Comments
Let's say you worked hard and your sleepless nights brought you to Google but initially you have to go through the previous code base that has been written by other programmers. So to understand those million lines of code some description or important information is attached to understand the functionality of a specific block of code.
These important and self-explanatory blocks of information are called as comments.
What are comments?
While programming we require some blocks of code that the computer should not run and just ignore. These blocks are created by using comments.
We introduce comments by inserting two forward slashes //
.
Let's see an example to demonstrate it.
#include <iostream>
using namespace std;
int main(){
// This is a comment
cout << "I am loving Learn Yards ";
return 0;
}
Output :
I am loving Learn Yards
As you can notice, the "This is a comment" line is not being executed by the compiler and is just ignored.
You should definitely have one question : If it is not being read and executed by the computer , WHY are we using it ?
Comments are very useful for programmers, it is being used to add some important information or to annotate code for future reference. It is more like documenting your work in the code itself for the readability of the code for other fellow programmers.
Types of Comments
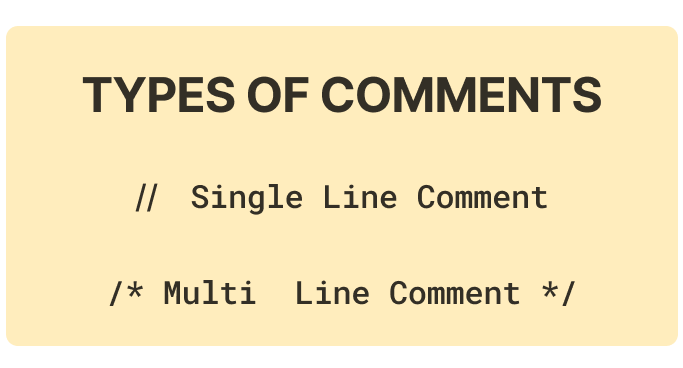
There are two types of Comments in C++:
1 . Single-Line Comment
As the name suggests, it is used to add comments on a single line. It is being added using //
.
#include <iostream>
using namespace std;
int main(){
// prints a string
cout << "Marks " ;
// prints a number
cout << 78 ;
return 0;
}
Output :
Marks 78
// prints a string
// prints a number
Both are single-line comments which are not being executed by the compiler.
2 . Multi-Line Comment
A multi-line comment occupies multiple lines of comments, it starts with /* and ends with */, but it cannot be nested.
Syntax
/*
Multiline Comment
.
.
.
.
.
.
*/
Let's see with an example, how we can add multi-line comments in our code.
#include <iostream>
using namespace std;
int main(){
/* This is a code
to print the marks scored by
a student
*/
cout << "Marks " ;
cout << 65 ;
return 0;
}
Output :
Marks 65
Now let's predict the output of the code below :
#include <iostream>
using namespace std;
int main(){
// This is a code
to print the marks scored by
a student
cout << "Marks" ;
cout << 65 ;
return 0;
}
Guess the output
ERROR
This error occurred because we tried to add a multi-line comment using a //
which is used to take a single line as a comment because of which these two lines are read by the compiler as a code, hence throwing an error.
"to print the marks scored by
a student"
Let's try another one.
#include <iostream>
using namespace std;
int main(){
/*
This is a comment
/*
This is a comment inside a comment
*/
*/
cout << "Hey, there!";
return 0;
}
Guess the Output
It was a trick question. Actually, nested comments are not supported in C++. How this thing works is that as soon as the compiler finds /*, it starts looking for */, and as soon as it finds it, everything that follows is treated is code only, and not a comment.So, in the above case, the second */ is actually treated as code only, because the compiler already found one */ above, which results in an ERROR.
Quiz
#include <iostream>
using namespace std;
int main(){
cout << comments ; // print the output
return 0;
}
#include <iostream>
using namespace std;
int main(){
// cout << "Hello World";
cout << 10;
return 0;
}
Code without comments is like a book without a title; it leaves you guessing and lost in its pages.