Function Within a Function-Nesting of Functions
Overview
In this article, we will learn about functions calling other functions.
Most of us have witnessed the journey of complaints for the serious mischievous behaviours in our school. The class monitor calls the subject teacher, then the subject teacher calls the class teacher, and then the class teacher calls the supervisor. And if we have done something really serious, the supervisor calls the principal. Suppose each person involved is a function. Now, we can visualize the calling of a function within a function. ( Maybe visualizing this was not a very pleasent experience. 😂 )
Example
Let's see an example to understand this:-
In this example, we will calculate the square of the sum of two numbers using many functions that will be calling each other to understand the concept.
Code
#include <iostream>
#include <cmath>
using namespace std;
void PrintSquare(int sq){
cout << sq * sq;
}
void Square(int sum){
PrintSquare(sum);
}
void Add(int a, int b){
int sum = a + b;
Square(sum);
}
void CalculateSquareOfSum(int num1, int num2){
Add(num1, num2);
}
int main(){
int a,b;
/* Input numbers from user */
cout << "Enter number 1: ";
cin >> a;
cout << "Enter number 2: ";
cin >> b;
//We have to calculate Square Of Sum Of two Numbers (a+b)^2
CalculateSquareOfSum(a,b);
return 0;
}
In the above code, we can see that main function calls CalculateSquareOfSum function which calls Add function inside it, Add function calls the Square function within it and then inside Square function finally PrintSquare is called.
Flow of Function Call
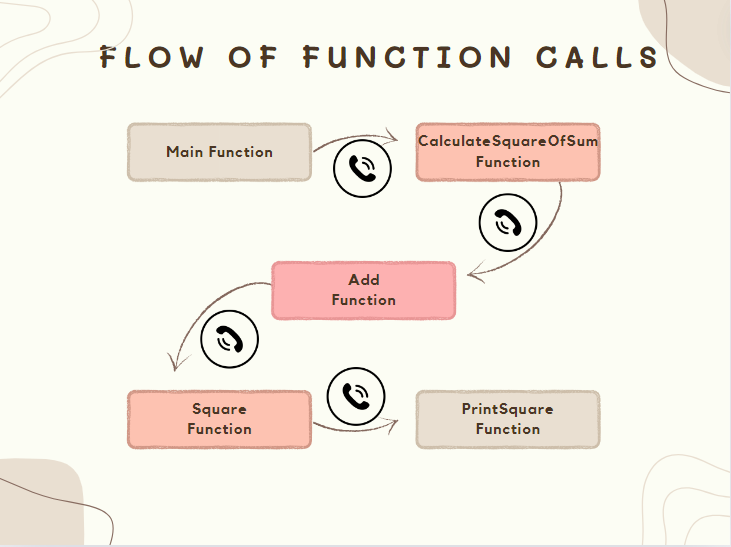
Function Call Stack
Now let's see the function call stack:
Function call stack is a dynamic data structure where elements are stored at contiguous memory locations. Function call stack is maintained for every function call where it contains its own local variables and parameters of the callee function.
Note: We will learn about Stack data structure later in our DSA course also in detail.Function call stack also works in a similar method as that of Stack. Stack is a data structure which follows Last-In-First-Out (LIFO) mechanism. (Eg. The one who enters a classroom at last will be the first one to get out of the classroom. )
The function call stack also stores the return address of the function itself.
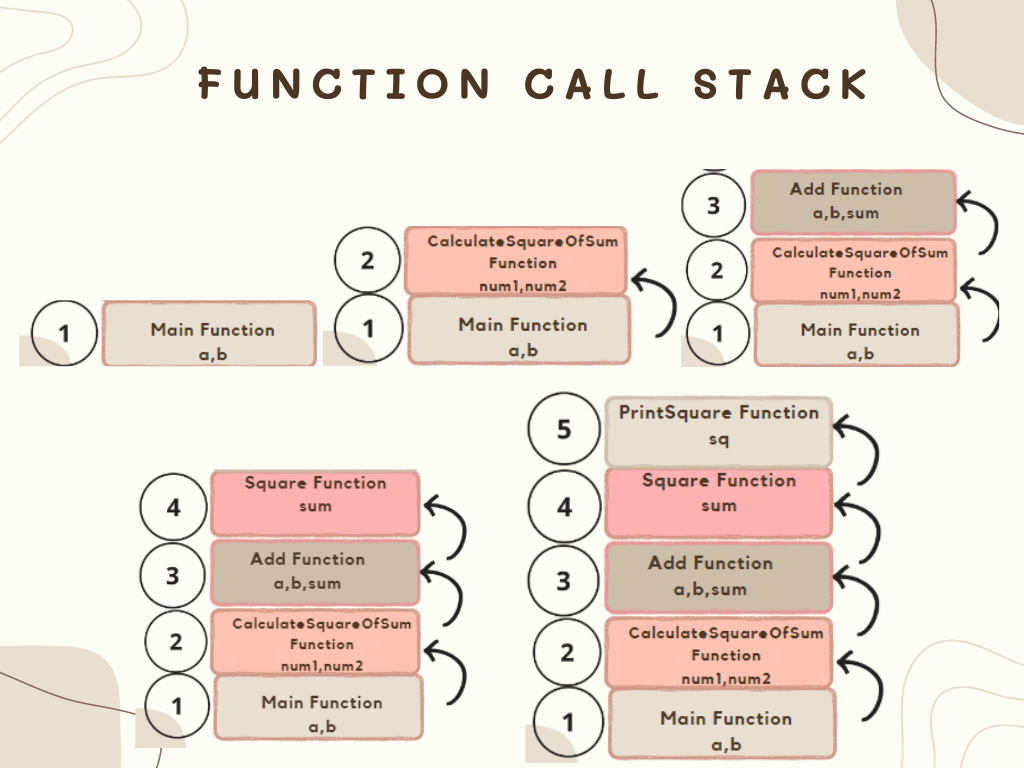
The flow of function calls discussed above is represented in the form of a stack.
Step 1: As the execution of the program starts from the main function, it will be pushed into the stack first. It will store the local variables of the main function i.e. a and b.
step 2: As soon as we reach the CalculateSquareOfSum function inside the main function, it will call the CalculateSquareOfSum function and it will be stored in a stack with its local variables num1 and num2.
Step 3: Now, the CalculateSquareOfSum function contains the Add function. Hence, it will call the Add function and now, the Add function will be pushed in the stack with its parameters and local variable i.e. a,b and sum.
Step 4: Similarly, the Add function will call the Square function and put it into the stack with its variable sum.
Step 5: Finally, the Square function calls the PrintSquare function and push it into the stack and we get the final call stack.
While clearing the stack the order will be reversed which implies the function which is at the top of the function call stack will be popped out from the stack at first.
Clearing Memory of Call Stack
Now, We will see how the memory of call stack will be freed.
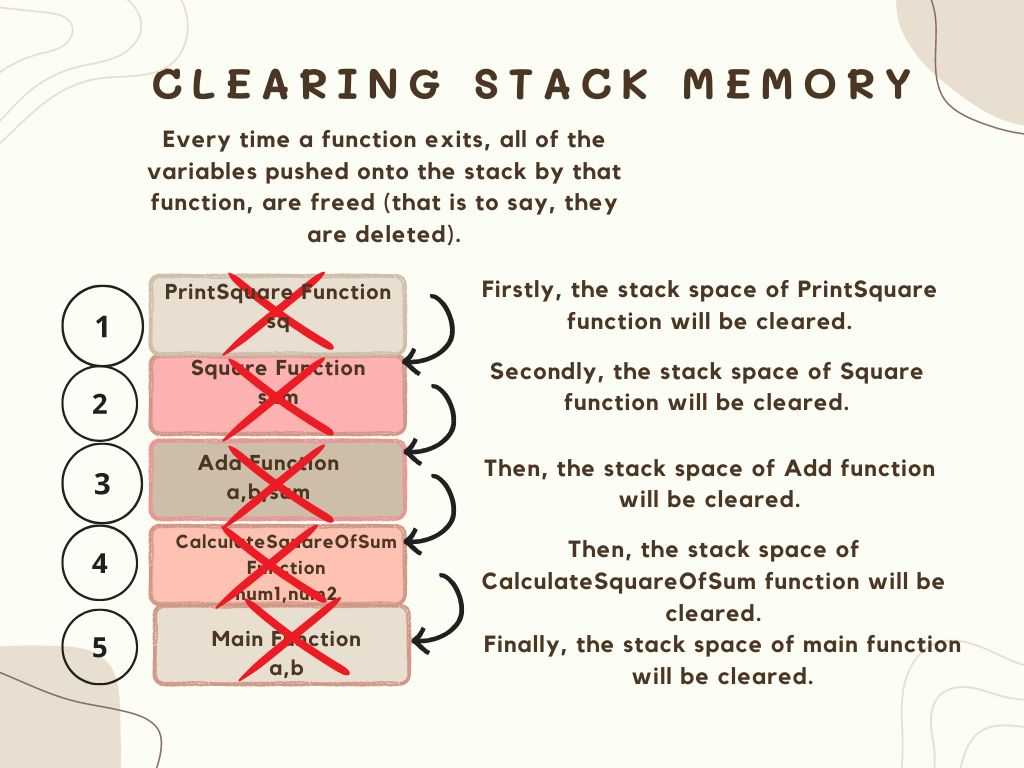
The stack memory will be cleared in the reverse order. ( As, Stack is a data structure that follows Last-In-First-Out (LIFO) mechanism. )
Now, I am assuming that we have understood how one function calls the other function.
Function Calling Itself
We have seen how a function calls another function but what if instead of calling other functions it calls itself?
Let's see this with an example.
#include <iostream>
#include <cmath>
using namespace std;
void Add(int a, int b){
int sum = a + b;
cout << sum << endl;
Add(a,b);
}
int main(){
int a,b;
/* Input numbers from user */
cout << "Enter number 1: ";
cin >> a;
cout << "Enter number 2: ";
cin >> b;
//We have to calculate Square Of Sum Of two Numbers (a+b)^2
Add(a,b);
return 0;
}
Run this code on your system and observe what happens.
The system will keep on printing the sum of numbers you have entered and eventually, it might hang. But, Why?? Make the Function Call Stack and try to think of the answer in terms of stack space memory.
This interesting concept of function calling itself is known as Recursion. We will deep dive into recursion in out DSA course.
See you in the next article with more energy and excitement ✌️ till then Happy Learning !! 😀