π My Sense Frontend Engineer Interview Experience (2025) | Senior UI Developer
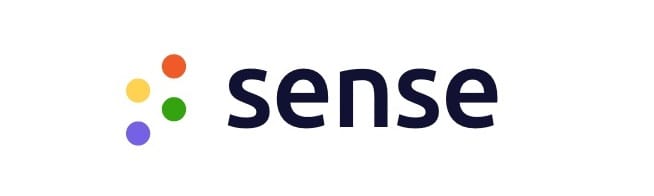
Frontend interviews at top product-based companies test far more than your UI skillsβthey assess your problem-solving ability, architectural thinking, and code clarity. I recently interviewed at Sense, and this article breaks down my complete journeyβDSA questions, advanced JavaScript challenges, system design discussions, and hands-on machine coding.
If you're preparing for frontend roles at startups or FAANG-level companies, this is your blueprint. Letβs dive in! π
π§© Interview Process Overview
The Sense interview process spanned 5 rounds, focusing on:
- Data Structures & Algorithms (DSA)
- Advanced JavaScript + Frontend Concepts
- System Design for Scalable UI
- Machine Coding (React Component Building)
- Behavioral & Hiring Manager Round
π‘ Round 1: Data Structures & Algorithms
π§ͺ Binary Search β Recursive Implementation
Task: Implement Binary Search in JavaScript from scratch.
// π Binary Search using Recursion in JavaScript
function binarySearch(list = [], targetElement = 0) {
// π Step 1: Get the length of the current list
let listLength = list.length;
// π― Step 2: Calculate the middle index
let middleIndex = Math.floor(listLength / 2);
// β
Step 3: Check if the middle element matches the target
if (list[middleIndex] === targetElement) return true;
// β Step 4: If the list has only one element and it's not the target
if (list.length === 1) return false;
// π Step 5: Recursively search in the left or right half of the list
return list[middleIndex] > targetElement
? binarySearch(list.slice(0, middleIndex), targetElement) // π Search in the left half
: binarySearch(list.slice(middleIndex), targetElement); // π Search in the right half
}
// π Sample sorted list to test the function
let sortedList = [1, 2, 3, 4, 5, 15, 20, 25, 100];
// π§ͺ Test Cases
console.log(binarySearch(sortedList, 21)); // β false - not found
console.log(binarySearch(sortedList, 20)); // β
true - found
Problem Statement
π Notes:
- This is a recursive implementation of binary search.
- It's based on divide-and-conquer, repeatedly halving the array.
- Complexity: O(log n) time for sorted arrays.
list.slice(...)
creates a new array in each recursion, which uses extra memory. A more memory-efficient version would use start and end indices instead.
β Other DSA Questions:
- π Shallow vs Deep Cloning β With custom deep clone implementation
- π Handling Circular References in Object Deep Copy Logic
- π§
object.keyName
vsobject[keyName]
β JavaScript key access patterns
π₯ Pro Tip: Focus on recursion, hash maps, array/object manipulation, and edge cases. Practice on LeetCode, JavaScript.info, and GeeksforGeeks.
π‘ Round 2: Advanced JavaScript
1οΈβ£ SSR vs CSR β Which is better for frontend performance?
β Server-Side Rendering (SSR) advantages:
- SEO-friendly out of the box (better crawlability)
- Faster initial page load (especially on slow networks)
- Better Core Web Vitals β improved LCP (Largest Contentful Paint)
2οΈβ£ Implement flow()
like Lodash
Task: Chain functions together and process input sequentially.
// π flow: Composes multiple functions from left to right
const flow = (...funcs) => (arg) =>
funcs.reduce((res, fn) => fn(res), arg);
// π§ Explanation:
// - Accepts multiple functions as input (...funcs)
// - Returns a function that takes a starting argument (arg)
// - Uses reduce to pass the result of each function to the next
// β Function 1: Adds 2 to input
const add = (x) => x + 2;
// βοΈ Function 2: Multiplies input by 3
const multiply = (x) => x * 3;
// π§ Compose functions: First add, then multiply
const process = flow(add, multiply);
// π§ͺ Test the composed function
console.log(process(5)); // Output: (5 + 2) * 3 = 21 β
Problem Statement
β Output Explanation:
add(5)
β‘οΈ7
multiply(7)
β‘οΈ21
- So
process(5)
gives21
.
π Why Use flow
?
- It's a cleaner way to compose multiple functions.
- Similar to
lodash.flow
orRamda.pipe
. - Encourages functional programming in JavaScript.
3οΈβ£ Debounce Polyfill + call
, apply
, bind
usage
π§ Expect to explain your approach, closure usage, and execution throttling logic.
π‘ Round 3: System Design
1οΈβ£ A/B Testing β How Does It Work in the Frontend?
π§ͺ Core Concepts:
- Split users into control & variant groups
- Use feature flags to toggle UI dynamically
- Track metrics: CTR, conversion, engagement
π οΈ Tools discussed: Google Optimize, Optimizely, VWO
2οΈβ£ Optimize Large Data Tables (Trillions of Records)
Strategies:
- π Virtualized Rendering with
react-virtualized
orreact-window
- π§ Pagination, Lazy Loading, and infinite scroll
- πΎ Use IndexedDB, localStorage, and intelligent caching
π₯ Performance tuning is critical in enterprise dashboards and analytics UIs.
π‘ Round 4: Machine Coding
π§± Task:
- Build a NavBar with a user list.
- On clicking a user:
- Remove them from the list
- Show their details in a side panel
- Optimize re-renders using React.memo, useCallback
π§ Focus Areas:
- Component reusability & structure
- Optimizing renders with React hooks
- UI state management using
useState
oruseReducer
π The interviewer appreciated a clean folder structure and well-separated logic for state, UI, and event handling.
π‘ Round 5: Hiring Manager
Questions Asked:
- π§ βTell me something about yourself thatβs not in your resume.β
- π¬ βWhy did you choose engineering?β
- π βWhy did you switch jobs every 2 years?β
- π§ βWhat do you expect from this role and company?β
π― Use the STAR Method (Situation, Task, Action, Result) for structured and impactful answers.
π Key Takeaways & Tips
β
DSA & JavaScript Core β Recursion, Closure, Event Loop
β
System Design Knowledge β A/B Testing, Data Handling, Rendering
β
React & Real-World Coding β Build & optimize UI components
β
Behavioral Prep β Be self-aware and communicate your growth story
π― Final Thoughts
The Sense interview process was well-structured and technical. It tested my problem-solving mindset, UI architecture thinking, and hands-on coding ability. If youβre preparing for a modern frontend engineering role, this kind of multi-dimensional preparation is essential.
Hey there from LearnYard! π
Glad to see you exploring the free content from our course! Weβve shared 10 valuable articles at no cost to help you get a real feel of whatβs inside.
If youβve found these helpful, weβd love to know! π¬
Share your thoughts or key takeaways on LinkedIn, Twitter, or Instagram β and donβt forget to tag Gourav Hammad & Mohammad Fraz. Weβll personally respond to your post!
Thanks for being part of the LearnYard journey. Keep learning, keep growing! π
Loved the free content? Want access to the full course β for free? πΈ
Hereβs how: share the course with your friends using your affiliate link (youβll find it on your profile or home page), and earn 20% commission on each sale.
Just 4β5 sales and youβll recover the full course price of βΉ999/- π
So why wait? Start sharing, start earning, and unlock the rest of the course effortlessly! π οΈπ
π‘ Tips to Crack the Sense Frontend Interview
β
Understand the Interview Format:
Sense evaluates candidates holistically β from JavaScript fundamentals to large-scale frontend architecture. Prepare accordingly.
β
Master Core JavaScript Concepts:
Closures, event loop, hoisting, scope chain, and asynchronous patterns are must-know topics.
β
Practice DSA in JavaScript:
Use platforms like LeetCode or GeeksforGeeks. Focus on recursion, string/array manipulation, binary search, and object-based problems.
β
Build Real-World Components:
Projects like modals, dropdowns, carousels, infinite scrolls, and dynamic sidebars show strong problem-solving skills.
β
Sharpen System Design Knowledge:
Learn how to design scalable, performant frontend apps. Understand concepts like SSR, CSR, CDN caching, and lazy loading.
β
Mock Interviews & Pair Programming:
Simulate interviews with peers or mentors to get real-time feedback on communication, clarity, and code structure.
β
Ace the Behavioral Round with STAR:
Prepare thoughtful answers using the STAR method β Situation, Task, Action, Result β for questions around team leadership, decision-making, and cultural fit.
π Recommended Topics to Prepare
π§ JavaScript & Browser Fundamentals
- Hoisting, Scope, Closures, Currying
- Event Loop, Microtasks vs Macrotasks
- Memory Management & Garbage Collection
- Call, Apply, Bind
- Debounce & Throttle
- Polyfills (e.g., for
map
,bind
)
βοΈ React & Frontend Frameworks
- Functional Components & Hooks
- Context API, useReducer, useMemo
- React.memo, useCallback
- Virtual DOM & Reconciliation
- Code Splitting, Lazy Loading
π¦ Frontend Architecture & System Design
- SSR vs CSR
- Component Communication Patterns
- Feature Flags & A/B Testing
- Optimizing Rendering (e.g., React Virtualized)
- Error Boundaries, Suspense, SuspenseList
π§ͺ Testing & Tooling
- Unit Testing with Jest, React Testing Library
- Browser DevTools & Lighthouse Audits
- Webpack, Vite, and Build Optimizations
π§© Machine Coding Patterns
- State Management Techniques
- Side Panel, List Filtering, Modal UI Logic
- Performance Optimization for Dynamic UIs
π’ Hashtags for Visibility
#FrontendInterview #JavaScriptInterview #ReactJS #SystemDesign #WebDevelop #MachineCoding #TechInterviews #SenseAI #LeadFrontendEngineer #DSAinJavaScript #InterviewTips #SoftwareEngineerLife #TechHiring #CodeNewbie #FrontendMasters #JSInterviewPrep #MachineCodingRound #LearnYard