do....while Loop
The Java do-while loop is similar to what 'while' and 'for' loop that lets you do something in your program over and over again until a certain condition is met. It's useful when you're not sure how many times you want to do it, but you want to make sure it happens at least once.
Think of it as a "try it first, check later" loop. It's a bit different from other loops because it checks the condition after doing the task. It's a friendly loop that guarantees you won't miss out on doing something important in your program.
Imagine you're playing a game, and you want to take a turn at least once. The do-while loop is like saying, "Take a turn, and keep doing it until you win." It ensures you get to play at least once.
It's syntax is as follows:
do {
//code to be executed
} while(condition);
The do-while loop executes the code first time irrespective of condition met or not and then checks the condition. If it's true, it executes the code over and over again until the condition is no longer true.
Here the interesting part is that it checks that condition over and over again. If it's still true, it keeps doing that thing.
FlowChart
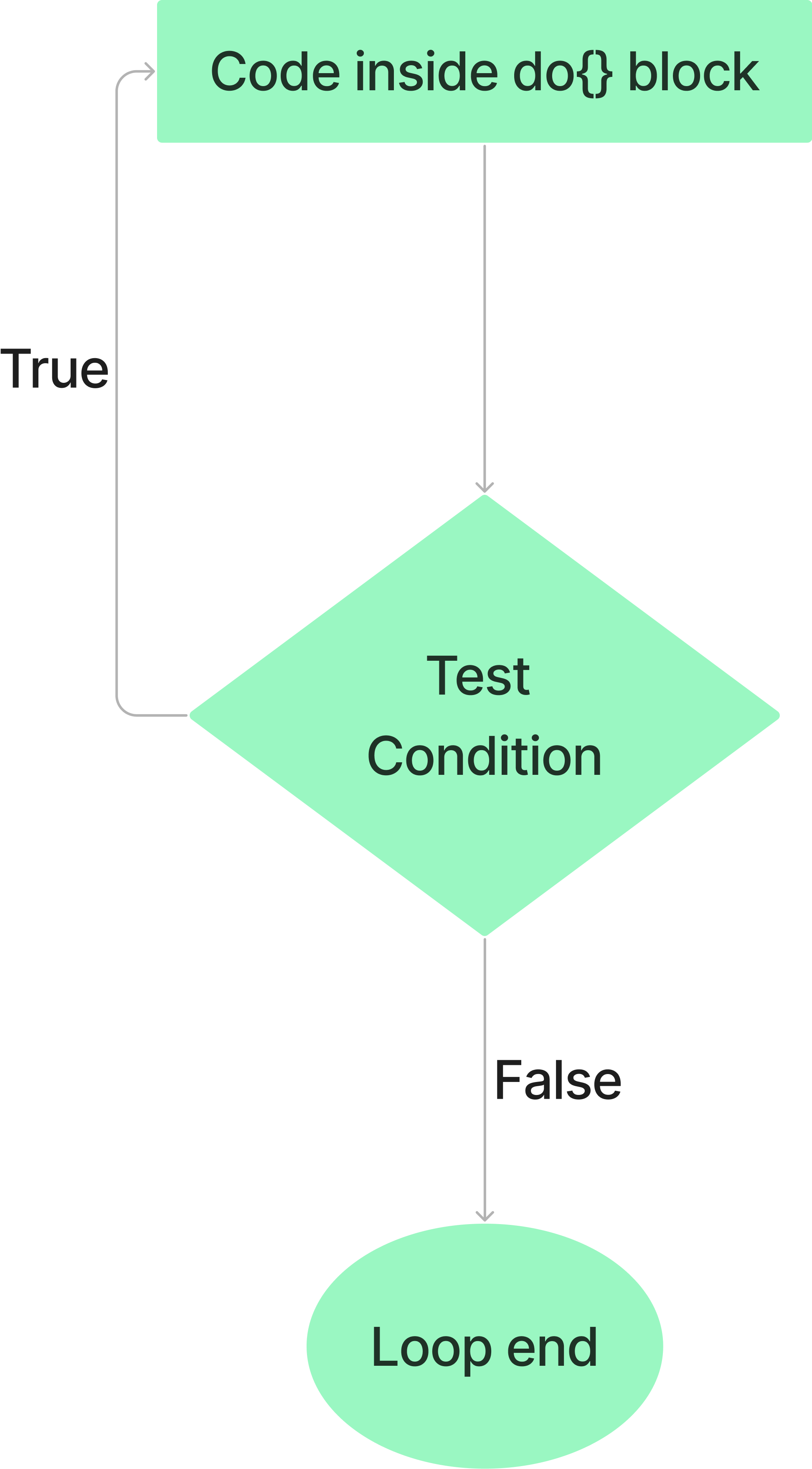
Example1
class Main {
public static void main(String[] args) {
int numberOfTimes = 1;
do {
System.out.println("Code inside do block is running.");
numberOfTimes++;
} while(numberOfTimes <= 3);
}
}
Output
Code inside do block loop is running.
Code inside do block loop is running.
Code inside do block loop is running.
- The do-while loop:
do { ... } while(numberOfTimes <= 3);
- It says: "Keep doing what's inside the curly braces as long as
numberOfTimes
is less than or equal to 3."
- Prints code inside the loop:
System.out.println("Code inside do block is running.");
- This line prints a message saying that the "Code inside the do block is running".
- Updating the counter:
numberOfTimes++;
- After running the code inside the loop, we increase the value ofnumberOfTimes
by 1.
- Loop condition:
while(numberOfTimes <= 3);
- The loop checks ifnumberOfTimes
is still less than or equal to 3. If it is, the loop continues. If not, it stops.
So, in this example, the message inside the loop will be printed three times because we set up the loop to run as long as numberOfTimes
is less than or equal to 3.
Example2
class Main {
public static void main(String[] args) {
int numberOfTimes = 4;
do {
System.out.println("Code inside do block loop is running.");
numberOfTimes++;
} while(numberOfTimes <= 3);
}
}
In this example, the message inside the loop will be printed only once because numberOfTimes
is already greater than 3 so while condition is not satisfied.
An infinite do-while loop Example
class Main {
public static void main(String[] args) {
// code inside do-while loop will run infinite times.
do {
System.out.println("Code inside do block loop is running.");
} while(true);
}
}
Output
Code inside do block loop is running.
Code inside do block loop is running.
Code inside do block loop is running.
Code inside do block loop is running.
Code inside do block loop is running.
Code inside do block loop is running.
Code inside do block loop is running.
.....
In this example, the message inside the loop will be printed infinite times since condition inside while loop is always true.