Deep-Dive into Memory Allocation
Introduction
Almost all of the runtime data of a C++ program is stored in RAM.
More specifically, if I talk about arrays that we declare/initialise using the way we just learned, and if we do it inside a function, their data is stored in something called the stack memory (a part of RAM).
Let's imagine memory as a series of storage slots or cells arranged linearly, where each slot holds a fixed amount of data (depending on the machine's architecture), and they are all sequentially connected.
The special thing is that when an array is allocated in memory, it occupies a consecutive set of these slots, one after the other. The first slot's memory address is the starting point of the array, and each subsequent slot can be accessed by incrementing the memory address. I'm hoping that this is something you'd have learnt a little bit about while studying pointers.
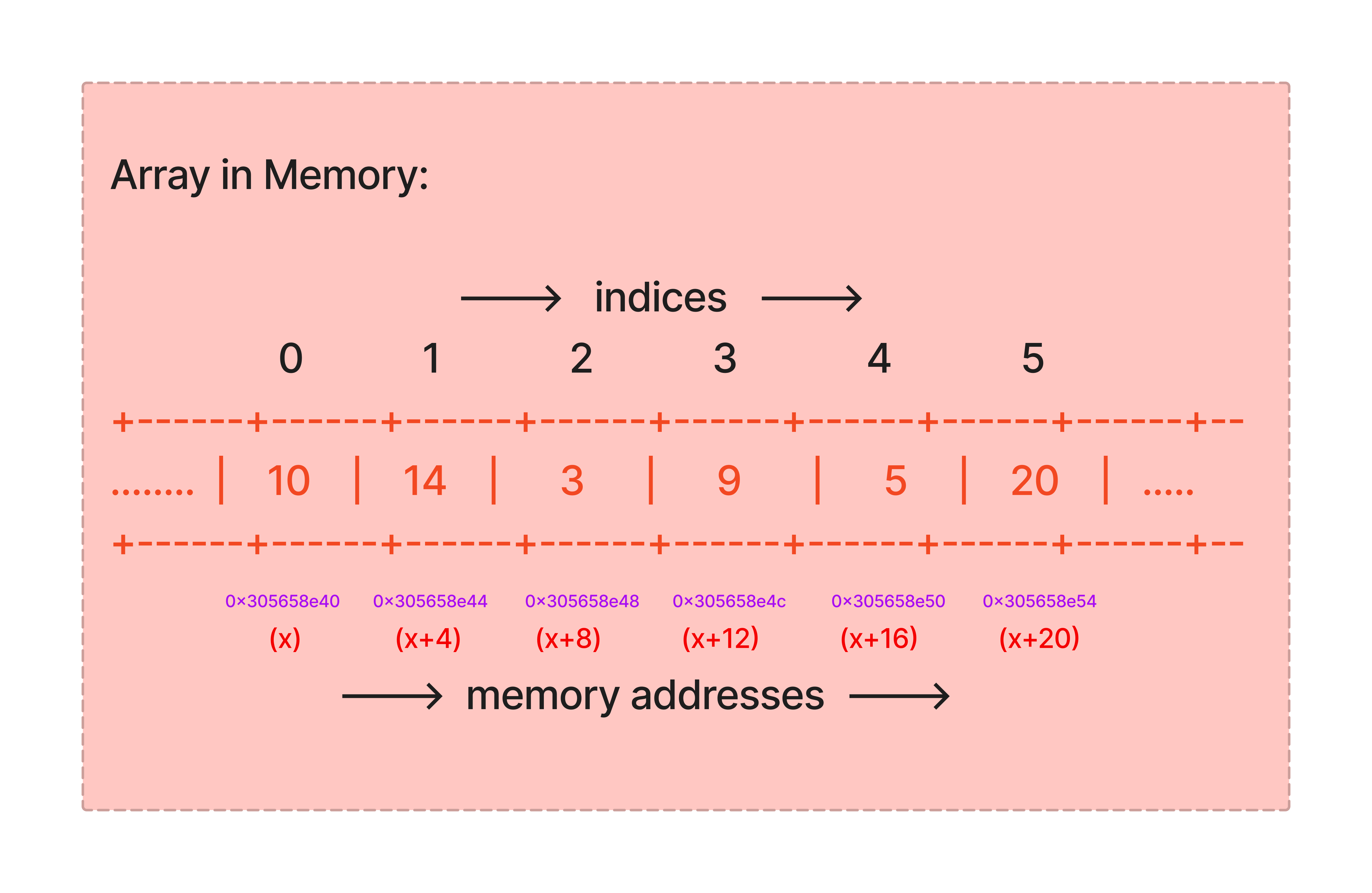
Playing around with pointers
Now, we already saw that printing arr[i] gives the value of the ith element for an array of integers.
From the FAQs of the previous article, you'd know that printing arr itself gives the memory address of the starting point of the array.
int arr[20] = {10, 14, 3, 9, 5, 20};
cout << arr;
Output
0x3061eae50
Also, you'll notice that if you print (arr+i) now, 4*i will be added to the hexadecimal number printed above, and the resultant will be printed. This is because an integer has a size of 4 bytes. If it were an array of long long, then the increment would've been 8 bytes.
cout << (arr+1) << '\n';
cout << (arr+2) << '\n';
cout << (arr+3) << '\n';
cout << *(arr+3) << '\n';
Output
0x3061eae54
0x3061eae58
0x3061eae5c
9
Notice the last 2 digits in the 1st three lines: after 50 from the previous output, 54 got printed, then 58 and then 5c (where c = 12).
Then, because the de-reference operator (*) is used in the last line, 3 got printed, which is the value of arr[3].
When we access the elements using arr[i], that also internally does *(arr + i) only to get the value, which leads me to share an interesting fact:
If you try to access ith element of an array using i[arr] instead of arr[i], that should also work.
Let's try.
for(int i = 0; i < 5; ++i)
cout << i[arr] << ' ';
cout << '\n' << 5[arr];
Output
10 14 3 9 5
0
Didn't believe me before actually seeing it? xD
Let's end it here with an interesting question.