if...else Statement
Introduction
You're planning a weekend outing, and the weather forecast is predicting a day of potential rain. You have two options in mind: a day at the beach or a visit to a nearby museum. Your way of making choices is similar to using an "if-else" statement in everyday life.
Scenario:
- "if" the weather is sunny and clear, you decide to go to the beach to enjoy the sun, sand, and water.
- "else," if the weather forecast indicates rain or storms, you opt for a visit to the museum, appreciating indoor activities over getting wet and sandy.
This simple decision-making process mirrors how computer programs use "if" and "else" to make choices. It's a fundamental concept in both everyday decision-making and programming, that ensures the right course of action is taken based on certain conditions or circumstances.
Now let's consider a programming task example,
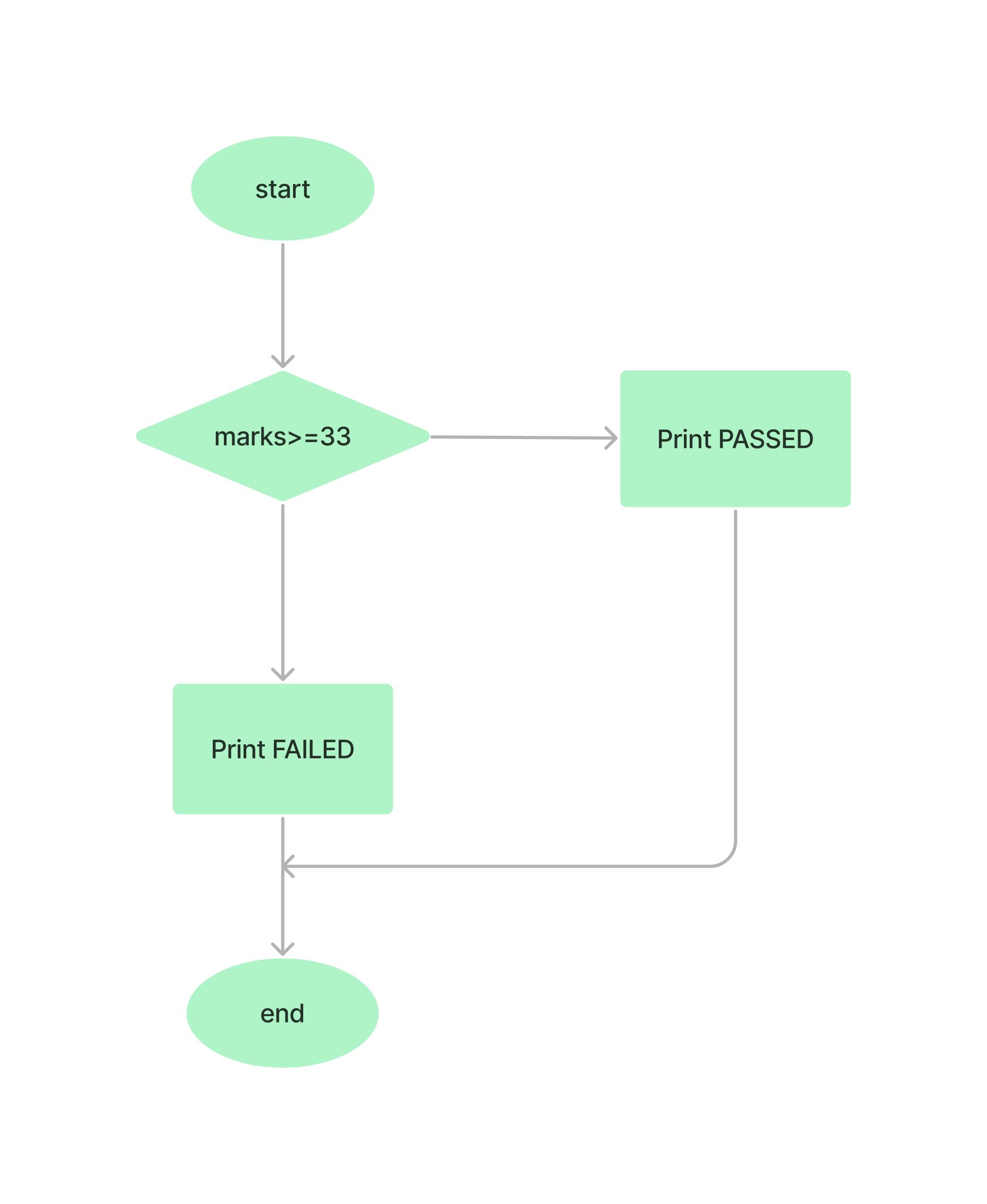
Here, we need to check the marks of the student; if he got marks >= 33, then print passed; otherwise, print failed.
So here we want to execute one instruction (print passed) if the condition is true, otherwise another instruction (print failed).
The if statement
Syntax -
if (when a certain condition is met) {
// Do this
}
How does this work?
The if statement assesses whether a given condition is met, and this condition can result in either a "true" or "false" outcome.
If the condition is met (which can be thought of as a "yes" answer) - then the actions within the if statement are carried out.
If the condition is not met (which can be seen as a "no" answer) - the actions within the if statement are skipped.
Let's move on to an example to see this in action!
#include <iostream>
using namespace std;
int main() {
int student1_score = 75;
int student2_score = 20;
int passing_score = 33;
if (student1_score >= passing_score) {
cout << "Student1 passed the exam." << endl;
}
if (student2_score >= passing_score) {
cout << "Student2 passed the exam." << endl;
}
return 0;
}
Student1 passed the exam.
Explanation
"Student2 passed the exam" is not printed, because the condition in the second if statement (student_score2 >= passing_score) is false.Quiz
What is the output of this code?
#include <iostream>
using namespace std;
int main() {
int temperature = 25;
if (temperature > 30) {
cout << "It's a hot day!" << endl;
}
cout << "This is always executed." << endl;
return 0;
}
The else Statement
Why We Need the else Statement
Imagine you want to tell someone whether it's a good day for outdoor activities. If the weather is sunny, you might say, "It's a good day to go outside." But if it's rainy or cloudy, you would say, "It's not a good day to go outside." The if statement alone can help with the first part, but what about the second part? That's where the else statement is useful –– it provides an alternative action when the condition is not met.
#include <iostream>
using namespace std;
int main() {
bool isSunny = true;
if (isSunny) {
cout << "It's a good day to go outside." << endl;
} else {
cout << "It's not a good day to go outside." << endl;
}
return 0;
}
In this code:
- The if statement checks whether isSunny is true. If it is, it prints, "It's a good day to go outside."
- The else statement handles the case when isSunny is not true (in this case, when it's `false). It prints, "It's not a good day to go outside."
Another Example: Vending Machine
Imagine we're coding a vending machine program in C++. It will dispense a snack if the user inserts enough money, and if not, it will return the money. We'll use if and else statements to make decisions based on the user's input.
Some Common Beginners' Mistakes
Using a Single Equal Sign (=) for Comparison
Instead of using == to compare values in an if statement, beginners sometimes use a single equal sign, which is the assignment operator. This mistake can lead to unintended variable assignments.
Example of the mistake:
if (x = 10) {
// This will always be true because x is assigned 10.
}
Using Semi-Colons Incorrectly
Placing a semicolon after an if statement can cause issues. The semicolon effectively ends the statement, so the code block that follows the if statement is not part of the condition. So irrespective of the condition that code block will get executed.
Example of the mistake:
if (x > 5); {
cout << "This will always be executed.";
}
Confusing Logical Operators
Mixing up logical operators like && (logical AND) and || (logical OR) can lead to incorrect results. Beginners might use the wrong operator, which can affect the condition's logic.
Example of the mistake:
if (x && y > 10) {
// The intent might be to check both conditions, but this is not how it works.
}
The right way of checking if both x and y are greater than 10 or not is :
if (x > 10 && y > 10) {
/*
This is how you check both the conditions. Both conditions need to be true to run the code of this if block.
*/
}
Adding Condition in else Block
Beginners often incorrectly use the else
statement with a condition in parentheses, like else (condition)
, which is not valid in C++. The correct syntax is to use else
without conditions for simple alternative actions.
if (number < 0) {
cout << "Negative Number";
}
else (number > 0) { // Common mistake
cout << "Positive Number";
}
This is wrong, and will give compilation error.
What's Coming Next !! 👩💻👨💻
Congratulations, you've now mastered the fundamentals of if-else
statements in programming. You've learned how to make decisions in your code, paving the way for more sophisticated problem-solving and application development.
But wait, the journey doesn't end here! Our programming adventure is just getting started, and the next chapter is something truly exciting. In our upcoming article, we're going to delve into the captivating world of else if
statements.