Data Types
What are data types?
As in the previous lesson, we have learned that every container/variable has uniqueness to store the data. We cannot store Apple
in the Stationary box
and Stationary
in Spice Box
.
Therefore every variable needs to specify the type of data it will store which is ultimately assigning the Data Type
to a variable.
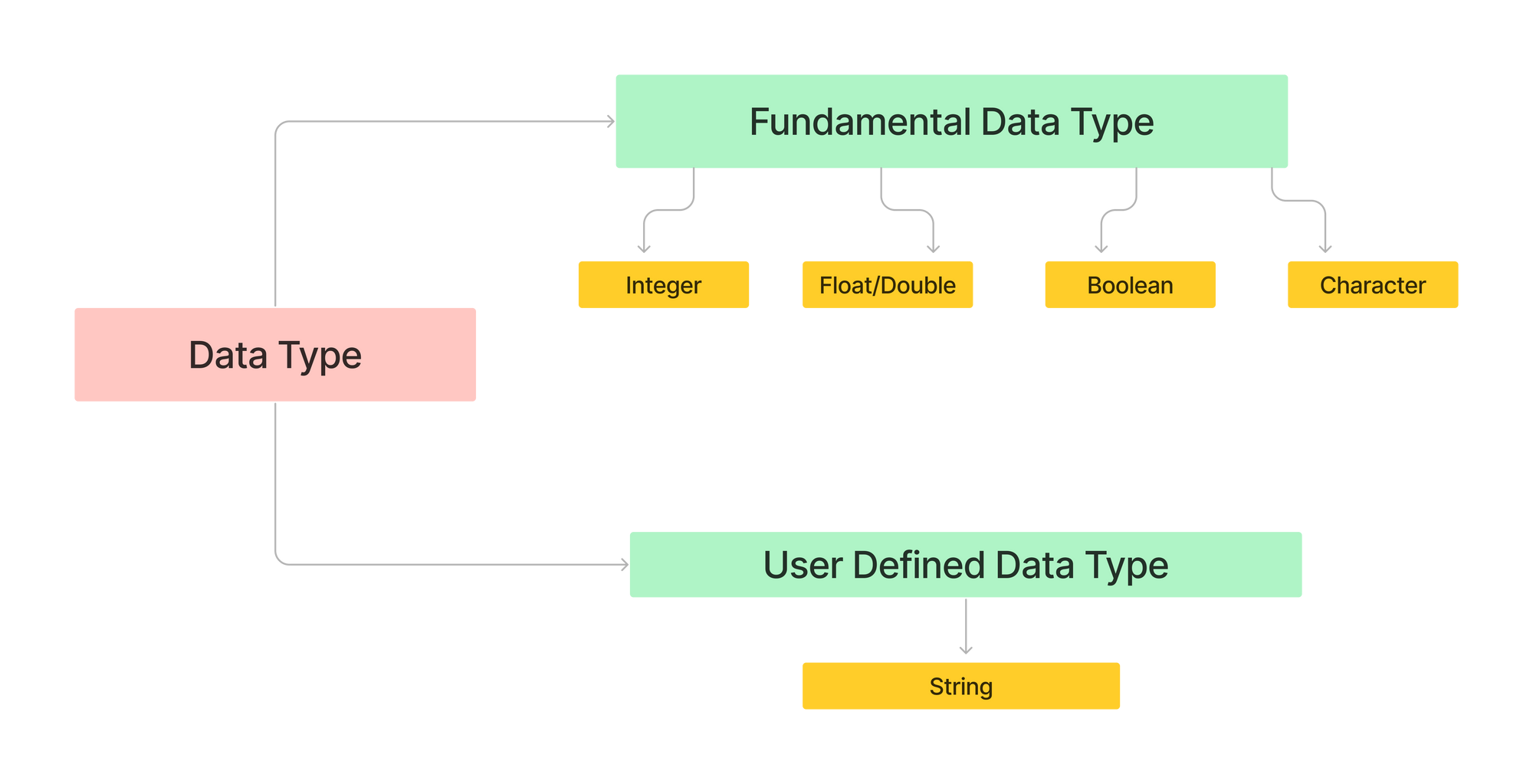
Data types can be broadly classified into three categories:
1 . Primary or Built-in or Fundamental data types
2. Derived data types
3. User Defined data types
We will only be discussing primary data types and some user-defined data types, the rest we will cover in our further lectures.
Let's discuss all the important datatypes one by one:
1. Integer (int)
The keyword used for integer data types is int. It is used to store all positive and negative integers like -81, 10, 9, 1,0. Its value ranges from -2147483648 to 2147483647.
int apples = 5; // You have 5 apples.
2. Double or Float
The float data types are used to store positive and negative numbers with a decimal point, like 35.3, -2.34, or 3597.34987.
float money = 10.50; // You have ₹10.50.
3. Boolean (bool)
A Boolean datatype is used to store either true or false. The keyword used for the Boolean data type is bool.
bool isRaining = true; // It's true that it's raining today.
4. Character (char)
Character data type stores only single characters, including alphabetical characters, number characters, and special characters like ' Z ', '5', ' _ ', and '?'. Character data is always placed within single quotes(' ').
char firstLetter = 'A'; // The first letter of your name is 'A.'
5. String ( string )
A string data type is a user-defined data type that is being used to store a sequence of characters. Since it is not a built-in data type, we have included a string header file to create string variables. Some examples: "river", "Gautami", "I am Ravish", "Hello Yardians" etc. Always string data is placed within double quotes (" ").
string bookTitle = "Bhagavad Gita"; // Your favourite book name.
Printing Variables
We already know how to print int
variables. Now, let's use the same concept to print double
variables.
#include<iostream>
using namespace std;
int main(){
double savings = 1230.75;
// Printing savings
cout << " My savings : " << savings;
return 0;
}
Output :
My Savings : 1230.75
Now, let's see an example of how we can use character
variables in C++.
#include<iostream>
using namespace std;
int main(){
char alphabet = 'U';
// Print alphabet
cout << " Alphabet is : " << alphabet;
return 0;
}
Output :
Alphabet is : U
Similarly printing a string
variables :
#include<iostream>
using namespace std;
int main(){
string message = "LearnYard";
cout << message ;
}
Output:
LearnYard
IMPORTANT
Don't confuse Character
with String
as both of these resemble. In C++, we use single quotes to represent characters and double quotes to represent strings. Hence,
-
"m", "5" and "message" are examples of strings (represented by double quotes)
-
'm', '5', '+' are examples of characters (represented by single quotes)
PRACTICE
- Create two variables
number1
andnumber2
with values 123 and 12451.34. - Print
number1
andnumber2
in different lines.
Expected Output :
123
12451.34
Solution Code :
#include <iostream>
using namespace std;
int main(){
int number1 = 123; // int type variable
double number2 = 12451.34; // floating-point type variable
cout << number1 << endl;
cout << number2 << endl;
return 0;
}
QUIZ
Changing Values Stored in Variables
We can change the value stored in variables as per our needs.
#include<iostream>
using namespace std;
int main(){
int num = 13;
cout << num << endl;
num = 89; // new value is assigned
cout << num << endl;
return 0;
}
Output:
13
89
We can observe that firstly 13
is being printed and in the second step a new value 89
is being assigned to the same variable num
. Therefore 89 is printed afterwards.
Similarly, we can change the value assigned to any variable in the code after initialization.
QUIZ
What's the output of this code?
#include <iostream>
using namespace std;
int main() {
double number = 25.5;
number = 66.6;
number = 535.259;
cout << number;
return 0;
}
Value of One Variable to Another
It's also possible to assign the value of one variable to another. Let's take an example.
#include <iostream>
using namespace std;
int main() {
int num1 = 100;
cout << num1 << endl;
int num2 = 200;
// assign value stored in num2 to num1
num1 = num2;
cout << num1;
return 0;
}
Output:
200
Initially value of num1
was 100 but after assigning a value of num2
to num1
the original value is being overwritten by 200.
Let's take another example:
#include <iostream>
using namespace std;
int main() {
int num1 = 100;
cout << num1 << endl;
// assign value stored in num2 to num1
num1 = num2;
int num2 = 200;
cout << num1;
return 0;
}
Output:
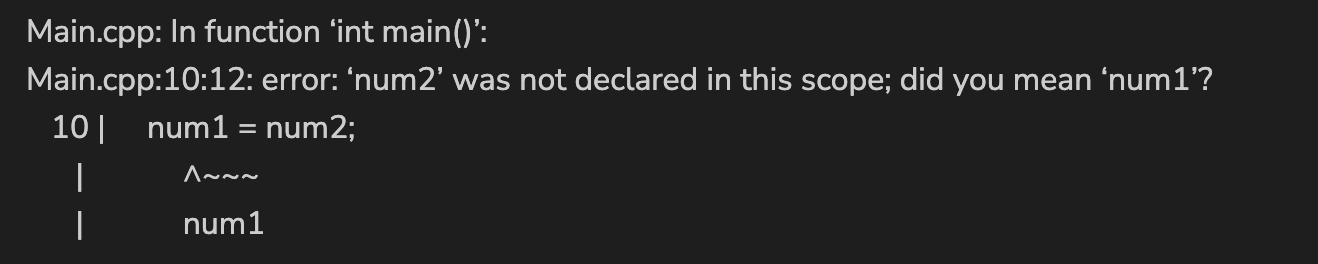
In the above example, the line num1 = num2;
results in an error. It's because up until that that point, the num2
variable has not been defined.
Remember, the code runs sequentially. Hence, the computer is not able to find the num2
variable, which is defined only after using it.
Creating multiple variables together
Instead of creating multiple variables line by line, we can declare two or more variables of the same data type in a single line.
#include<iostream>
using namespace std;
int main(){
int num1 = 100;
int num2 = 200;
return 0;
}
Alternate Way:
#include<iostream>
using namespace std;
int main(){
int num1 = 100, num2 = 200;
return 0;
}
Selecting Proper Variable Names
Declaring variables with appropriate names is very necessary for writing clean and readable code.
For example :
int b = 1000;
int bonus = 1000;
Among both of them, definitely 2nd one is preferred because of its variable name is self-explanatory.
Some important points to take care of while creating variables :
- The name of the variable should not be a predefined
keyword
in C++ language such as delete, for, cout, string, char, while, static etc. Because these keywords have a specific meaning in C++. - Variable names should be clear and concise. Example :
Salary
instead ofs
,Number1
instead ofn1
. - If the variable name consists of two or more words try using Snake Case or Camel Case to create a name.
Example :
todayscurrentdate
Instead use:
current_date ( Snake Case: Inserting an underscore between words )
OR
currentDate ( Camel Case: Capitalizing the first letter of a new word)
- You cannot use spaces or other symbols (except alphabets, numbers, and underscore) as variable names. Some invalid variable names :
full name
,1number
,salary $
etc.
C++ variables conduct the language's symphony, each datatype a note, crafting a readable composition that resonates with clarity and efficiency