
- As the function MultiplyNumbers( ) is encountered, the program pauses the main function and jumps to the MultiplyNumbers( ) function. As we have called the MultiplyNumbers( ) function with arguments(6,8). The value is assigned to number1 and number2 respectively. ( 1 in the diagram shows this flow)
- Then, the MultiplyNumbers( ) function starts executing line by line by line. Inside the function, we have computed the product of numbers using int result = number1 * number2. ( 2 in the diagram shows this flow)
- Now, when the return statement of the function is encountered, the program's control jumps back to the main function and the main function again starts executing line by line. Since the function is returning an integer value, we have assigned the function call to the integer variable result1. We store the result in variable result1. ( 3 in the diagram shows this flow)
- Now print statement will be executed and the output will be printed. ( 4 in the diagram shows this flow)
- Finally, when return 0; is encountered, we exit from the program. ( 5 in the diagram shows this flow).
Note: We can call the function multiple times.
Example: Multiple return statements
Let's illustrate this with an example. Consider a program to find out if a number is odd or even using a function.
#include <iostream>
using namespace std;
// The function checks if a number is even (true) or odd (false).
bool CheckParity(int number) {
if (number % 2 == 0) {
return true;
} else {
return false;
}
}
int main()
{
int num;
cout << "Enter a number: " ;
cin >> num;
bool result = CheckParity(num);
// Prints if the result is true (even) or false (odd).
if (result) {
cout << "Number is even" << endl;
} else {
cout << "Number is odd" << endl;
}
return 0;
}
In the above code, two return statements are used inside the CheckParity function. But, there is a catch. We have used the return statements inside if-else blocks. Hence, when the number is even, the return statement inside the if block will be executed and the function will return true. When the number is odd, the return statement inside the else block will be executed and the function will return false.
There are some times when you want more than one return statement. This occurs when there is some operation that you want to stop - such as in recursion(This topic we will do later). So, we can use multiple return statements also in our functions according to the need. ✌️
main() Function
So far, we have been using a main( ) function in all of our programs. If we see the syntax it looks similar to that of a function.
int main() {
return 0;
}
These are components of the above code:
- main() - name of the function
- int - return type of the function
- return 0 - the function returns integer 0
Note: The execution of a C++ program starts from the main( ) function. So every valid C++ program should include a main( ) function.
Why does the main function return 0?? Can we figure out? 😄
The return value of main( ) becomes the exit status of the process. Return 0 is used to indicate that the program has executed successfully and is returning a value of 0 to the operating system.
Similarly, we can also use return 1 based on some conditions. Return 1 in the main function means that the program did not execute successfully and there is some error.
The one who initiates the execution is the one who is responsible for the end as well. Our one and only main function. ✌️😄
With this article, we complete the basics of functions that are necessary to write a function. I hope you have understood these concepts clearly. Understanding the basics of a topic is essential for problem-solving and concept clarity. Problems change over time but concepts don't.
See you in the next article with more energy and excitement ✌️ till then Happy Learning !! 😀