while Loop
What are Loops?
Imagine you have a bunch of toys you want to put in a toy box. You could pick up one toy, put it in the box, then go back for the next one. This would take a lot of time.
Now, think of loops as a magic hand that grabs toys one by one and puts them in the box for you. It keeps doing this until all the toys are in the box. In programming, loops are like that magic hand, helping us do repetitive tasks quickly and easily.
Another example
Let's say you want to write a program that prints "Hello" 1000 times. Without loops, you'd have to write print("Hello")
1000 times, which would be quite a chore. But with loops, you can simply tell the computer, "Hey, print 'Hello' a bunch of times for me!" and it will do the job for you.
Loops make it super easy to repeat tasks in programming, saving you a ton of time and effort.
Would you like to explore how to use these magical loops in programming?
The while Loop
In programming, a while loop is like a repeat-until loop. You keep doing something as long as a certain condition is true. Here's how it looks:
while(boolean expression){
//statement(s)
}
How it works:
- First, you check a condition, like asking, "Is it sunny?"
- If the answer is "yes" (condition is true), you do something, like playing outside.
- Then, you go back and check the condition again. If it's still "yes" (still sunny), you keep playing outside.
- This keeps going until the condition becomes "no" (cloudy or raining), and then you go inside.
Example
int count = 1;
while (count <= 5) { // Keep going while count is less than or equal to 5
cout << "Count: " << count << endl;
count++; // Increment count by 1
}
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
Explanation
- We start with the variable
count
set to 1. - The while loop is initiated with the condition
count ≤ 5
, which means it will run as long ascount
is less than or equal to 5. - Inside the loop, it prints "Count: " followed by the current value of
count
, which is initially 1. So, it prints "Count: 1". - After printing, it increments the value of
count
by 1 usingcount++
, making it 2. - The loop goes back to the condition, which is still true (2 is less than or equal to 5), so it repeats the process.
- It prints "Count: 2" and increments
count
to 3. - This continues until
count
reaches 6, at which point the condition becomes false (6 is not less than or equal to 5). - The loop stops, and the program ends.
Count: 1 Count: 2 Count: 3 Count: 4 Count: 5
Yet Another Example
#include <iostream>
using namespace std;
int main() {
int balloons = 5; // Start with 5 balloons
cout << "Let's have a balloon popping party!" << endl;
while (balloons > 0) { // Keep popping balloons until there are none left
cout << "Pop! One balloon bursts!" << endl;
balloons--;
if (balloons > 1) {
cout << "There are " << balloons << " balloons left!" << endl;
} else if (balloons == 1) {
cout << "There is 1 balloon left!" << endl;
} else {
cout << "No more balloons left. Party's over!" << endl;
}
}
return 0;
}
Let's have a balloon popping party!
Pop! One balloon bursts!
There are 4 balloons left!
Pop! One balloon bursts!
There are 3 balloons left!
Pop! One balloon bursts!
There are 2 balloons left!
Pop! One balloon bursts!
There is 1 balloon left!
Pop! One balloon bursts!
No more balloons left. Party's over!
Explanation
- First, we start with 5 balloons. The variable
balloons
is set to 5. - The program displays a cheerful message: "Let's have a balloon popping party!" to set the mood.
- We enter a while loop, which keeps going as long as there are balloons left to pop.
- Inside the loop, it makes a popping sound: "Pop! One balloon bursts!" as if we're popping a balloon.
- The program keeps track of the number of balloons left by decreasing the value of
balloons
by 1. If we started with 5 balloons, now we have 4. - After popping a balloon, the program checks how many balloons are left. If there are more than 1, it says "There are X balloons left!" where X is the number of balloons left.
- If there's only one balloon left, it says "There is 1 balloon left!".
- If there are no more balloons left (when
balloons
becomes 0), it says, "No more balloons left. Party's over!". - The program continues looping and popping balloons until there are no more left to pop.
- When all the balloons are popped (no balloons left), the loop stops.
- The program finishes with a cheerful message indicating the end of the party.
Flowchart of while Loop
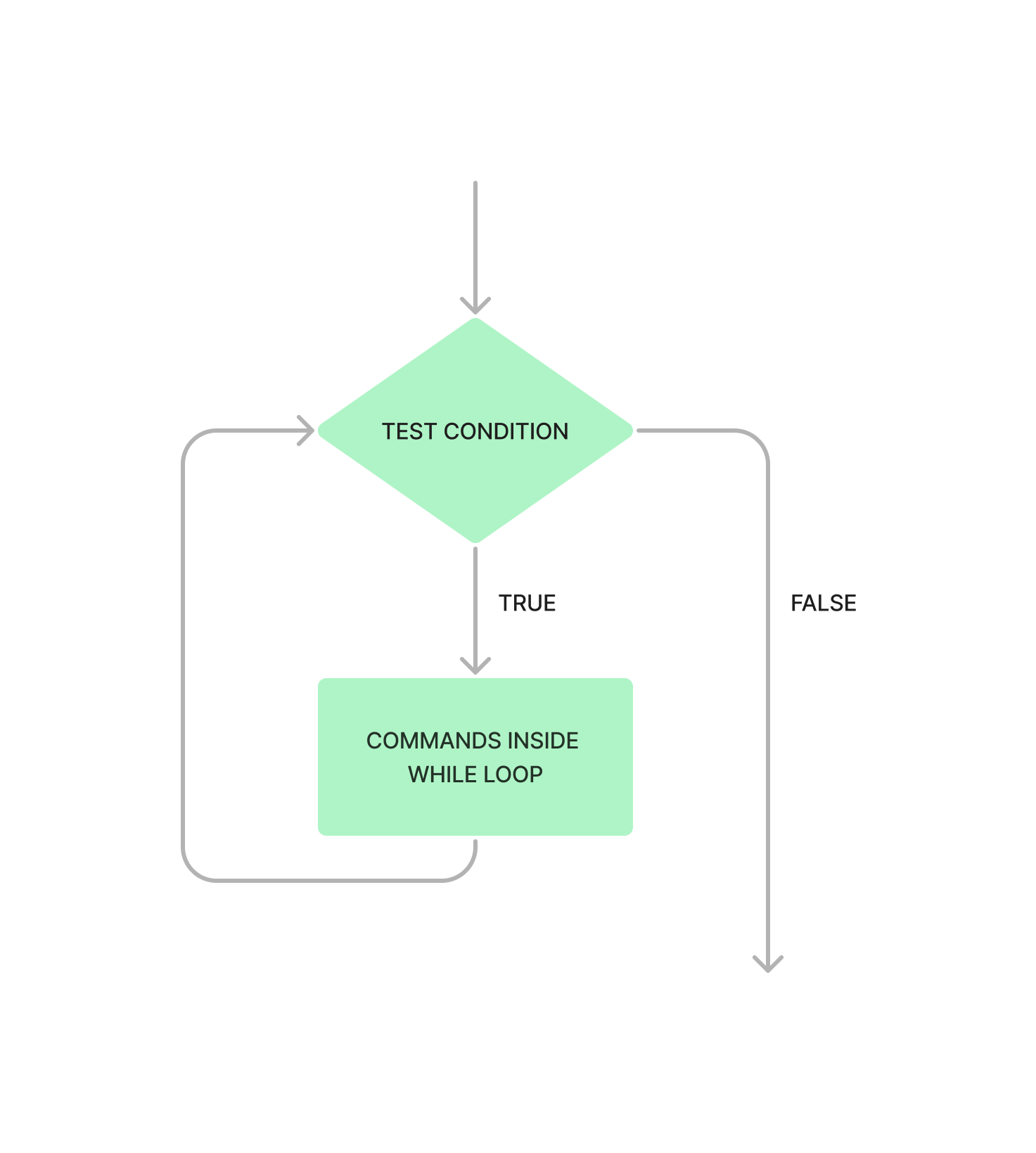
Introductory Problem : Print Numbers From 5 to 1 Using while Loop
#include <iostream>
using namespace std;
int main() {
int number = 5; // Start from 100
while (number >= 1) {
cout << number << endl;
number--;
}
return 0;
}
5
4
3
2
1
Quiz
What is the output of the following code?
#include <iostream>
using namespace std;
int main() {
int x = 10;
while (x <= 14) {
x++;
cout << x << " ";
}
return 0;
}
Tasks
Write a program that takes two inputs (a and b) from the user and prints the numbers from a to b using while loop.
C++ Code
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter the starting number (a): ";
cin >> a;
cout << "Enter the ending number (b): ";
cin >> b;
cout << "Numbers from " << a << " to " << b << " are: ";
while (a <= b) {
cout << a << " ";
a++;
}
return 0;
}
Now print the numbers in the reverse order from b to a using while loop.
C++ Code
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter the starting number (a): ";
cin >> a;
cout << "Enter the ending number (b): ";
cin >> b;
cout << "Numbers from " << a << " to " << b << " in reverse order are: ";
while (b>=a) {
cout << b << " ";
b--;
}
return 0;
}
do...while Loop
Hey there, students! Think of the do...while loop like a fun adventure. You take a step, check your compass, and keep going until you decide it's time to stop. Just like exploring a magical forest with surprises along the way. Let's jump into the world of do...while loops and have some coding adventures!
In C++ programming, there is another version of the while
loop known as the do...while
loop.
Syntax
do {
// statement(s)
} while (boolean_expression);
Here's how this code works.
- First, the code inside the do...while loop runs. It's like the first step of our journey.
- After that, we check a condition (the boolean expression).
- If the condition is true, we go back to the beginning and do the loop again, just like taking another step on our journey.
- If the condition is false, the journey ends; we're done.
So, in a do...while loop, we always take that first step (execute at least once), and then we decide if we want to continue based on the condition. It's like starting an adventure, and you decide if you want to keep going after the first part.
Now, let's dive into an example to see it in action!
Introductory Problem
Let's play a counting game! Start with the number 1 and keep counting up. Your mission is to count until you reach a number that is divisible by 7. When you find it, shout 'Lucky 7!' and the game ends. Can you find the lucky number?
#include <iostream>
using namespace std;
int main() {
int number = 1;
do {
cout << "Count: " << number << endl;
number++;
} while (number % 7 != 0);
cout << "Lucky 7!" << endl;
return 0;
}
Explanation:
We're playing a counting game where we start at 1 and keep counting up. The goal is to find the number that is divisible by 7 and shout 'Lucky 7!' when we find it.
- Start with the number 1 and set the variable
number
to 1. - Enter the do...while loop, which is our counting game.
- Inside the loop, print "Count: 1" because
number
is 1. - Increment
number
by 1, making it 2. - Check if the new number (2) is divisible by 7. It's not, so continue.
- Print "Count: 2" and increase
number
to 3. - Keep repeating this process, counting and increasing
number
- Continue until we reach a number (in this case, 7) that is divisible by 7.
- Once we reach 7, which is divisible by 7, the condition
number % 7 != 0
is no longer true, so we exit the loop. - We celebrate our success with a shout of 'Lucky 7!' because we found the lucky number!
That's how our counting game using a do...while loop works. We keep counting until we find our goal, in this case, a number divisible by 7.
while vs do...while Loop
Remember that do while executes at least once no matter what?
This is the key difference between a while and do while loop.
Let's demonstrate this to you.
#include <iostream>
using namespace std;
int main() {
int x = 10;
do {
cout << "This will be executed at least once, even though x is not greater than 15." << endl;
} while (x > 15);
return 0;
}
Output
This will be executed at least once, even though x is not greater than 15.
In this example, the do...while loop's body is executed at least once, even though the condition x > 15
is false. The loop checks the condition after the first execution.
Let's now see what happens in the case of a while loop.
#include <iostream>
using namespace std;
int main() {
int x = 10;
while (x > 15) {
cout << "This won't be executed because the condition (x > 15) is false." << endl;
}
return 0;
}
In this case, nothing is printing, as the program execution is not going inside the while loop as the condition is false.
So, the key difference is that the while loop checks the condition first, while the do...while loop ensures at least one execution before checking the condition.
Quiz
What is the output of the following code?
int number = 1;
do {
cout << number << " ";
number += 2;
} while (number <= 10);
🚀 What's Coming Next?!! 🌟
In the next articles, we're taking our 'while loop' journey to the next level! 🎉🔍 We've got some fascinating and challenging problems lined up that will make you a 'while loop' master. 🚀💡 Get ready for an exciting coding adventure that's just around the corner!