Plain Old Basics
Introduction
What would you do if you were asked to write a program to take input of marks of Ayush, Rohan and Vinay and then print the average marks?
I'd probably create 3 variables, take input, and print the average.
Something like this!
#include<bits/stdc++.h>
using namespace std;
int main()
{
int m1, m2, m3; cin >> m1 >> m2 >> m3;
double avg = (m1 + m2 + m3)/3.0;
cout << avg << '\n';
return 0;
}
What if the number of students increased from 3 to the whole class? I don't know about you, but my college batch had ~75 students. There is no way my lazy ass creates 75 different variables 😛
Fortunately, this is exactly the problem that Arrays solve.
An array is like a list of items, but each has a specific place. It's similar to lining up a bunch of boxes in a row. You can put things in these boxes (items) and easily find a specific box by knowing its position in the row (index). This helps you organize and work with lots of items efficiently.
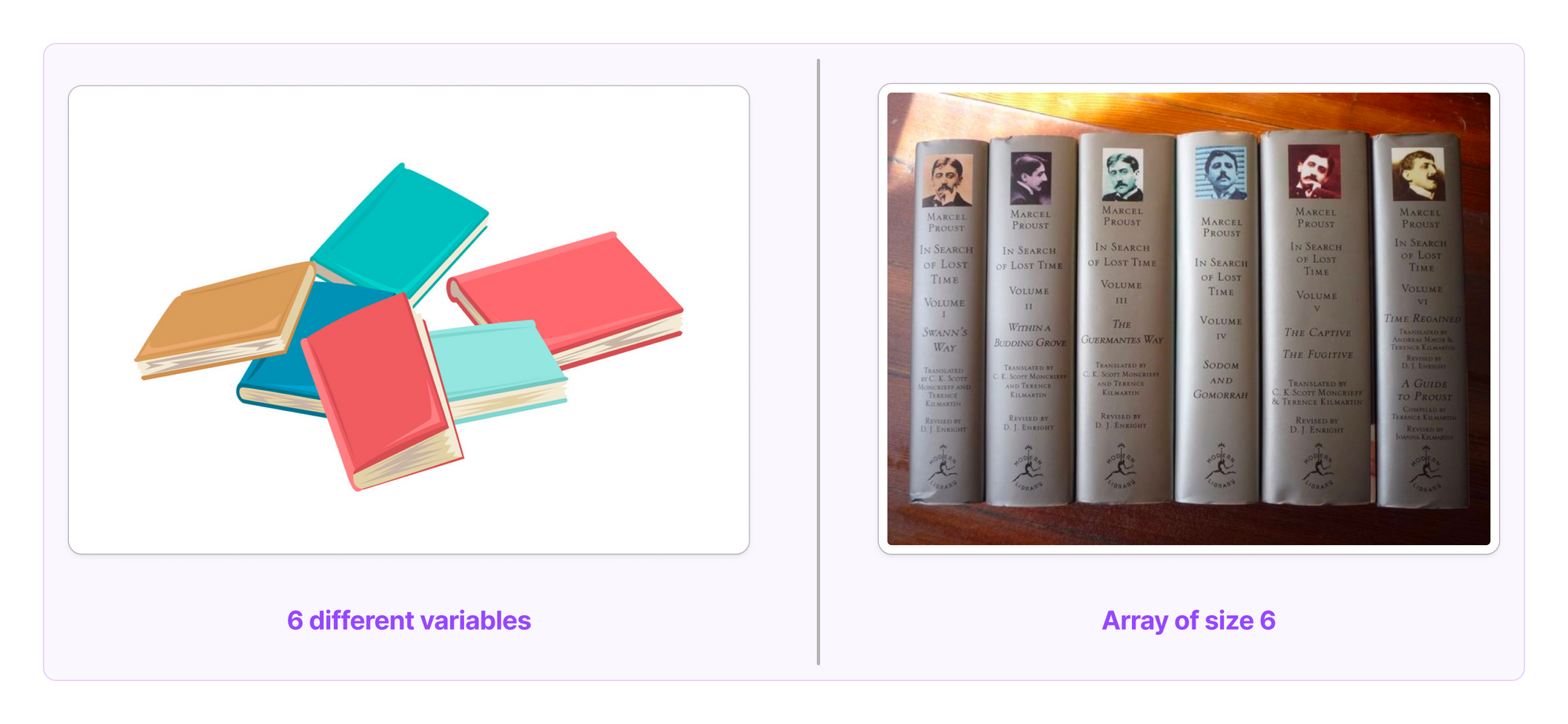
There are a few languages (like Python) where the items can be of any type, but specifically in C++, an array can contain items of the same data type only.
Creating an Array
Declaring
The following code explains one of the ways to declare an array in C++:
// The skeleton:
// data-type array-name[array-size];
// Examples:
int marks[5];
char grade[5];
string name[20], address[20];
// Example for variable sized array:
int n; cin >> n;
int arr[n];
When we declare an array inside a function and don't initialise it with anything, the values at different indices are random for many data types (e.g. int, double, char etc.) and empty for a few others (e.g. string). The random values are termed garbage values.
Note: In C++, it's not feasible to change the size of the array, once created. Sure, you can create a new array of desired size and copy the elements, but resizing the existing array isn't possible.
Initialising
In a normal way, the following is the way to do it where you enclose the values in {}. Eg.
int arr[5] = {1, 2, 3, 4, 5};
What if you enter fewer values than the array_size in {}?
int arr[5] = {1, 2, 3};
int arr[5] = {};
In the cases above, the elements aren't assigned garbage values; the values are 0.
FAQs
So, the above is all about initialisation. Following are a few questions you may have:
What if I enter more values inside {} than the corresponding size?
You'd most likely get a compilation error.What if I enter a string in an integer array?
Again, a compilation error.What if I declare an array first int arr[3]; and then initialise it later on: arr = {1, 2, 3}?
No point for guessing, compilation error again!Accessing the array elements
If we create an array of size N, then the positions of the elements in the array are 0, 1, 2, and so on ... N-1. The positions are called "indices" (singular being "index"). In other words:
- The 1st element is present at index 0.
- The 2nd element is present at index 1.
- ... The pattern goes on ...
- The (N-1)th element at index N-2.
- Finally, the Nth element at index N-1.
How to access the value at index i?
The way to access the ith element of an array having the name arr is arr[i] (where [] is called the subscript operator). Let me explain this using different use cases.
Imagine we have an integer array arr of size 10.
Taking input of all the elements
for(int i = 0; i < 10; ++i)
cin >> arr[i];
Printing elements
// Let's say we want to divide the elements by 2 until they're even,
// and then print the odd leftover.
for(int i = 0; i < 10; ++i) {
while(!(arr[i]%2))
arr[i] /= 2;
cout << arr[i] << ' ';
}
FAQs
What other things can I do with arr[i]?
Let's say arr is an integer array. You can treat arr[i] as if it were an integer variable only.What if someone tries to access a negative index? (eg. arr[-1])
It's not a very uncommon mistake and has personally happened to me as well. In such a case, you don't get a compilation error; the behaviour is undefined at runtime. It could:- Either lead to a runtime error.
- Or the value could be a random garbage value.