Sum of Digits
Given a number num, find the sum of digits of the number.
Examples
Input: 687
Output: 21
Explanation: The sum of digits i.e. 6 + 8 + 7 is equal to 21.
Input: 12
Output: 3
Explanation: The sum of digits i.e. 1 + 2 is equal to 3.
So, if you are given an integer num and you are told to find the sum of the digits of that number.
The simple approach would be to extract the digit of that number and keep adding it to the sum.
But the question is how to extract the digit.
We know we can either extract the digit from the front or the back of a number.
If we want to extract it from the front.
Let’s understand it with an example.
Let's take the number 9742, we can write it as Nine Thousand four hundred forty-two.
So to extract the digit in the thousands place we can perform operations like 9742/1000=9, to extract the digit in the hundreds place we can perform operations like (9742 - 9*1000) / 100 = 7, to extract the digit in the tens place we can perform like (9742 - 9*1000 - 7*100) / 10 = 4, and finally to extract the digit in one's place we can perform operations like (9742 - 9*1000 - 7*100 - 2*10) = 4.
So we can see that extracting from the front side is complex. Also, the one's place starts from the back (i.e. from the right-hand side).
So let's try extracting from the back side.
i.e. starting from the right it comes to ones -> tens -> hundred -> thousand and so on. So the idea is to extract the digits from the back side(right-hand side) and keep adding it to our answer.
Let's take an example for number 15, if we want to find the digit in the one's place of this number what should we do here?
We know that the given number is in base 10, so somehow if we get the remainder of the number when divided by 10, we will get the digit in the ones place of that number. Now there is an operator that makes our work easy here i.e. modulo(%) operator.
The modulo (%) Operator is used to find the remainder.
Let's take a question: when A = 15 is divided by B = 10 what is the remainder?
We can simply find it using A%B i.e. it will give us the remainder when 15 is divided by 10.
So the remainder is 5.
In general, In the decimal number system to get the :
Last digit: we modulo it with 10^1 = 10 (remainder lies from 0 to 9).
Last two digits we modulo it with 10^2 = 100 (remainder lies from 0 to 99).
Last three digione's99).
Last n digit we modulo it with 10^n = 1000….n times (remainder lies from 0 to 10^n - 1).
But here in this question, we only need a single digit at a time and add that digit to our answer to get the answer. But the question is after adding ones digit to our answer what should we do to add the tens place digit to our answer?
To add the tens place digit to our answer we have to first divide the current number by 10, to get the tens place shifted to the one's place and then extract that particular digit in one's place, and this process will continue till our number is greater than 0.
Let's understand it with an example for num = 12345
Initially num = 12345 and sum=0.
num is in the decimal number system (Base 10).
At the first iteration, if we divide the number by 10 we get the quotient as 1234 and the remainder as 5 (last digit of 12345).
Our num becomes 1234.
sum = 0 + 5.
Now at the second iteration, if we divide the number by 10 we get the quotient as 123 and the remainder as 4 (last digit of 1234).
Our num becomes 123.
sum = 0 + 5 + 4.
Now at the third iteration, if we divide the number by 10 we get the quotient as 12 and the remainder as 3 (last digit of 123).
Our num becomes 12.
sum = 0 + 5 + 4 + 3.
Now at the fourth iteration, if we divide the number by 10 we get the quotient as 1 and the remainder as 2 (last digit of 12).
Our num becomes 1.
sum = 0 + 5 + 4 + 3 + 2.
Now at the fifth iteration, if we divide the number by 10 we get the quotient as 0 and the remainder as 1 (last digit of 1).
Our num becomes 0.
sum = 0 + 5 + 4 + 3 + 2 + 1.
Now since our num has become 0, dividing it with 10 will keep giving us a remainder 0 for infinite times, so there is no sense of dividing it anymore and getting the remainder.
So our loop should terminate, Exit.
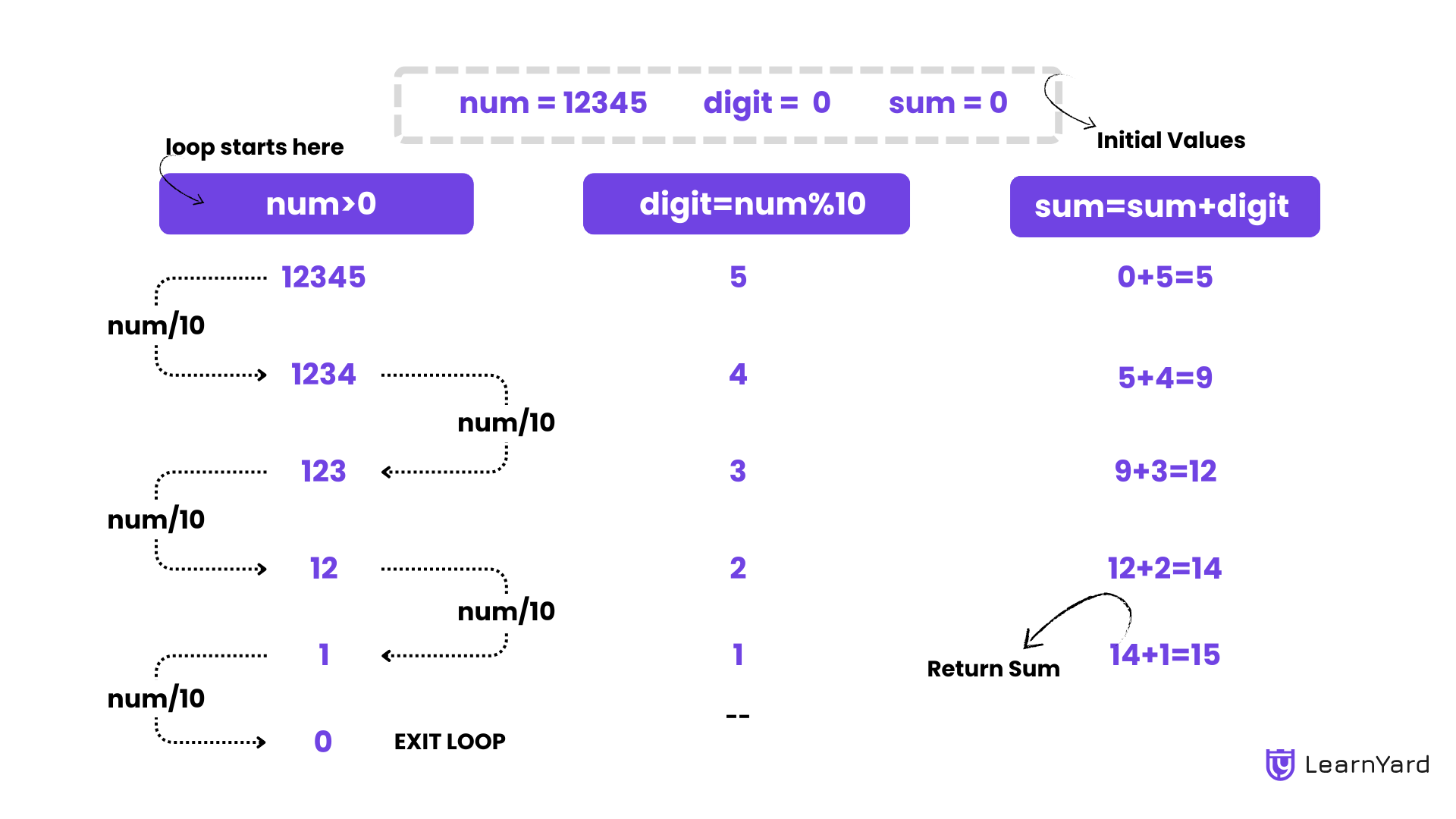
Code Implementation
C++ Code Try on Compiler!
int sumOfDigits(int num) {
int sum = 0;
while (num > 0) {
// Extract the last digit
int digit = num % 10;
// Add the digit to the sum
sum = sum + digit;
// Remove the last digit
num =num / 10;
}
return sum;
}
Java Code Try on Compiler!
class Solution {
public static int sumOfDigits(int num) {
int sum = 0;
while (num > 0) {
// Extract the last digit
int digit = num % 10;
// Add the digit to the sum
sum += digit;
// Remove the last digit
num /= 10;
}
return sum;
}
}
Python Code Try on Compiler!
def sum_of_digits(num):
sum = 0
while num > 0:
# Extract the last digit
digit = num % 10
# Add the digit to the sum
sum += digit
# Remove the last digit
num //= 10
return sum
Javascript Code Try on Compiler!
function sumOfDigits(num) {
let sum = 0;
while (num > 0) {
// Extract the last digit
let digit = num % 10;
// Add the digit to the sum
sum += digit;
// Remove the last digit
num = Math.floor(num / 10);
}
return sum;
}
Time Complexity : O(logn)
The time complexity arises from the number of digits in the number. The number of times the while loop runs depends on the number of digits in the given number num.
For a given number num in a decimal representation, the number of digits is approximately equal to log10(n). This is because each digit represents a power of 10.
The loop executes once for each digit in num.
Therefore, the loop runs log10(num) times, simplifying to O(logn).
Space Complexity : O(1)
We are using only the sum variable, which uses constant space.
Since the space required by these variables does not depend on the size of the input number num, the space complexity is O(1).
Now we have learned how to extract digits from a number, so let's solve another problem: reverse a number using the concept we have learned before.