Introduction to C++
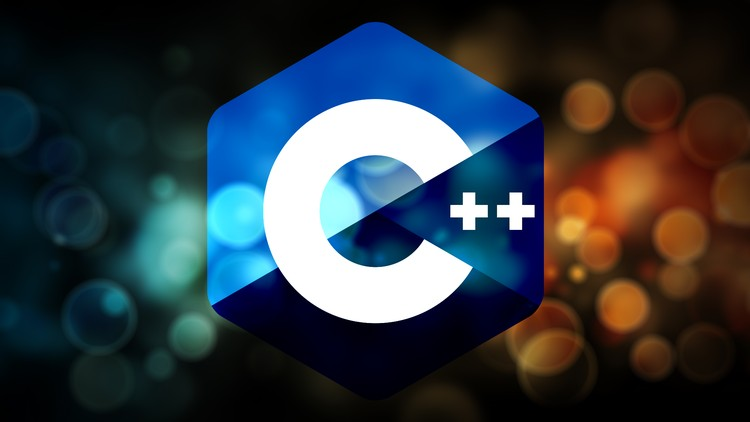
What is a programming language and why do we need it ?
Before we get into C++, let’s talk about what a programming language is and why we need it.I want my computer, which is just a machine, to do something for me — like perform some calculations, run a program, play songs, or maybe even play more songs if I ask it to. If I were asking a human to do this, I could just speak to them in English or Hindi, and they’d understand me. But computers don’t work like that. They can’t understand languages spoken by humans, like English or Hindi. Instead, they only understand something called binary, which is made up of 0s and 1s and it's good also that computers can't understand our language like Hindi and English since our language might contain ambiguity.
That’s where programming languages come in. Think of them as a bridge between us and the computer. They allow us to write instructions in a way that’s much easier for humans to understand. Once we write the code, the computer translates it into 0s and 1s behind the scenes and then runs the task for us. Cool, right?
Programming languages are designed to solve two key problems:
- Ambuiguity in Natural Language
Unlike natural languages such as Hindi or English, where words or sentences can have multiple meanings depending on context, programming languages are precise and unambiguous. They achieve this by enforcing a clear, standardized syntax, ensuring every instruction has a single, well-defined meaning. For example, in natural language, a sentence like "He saw the man with the telescope" could be interpreted in multiple ways. Programming languages, however, eliminate such vagueness by adhering to a strict and concise structure, making them a reliable bridge between humans and machines.
- Clarity in Communication
Programming langugae solve a second key problem by acting as a middle ground between humans and computers. Computers operate using binary(0s and 1s), which is nearly impossible for humans to write or understand. On the other hand, computers cannot interpret natural human languages directly.This gap is where the programming language comes in- they are designed to simplify complex tasks into instructions that are easy for humans to write and understand, while still being translatable into machine-executable code.
Let’s take a simple example:Imagine you’re using Google Maps to find the fastest route to a new restaurant. The app figures this out by analysing real-time traffic data, checking road closures, and calculating distances — all within seconds! This magic happens because programmers wrote instructions (code) in a programming language that helps the app “think” and make decisions. Without programming languages, creating apps like Google Maps would be nearly impossible.
Introduction to C++
C++ is a programming language that’s used for many different purposes, which makes it super versatile. Think of it as a tool that can handle a variety of tasks, like a Swiss Army knife. It’s fast, powerful, and can be used to create anything from video games to operating systems.
C++ builds on an older language called C, which we’ll talk about in more detail in upcoming articles about C++. For now, just know that C++ takes everything great about C and adds something even better: the benefits of Object-Oriented Programming (OOP). This means you can organize your programs into reusable, modular pieces, making it easier to manage and expand your code.
C++ is widely used by some of the biggest companies and industries around the world.Companies like Epic Games use C++ to build highly complex and visually stunning games with engines like Unreal Engine.Web browsers like Google Chrome, Mozilla Firefox, and Safari use C++ for rendering engines and performance-critical tasks.Programs such as Adobe Photoshop, Blender rely on C++ for robust performance in graphic and design applications.
In short, C++ is great for beginners because it’s widely used in building software and game development. Many schools and universities use C++ as an introductory programming language because it combines simplicity for beginners with the depth needed for advanced programming concepts.
The best way to learn programming: Start writing code
The best way to learn programming is by actually writing code! Programming isn’t something you can master just by reading about it—it’s all about practice.When learning a programming language, most people start with a simple program to print "Hello World" on the screen. Why? Because it’s easy to write and gives you immediate feedback when it works. Seeing those two words appear on your screen feels like unlocking a door to a new world!
So, let’s do it together. Open a code editor of your choice
- If you have VS Code or Atom installed, use one of them.
- If you prefer an online editor, try platform like playground.learnyard.com.
Once you’re ready, select C++ as the language and type the following code:
#include<iostream>
using namespace std;
int main(){
cout << "Hello World!";
return 0;
}
Now, hit the Run and Execute button. You should see this output:
Hello World!
At first glance, you might wonder: Why are we writing all this extra stuff just to print one line of text? Let’s break it down by understanding the structure of the program.
Structure of the program
Preprocessor directive (#include)
This is the line at the top that starts with #include. It’s like telling the computer, “Hey, I’m going to borrow some tools from a library to help me do stuff in this program.”For example, when we write #include <iostream>, we’re including the Input/Output Stream library, which lets us use objects like cout (to print things on the screen) and cin (to take input from the user).
Namespace declaration (using namespace std;)
Before we dive into what using namespace std; does, let’s first understand what a namespace is.
Namespaces in C++ are like shelves in a library. Imagine you have two books with the same title but written by different authors. If you put both books on the same shelf without any labels, it would be confusing to find the right one.
To avoid this confusion, you organize the library by creating shelves (or sections) for different genres, authors, or categories. Now, even if two books have the same name, you can easily find the correct one by looking at the shelf they belong to.Similarly, in programming, namespaces are used to organize and group functions, variables, and classes to avoid ambiguity.
Without namespaces, if two libraries or pieces of code define elements with the same name, it would lead to conflicts, as the compiler wouldn't know which one to use.In C++, many built-in objects, like cout (to print things) and cin (to take input), are grouped in a namespace called std, which stands for “standard.”
Why do we use using namespace std;?By default, if you want to use something from the std namespace, you need to include the namespace every time, like this:
std::cout << "Hello, World!";
But writing std:: repeatedly can get annoying. To make things easier, we can declare:
using namespace std;
This tells the program:
“I’ll be using the standard namespace a lot, so let’s skip the std:: prefix.”
Now, you can simply write:
cout << "Hello, World!";
Main function (int main() { ... })
This is the heart of every C++ program. The computer always starts running your code from the main() function. Think of it as the front door of your program—it’s where everything begins.The int before main() means that the function will return an integer (a number) when it’s done. Usually, it returns 0, which means the program ran successfully.
Curly braces ({ })
These are used to group your code together. Everything inside the curly braces { } is the main function’s block of code. It’s like the walls of a house—everything that happens in your program is contained inside these braces.
Return statement (return 0;)
At the end of the main() function, we usually write return 0;. This tells the computer:“I’m done now, and everything went fine.”It’s the polite way to signal that the program finished successfully.Now that you’ve written your first “Hello World!” program, try experimenting with it.
Change the message:
cout << "Welcome to LearnYard for Learning C++!";
Run it again and see how the output changes.
Add multiple lines:
cout << "Hello World!";
cout << "Programming is fun!";
You must be wondering—despite writing the code in two different lines, the output appears on the same line:
Hello World!Programming is fun!
Why does this happen? Well, the cout
statement doesn't automatically move to the next line after printing. To fix this and make each message appear on a new line, we have two solutions
Solution-1: Using the \n
character
You can explicitly tell the computer to move to a new line by adding the \n
character at the end of each string this \n
is like the special character over here this is not going to be printed when you are going to run this code.
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!\n";
cout << "Programming is fun!\n";
return 0;
}
Output:
Hello World!
Programming is fun!
Solution-2: Using endl
statement
You can use the endl
manipulator, it's primary function is to insert a newline character (\n
), which moves the cursor to the next line on the screen. This is similar to using the above method only
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl;
cout << "Programming is fun!" << endl;
return 0;
}
Output:
Hello World!
Programming is fun!
Cascading Effect
Here's something even cooler—you can chain multiple cout
statements together using cascading, making your code more concise:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl << "Programming is fun!" << endl;
return 0;
}
Output:
Hello World!
Programming is fun!
This is called the cascading effect, where you use the <<
operator multiple times in a single statement to combine outputs and manipulators like endl
.For now, just go through the entire structure of the program. Even if you don’t fully understand certain terms or concepts, don’t worry—just keep going. You’ll grasp them as you progress through the course.
Note: In every C++ programming statement it must end with the semicolon(;). It's like adding a period at the end of a sentence in English-it tells the computer that the instruction is complete.If you forget the semicolon, the program won't run and you'll get an error.
Why to prefer C++ over other programming languages?
Speed and Performance
Let’s say you’re building something that needs to run super fast, like a video game or a system that reacts in real time. C++ is perfect for this because it’s a compiled language, meaning the computer translates the code into machine language (0s and 1s) before running it. This makes programs written in C++ much faster compared to languages that are interpreted (translated on the fly), like Python.
Strong Control over Hardware
If you ever need to communicate directly with the hardware, like writing drivers or controlling a robot, C++ is the go-to choice. It gives you low-level tools like pointers and direct memory allocation, which means you can manage the computer’s memory and resources exactly how you want.Think of it as driving a manual car instead of an automatic—you’re in total control of what happens under the hood.
Versatility
C++ is like a multitool—it works for both system-level tasks (like building operating systems) and application-level tasks (like making video games or software for users). This makes it great for developers who want one language that can handle both ends of the spectrum.
Portability
Imagine you’re creating a program, and you want it to run on Windows, macOS, and Linux. C++ makes this easy because its code can be compiled to run on different platforms with only small changes. This “write once, run anywhere” flexibility makes it a favorite for cross-platform development.It’s like packing a universal adapter for your travel—your program can work wherever it goes!
Conclusion
And that’s a wrap for this article! 🎉 We’ve gone through the basics to help you understand programming and take your first steps with C++. From what makes C++ awesome to breaking down how its structure works, you’re now ready to start your coding journey. 🚀.
In the next articles, we’ll explore cool topics like arrays, pointers, and memory allocation—these are like the secret ingredients that make C++ so powerful and versatile.I hope this article gave you a solid boost to dive into coding with confidence.
Stay curious, keep experimenting, and let’s unlock the full potential of C++ together. 💻✨.