Types of Array
There are two types of array.
- Single Dimensional Array
- Multidimensional Array
Single Dimensional Array
Contains only one level or dimension. It's like a simple list having a row of boxes where you can store and retrieve elements using their position (index) in the row.
Syntax
int[] numbers = new int[]{15, 20, 7, 10, 9, 19, 45};

We have learned about single-dimensional arrays in detail in the last chapter.
Multidimensional Array
Contains multiple levels or dimensions. It's like having lists within lists, forming a grid or table.
In such case, data is stored in row and column based index known as matrix form.
Here's how the 2-D array will look like -
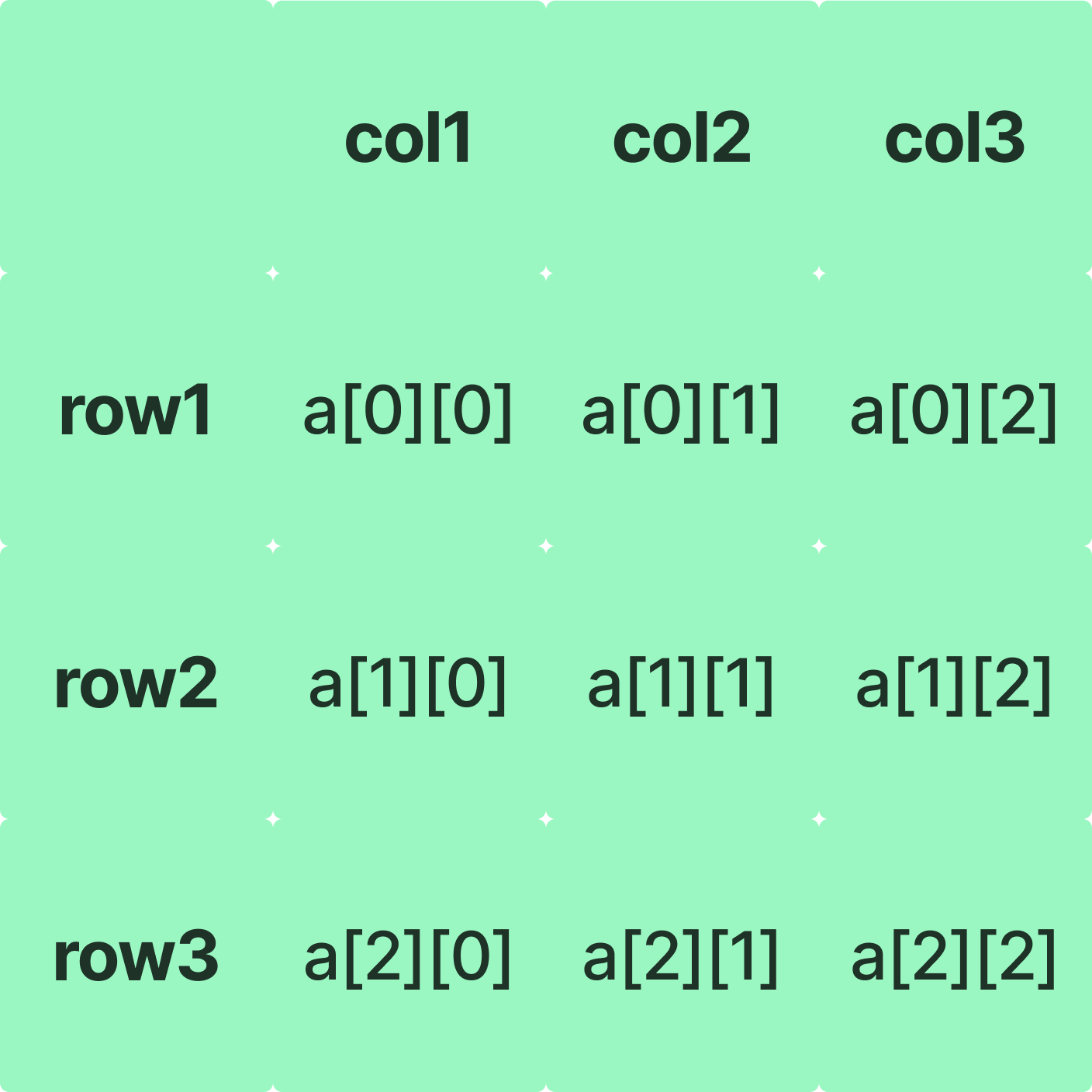
Syntax to Declare Multidimensional Array in Java -
You can declare a multidimensional array using various syntaxes:
dataType[][] arr; OR
dataType [][]arr; OR
dataType arr[][]; OR
dataType []arr[];
These different forms are equivalent and valid ways to declare a multidimensional array in Java.
Example to Instantiate Multidimensional Array in Java:
To create a multidimensional array, you can use the following syntax:
dataType[][] arrayName = new dataType[rows][columns];
For instance:
int[][] arr = new int[3][3]; // Creates a 3x3 integer array
Example to Initialise Multidimensional Array in Java:
Here is how we can initialise a 2-dimensional array in Java.
int[][] arr = {
{1, 2, 3},
{4, 5, 6, 9},
{7},
};
Another way to initialise multidimensional array is after creating the array, you can set values for each element:
arr[0][0] = 1;
arr[0][1] = 2;
arr[0][2] = 3;
arr[1][0] = 4;
arr[1][1] = 5;
arr[1][2] = 6;
arr[2][0] = 7;
arr[2][1] = 8;
arr[2][2] = 9;
These assignments initialise the elements of the array with specific values, creating a 3x3 matrix of integers.
Let's explore another example of a multidimensional array, this time with three dimensions. Consider the following declaration:
int[][][] data = new int[3][4][2];
In this example, data
is a three-dimensional array capable of holding a maximum of 24 elements (calculated as 3 * 4 * 2), and each element is of type int
.
How to find an array length?
In Java, every array comes with a built-in property called "length" that tells you how many elements it can hold. This is like knowing the total capacity of the array. You can find the length by using the array name followed by a dot (.) and then the word "length."
For example, if you have an array of integers named arr
that can store 5 elements, you can find its length like this:
int[] arr = new int[5];
int arrayLength = arr.length;
Here, arrayLength
is a variable that now holds the length (or capacity) of the array.
It's important to note that the "length" property indicates the maximum number of elements the array can contain, not how many elements are currently in it. If you initialise the elements when you create the array, the length will be the same as the number of initialised elements.
When we talk about the logical size or the index of an array, we need to consider that array indices start from 0. So, if you want to find the logical size, which is the highest index in the array, you can use int arrayLength = arr.length - 1
.
Here's why: since array indices start at 0, the highest index is always one less than the actual size of the array. So, subtracting 1 from the length gives us the highest index or the logical size of the array.
Example
class Main {
public static void main(String[] args) {
// create a 2D array
int[][] a = {
{1, 2, 3},
{4, 5, 6, 7},
{8, 9},
};
// calculate and display the length of each row
for (int i = 0; i < a.length; i++) {
System.out.println("row " + (i + 1) + "length : " + a[i].length);
}
}
}
Output
row 1 length : 3
row 2 length : 4
row 3 length : 2