Intoduction to Functions
Overview
Suppose you go to a restaurant, they serve you main course, juices, ice cream, fruits, water, tissue everything in a single bowl. Would you like that?? I guess it's a strong "No". We want things to be organized i.e. every dish should be in a proper and separate utensil according the function it serves. For example: Water in a glass, Rice in plate, curry in a bowl etc.
In this article, we will learn about functions that help us in organising our code.
As our programs get more complex, the code can start to look messy and become difficult to understand. Functions allow us to break our program into smaller, more manageable pieces, making it much easier to understand and use.
Suppose we are playing an online game where we have a program for managing our games. Instead of doing everything at once, functions help us to create specific tools for each job:
- Function to calculate total games played.
- Function to calculate total games won.
- Function to remind us to go back to study. (If we have turned on Focus mode XD)
This way, we can solve one part of the problem at a time, which reduces the complexity and increases the simplicity of the program.
Function Creation
Now let's see how can we create a function.
Syntax for creating a function
While creating a function, we follow the following structure:
return_type FunctionName() {
// our code will come here
}
1) return_type - This represents the type of data returned by the function (like for integers the return type will be int, for words return type will be string, etc.).
2) FunctionName() - This is the name of our function. (Example: Every part of our body that performs a particular function has a name like the brain, heart, eyes, etc. ).
Some points to keep in mind:
- The function name should describe what the function is doing. It is a good coding practice. For example: If you are writing a function to add numbers, the function name could be AddTwoNumbers.
- Functions should start with a capital letter and have a capital letter for each new word. This is suggested by Google. So, it is a great coding practice and can earn you brownie points in your interviews.
- You can name your function in any way you want but I will suggest following the guidelines.
3) {...} - This describes the body of the function. It basically describes what the function does.
Example
Consider a simple example where we create a program to print a birthday message 5 times. We'll use a function to handle this repetitive task:
void BirthdayGreeting() {
for (int i = 0; i < 5; i++) {
cout<<"Happy Birthday");
}
}
In this example:
- We've created a function named BirthdayGreeting( ).
- We use void return type if the function doesn't return any value.
- Inside the function, it uses a loop to print the message "Happy Birthday" five times.
- Curly braces represent the body of the function.
When we run the program and call the BirthdayGreeting function, it prints the message five times. This illustrates how functions simplify our code and make it modular and readable.
Calling a Function
Let's use the previous example and let's try to execute that function in a program -
#include <iostream>
using namespace std;
void BirthdayGreeting() {
for (int i = 0; i < 5; i++) {
cout<<"Happy Birthday");
}
}
int main() {
return 0;
}
Running the above code won't display any output because creating a function doesn't automatically execute the code that the function body contains.
Imagine your introvert friend who is always ready to help you but only when you call them. The functions are same as these introvert friends, they will perform the action and execute the code within them only when you call them.
The purpose of the code is to offer us a tool (the BirthdayGreeting() function) that we can use when needed. To make the greeting message appear, we must call (invoke/wake up) the BirthdayGreeting() function. The process of invoking the function is what triggers the execution of the code inside it. This calling or invoking step is essential to see the expected output.
How to Call a function in C++?
We use the function name followed by parentheses to call the function.
#include <iostream>
using namespace std;
//function definition
void BirthdayGreeting() {
for (int i = 0; i < 5; i++) {
cout<<"Happy Birthday"<<endl;
}
}
int main() {
//Function Call
BirthdayGreeting();
return 0;
}
Output
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Now, Let's see how the code works:
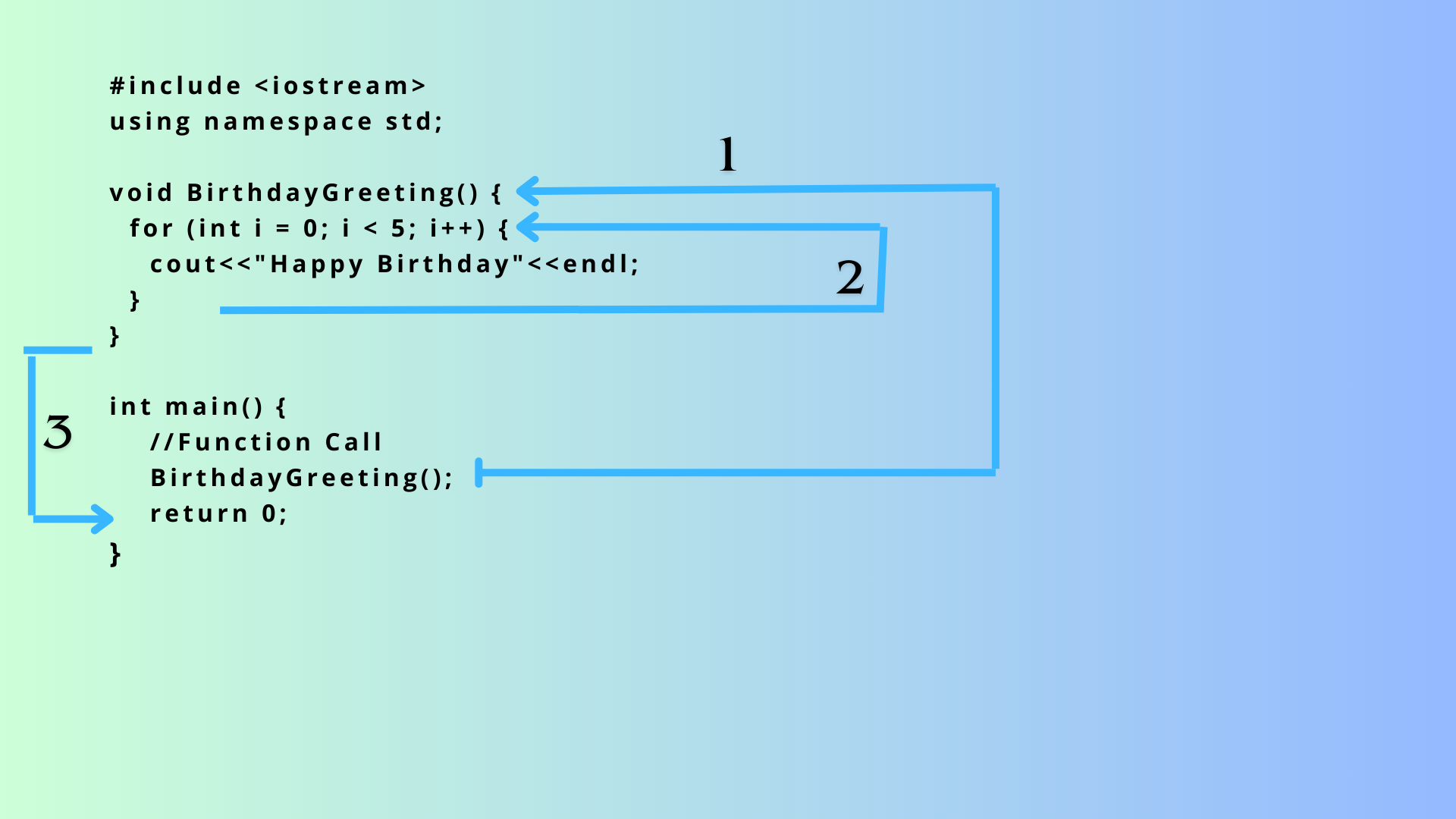
In C++, the program always starts from the main function. Then, it executes line by line. As the function is encountered, the program pauses the main function and jumps to the function, and starts executing the function line by line by line. When the return statement of the function is encountered, the program's control jumps back to the main function and the main function again starts executing line by line till return 0 is not encountered.
In the above diagram, 1, 2, and 3 depicts the following:
- When a function is called, the control of the program jumps to the function definition.
- Then, the statements inside the function are executed.
- After all the statements are executed, the program's control jumps back to the function call, and the next statement is executed inside the main.
Calling a function multiple times
After creating a function, we can use it multiple times there is no limit on that.
#include <iostream>
using namespace std;
//function definition
void BirthdayGreeting() {
for (int i = 0; i < 5; i++) {
cout << "Happy Birthday" << endl;
}
}
int main() {
//Function Call
BirthdayGreeting();
cout << endl;
//Call the function again
BirthdayGreeting();
return 0;
}
Output
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
Happy Birthday
In the above example, we have called the function BirthdayGreeting() two times.
The program always starts from the main function. Inside it, firstly, the BirthdayGreeting( ) function is encountered. Then, the program's control jumps from the main to the BirthdayGreeting( ) function, and the code inside the function is executed. After the function, BirthdayGreeting( ) ends, the control again jumps back to the main, and the next line(which is cout<<endl;) inside the main is executed.
Again, the BirthdayGreeting( ) function is encountered and the program's control jumps from the main to the BirthdayGreeting( ) function and the code inside the function is executed. After the function, BirthdayGreeting( ) ends, the control again jumps back to the main. The next line(which is return 0; ) inside the main is executed and the program ends here.
If we need to do a repetitive task, we can wrap the code inside a function and use the function multiple times.
Quiz
Question 1
Question 2
We are done with the basics of function concepts. I hope now you are confident with these concepts. It is important to understand the fundamentals.
See you in the next article with more energy and excitement ✌️ till then Happy Learning !! 😀